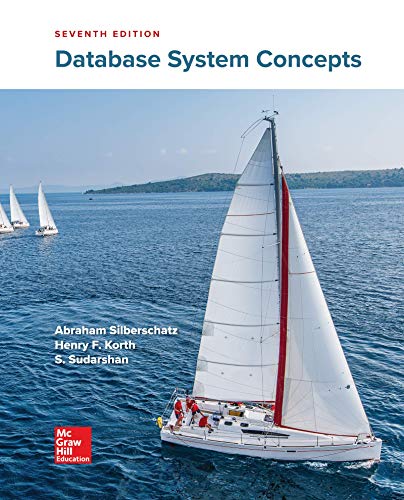
In python using beginners methods (no custom classes etc) and small files -
How do I have a user find and replace bad information in a text file? My instructor says:
- The program will READ in data from a text file named StudyHours.txt. The user corrects any bad data. The program updates the information in StudyHours.txt file. For example if the file contains a letter grade of K which is not a possible letter grade.
StudyHours.txt contains the following data:
- first line full name
- second line number of credits
- third line grade desired for each class
Example format StudyHours.txt file
Aaron RODgers
12
A
Tom brady
9
K
philip Rivers
apple
c
Joe Theismann
15
B
I need to show the user the contents of the file, have them enter in corrections, and then update the file with the correct information with the bad information deleted.
So far I have this:
import os
def main():
found = False
badFile = open('StudyHours.txt', 'r')
print(badFile.read())
search = input('Enter what you want to change: ')
newInfor = input('Enter the new info: ')
descr = badFile.readline()
while descr != '':
credit = str(badFile.readline())
descr = descr.rstrip('\n')
grade = str(badFile.readline())
credit = credit.rstrip('\n')
if descr == search:
tempFile.write(descr +'\n')
tempFile.write(str(newInfor) + '\n')
found = True
else:
tempFile.write(name + '\n')
tempFile.write(str(credit) + '\n')
descr = badFile.readline()
badFile.close()
tempFile.close()
os.rename('temp.txt', 'StudyHours2.txt')
if found:
print('The file has been updated.')
else:
print('That item was not found in the file.')
main()

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- write in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forwardTask. Your task is to develop a python program that reads input from text file and finds if the alphanumeric sequence in each line of the provided input file is valid for Omani car license plate. The rules for valid sequences for car plates in Oman are as follows: Each sequence is composed of 1 to 5 digits followed by one or two letters. Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences. The following list are the only valid letter combinations: ['A','AA','AB','AD','AR','AM','AW','AY', 'B','BA','BB','BD','BR','BM','BW','BY', 'D',DA','DD','DR','DW','DY', "R',RA','RR','RM','RW','RY', 'S','SS', 'M', 'MA','MB','MM','MW','MY', W',WA','WB','ww, Y,YA','YB','YD','YR','YW','YY'] Program Input/Output. Your program should read input from a file named plates.txt and write lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid sequence should be written to a file named invalid.txt. Each line from the input file must…arrow_forwardWrite a program that reads words from a file (filename given as a string parameter) and prints the occurance of each word(case insensitive). And print the words in alphabetical order. For example, if the file contains text Love is free free is love then the function should print free:2 is:2 love:2 def count_word(filename): # YOUR CODE HERE raise NotImplementedError()arrow_forward
- In Java: When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by subtracting the smallest value from all the values. The input begins with an integer indicating the number of integers that follow. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 30 50 10 70 65 the output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program must define and call a method:public static int getMinimumInt(int[] listInts, int listSize) import java.util.Scanner; public class LabProgram {/* Define your method here */ public static void main(String[] args) {/* Type your code here. */}}arrow_forwardWrite a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Hints: Use the find() function to find the index of a comma in each row of…arrow_forwardIn Python: So this is part of a large project I am working on but I'm having a hard time finding out how to read from one text file and then correct that information to another or same txt file as well as to do it alphabetically. Any help is appreciated. The code I've added was from a previous project that was similar as to what this one wants. I can't use custom classes and I believe I'm supposed to be opening the bad information file, writing it and corrections to a temp file and then creating a new file with the right information. I'm in a beginner class and we haven't covered anything past lists. Problem #1: How much should I study outside of class? Issue: Your fellow students liked the 2nd version of study hour’s application and want to expand it again by adding the features listed below. Minimum Study Hours per Week per Class Grade 15 A 12…arrow_forward
