There are multiple test files for this project. My program must handle not only the expected file but also files with missing records, missing data, bad data, no records, and no file. Please help me with my Python code. def read_data(filename): try: with open(filename, "r") as file: return file.read() except FileNotFoundError: print("Error: Please provide an input file ") return "" except Exception as ex: print("Error:",ex) return "" def extract_data(tags, strings): data = [] start_tag = f"<{tags}>" end_tag = f"" while start_tag in strings: try: start_index = strings.find(start_tag) + len(start_tag) end_index = strings.find(end_tag) if end_index == -1: break value = strings[start_index:end_index] if value: data.append(value) strings = strings[end_index + len(end_tag):] except Exception as exception: print(exception) return data def get_names(string): names = extract_data("name", string) return names def get_descriptions(string): descriptions = extract_data("description", string) return descriptions def get_calories(string): calories = extract_data("calories", string) return calories def get_prices(string): prices = extract_data("price", string) return prices def display_menu(names, descriptions, calories, prices): print("Names -", "Descriptions -", "Calories -", "Prices:") for i in range(len(names)): print(f"{names[i]} - {descriptions[i]} - {calories[i]} - {prices[i]}") def display_stats(calories, prices): total_items = len(calories) average_calories = sum(map(int, calories)) / total_items correct_price = [] for price in prices: try: if price: correct_price.append(float(price[1:])) else: correct_price.append(0.0) except ValueError: print("Error: Missing or bad data") return average_price = sum(correct_price) / total_items print("\nTotal items -", "Average calories -", "Average price:") print(f"{total_items} - {average_calories:.1f} - ${average_price:.2f}") def main(): filename = "simple.xml" string = read_data(filename) if string: names = get_names(string) descriptions = get_descriptions(string) calories = get_calories(string) prices = get_prices(string) if names and prices and descriptions and calories: display_menu(names, descriptions, calories, prices) display_stats(calories, prices) main()
There are multiple test files for this project. My program must handle not only the expected file but also files with missing records, missing data, bad data, no records, and no file.
Please help me with my Python code.
def read_data(filename):
try:
with open(filename, "r") as file:
return file.read()
except FileNotFoundError:
print("Error: Please provide an input file ")
return ""
except Exception as ex:
print("Error:",ex)
return ""
def extract_data(tags, strings):
data = []
start_tag = f"<{tags}>"
end_tag = f"</{tags}>"
while start_tag in strings:
try:
start_index = strings.find(start_tag) + len(start_tag)
end_index = strings.find(end_tag)
if end_index == -1:
break
value = strings[start_index:end_index]
if value:
data.append(value)
strings = strings[end_index + len(end_tag):]
except Exception as exception:
print(exception)
return data
def get_names(string):
names = extract_data("name", string)
return names
def get_descriptions(string):
descriptions = extract_data("description", string)
return descriptions
def get_calories(string):
calories = extract_data("calories", string)
return calories
def get_prices(string):
prices = extract_data("price", string)
return prices
def display_menu(names, descriptions, calories, prices):
print("Names -", "Descriptions -", "Calories -", "Prices:")
for i in range(len(names)):
print(f"{names[i]} - {descriptions[i]} - {calories[i]} - {prices[i]}")
def display_stats(calories, prices):
total_items = len(calories)
average_calories = sum(map(int, calories)) / total_items
correct_price = []
for price in prices:
try:
if price:
correct_price.append(float(price[1:]))
else:
correct_price.append(0.0)
except ValueError:
print("Error: Missing or bad data")
return
average_price = sum(correct_price) / total_items
print("\nTotal items -", "Average calories -", "Average price:")
print(f"{total_items} - {average_calories:.1f} - ${average_price:.2f}")
def main():
filename = "simple.xml"
string = read_data(filename)
if string:
names = get_names(string)
descriptions = get_descriptions(string)
calories = get_calories(string)
prices = get_prices(string)
if names and prices and descriptions and calories:
display_menu(names, descriptions, calories, prices)
display_stats(calories, prices)
main()

Step by step
Solved in 4 steps

Thank you so much for your help!! It worked and from 4 errors it'd down to 2. It says i'm still missing "Error: Missing or bad data" and "File is empty".
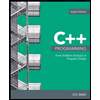
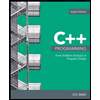