- In Java Suppose you are given a text file that contains the names of people. Every name inthe file consists of a first name and a last name. Unfortunately, the programmer thatcreated the file of names had a strange sense of humor and did not guarantee thateach name was on a single line of the file. Write a program that reads this file ofnames and writes them to the console, one name per line.For example, if the input file (Names.txt) contains:Bob Jones FredCharles EdMarstonJeffWilliamsThe output should be:Bob JonesFred CharlesEd MarstonJeff Williams Sample Output:Enter the name of the input file:Names.txtBob JonesFred CharlesEd MarstonJeff WilliamsFile processing completed.arrow_forwardCreate a file with given numbers. Read them from file with a python program andcalculate mean, median, variance and standard deviation for this data.Mean, Variance, Standard Deviation,Here μ = = Mean900 932 298 918 645 505 922 324 979 360 775 53 12 986 764 400 81 923 233 450897 166 787 148 376 385 49 62 149 618 568 270 52 295 278 705 942 341 365 74 538604 958 816 634 566 216 919 475 988 732 835 200 741 587 910 183 204 684 349373 29 503 63 217 213 656 416 350 386 257 827 820 807 369 634 185 690 255 312380 449 564 615 966 342 153 425 830 365 347 487 550 788 959 79 129 666 115 27arrow_forwardPython Create a program that reads the file (create a paragraph in a file). It should analyze each character of the file. For output, it should produce a well-formatted table indicating: The total number of printable characters The total number of capital letters The total number of lowercase letters The total number of numbers The total number of sentences (which end in a period) The total number of paragraphs (which end in a newline) Since the lab is an exercise in strings, you must use at least TWO different string methods to required information. And each stastic will need its own function.arrow_forward
- Write a python program that prompts the user for their favorite basketball team. It should be able to read the list of teams provided below in a file called favorite_teams.txt and check if their team is in that file. Teams in the file:JazzBullsMavericksSpursIf the team is in the file let the user know that their team is in the list of favorites. If the team is not in the file, add the team to the end of the file. Also, let the user know that their team will be added to the file.Sample Run in File:What is your favorite NBA team? Jazz [Enter]Your team Jazz is in the listFile before and after run: JazzBullsMavericksSpurs Sample Run not in File:What is your favorite NBA team? Pelicans [Enter]Your team Pelicans is not in the list. It will be added.File before run: JazzBullsMavericksSpursFile after run:JazzBullsMavericksSpursPelicansarrow_forwardWrite a program in C++ that prints a sorted phone list from a database of names and phone numbers. The data is contained in two files named "phoneNames.txt" and "phoneNums.txt". The files may contain up to 2000 names and phone numbers. The files have one name or one phone number per line. To save paper, only print the first 50 lines of the output. Note: The first phone number in the phone number file corresponds to the first name in the name file. The second phone number in the phone number file corresponds to the second name in the name file. etc. You will find the test files in the Asn Five.ziparrow_forwardFor this assignment, you will write a Python program that uses a file named scores.txt to store sets of bowling scores for different dates. scores.txt should store the data so that each line has a month, day, and the scores the user earned on that date. As an example, scores.txt might look something like this: January 15 200 300 126 200 250April 20 125 100 May 17 300 100 215 The very first time your program starts, scores.txt should not exist (i.e. create it with Python code the first time the program runs). In your main function, continuously give the user the following five options: Quit the program. View all Scores. If the user selects this option, call a function named view_scores. This function should print the scores in a nicely formatted manner. For example: "On January 15, you scored 200, 300, and 126", etc.. Add a Score. If the user selects this option, call a function named add_score. This function should ask the user for a month, day, and as many scores as they want to…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
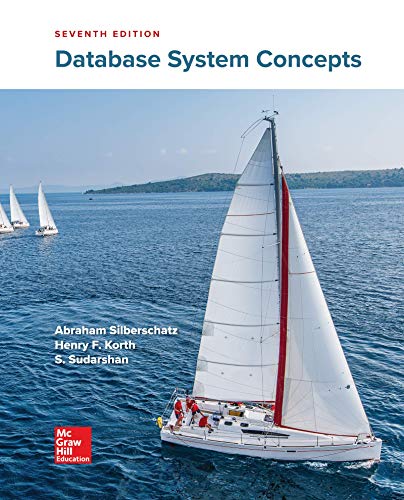
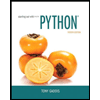
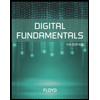
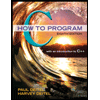
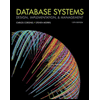
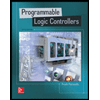