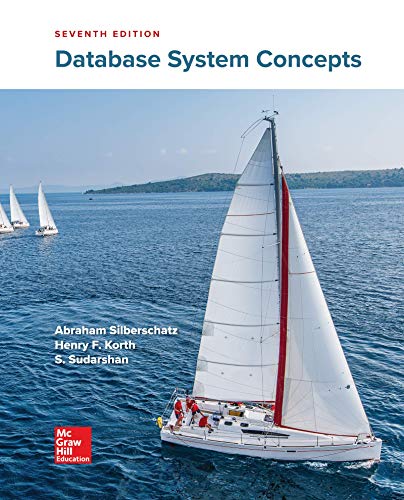
Concept explainers
JAVA
PLEASE MODIFY THIS PROGRAM and take out the following from the program please: Boynames.txt is missing.\n Girlnames.txt is missing.\n Both data files are missing.\n THE TEST CASE DOES NOT ASK FOR IT. PLEASE REMOVE IT. I HAVE PROVIDED THE TEST CASE THAT FAILED AND THE INPUTS AS A SCREENSHOT.
import java.io.*;
import java.util.*;
public class NameSearcher {
private static List<String> loadFileToList(String filename) throws FileNotFoundException {
List<String> namesList = new ArrayList<>();
File file = new File(filename);
if (!file.exists()) {
throw new FileNotFoundException(filename);
}
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine().trim();
String[] names = line.split("\\s+");
for (String name : names) {
namesList.add(name.toLowerCase());
}
}
}
return namesList;
}
private static Integer searchNameInList(String name, List<String> namesList) {
int index = namesList.indexOf(name.toLowerCase());
return index == -1 ? null : index + 1;
}
public static void main(String[] args) {
List<String> boyNames = new ArrayList<>(); // Initialize with an empty list
List<String> girlNames = new ArrayList<>(); // Initialize with an empty list
boolean boyFileExists = false;
boolean girlFileExists = false;
try {
boyNames = loadFileToList("Boynames.txt");
boyFileExists = true;
} catch (FileNotFoundException e) {
System.out.println("Boynames.txt is missing.");
}
try {
girlNames = loadFileToList("Girlnames.txt");
girlFileExists = true;
} catch (FileNotFoundException e) {
System.out.println("Girlnames.txt is missing.");
}
if (!boyFileExists && !girlFileExists) {
System.out.println("Both data files are missing.");
return;
}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Enter a name to search or type QUIT to exit: ");
String input = scanner.nextLine().trim();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
String capitalizedInput = input.substring(0, 1).toUpperCase() + input.substring(1).toLowerCase();
Integer boyIndex = searchNameInList(input, boyNames);
Integer girlIndex = searchNameInList(input, girlNames);
if (boyIndex == null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was not found in either list.");
} else if (boyIndex != null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular boy names list (line " + boyIndex + ").");
} else if (boyIndex == null && girlIndex != null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular girl names list (line " + girlIndex + ").");
} else {
System.out.println("The name '" + capitalizedInput + "' was found in both lists: boy names (line " + boyIndex + ") and girl names (line " + girlIndex + ").");
}
}
scanner.close();
}
}
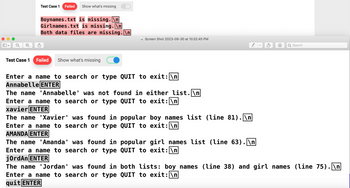
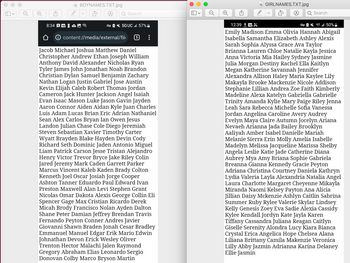

Step by stepSolved in 4 steps with 4 images

- Need help writing this java code, it has three objectives: to process strings to compare, search, sort, and verify location of specific pattern to output result via interface Description Write a Java program to read a text file (command line input for file name), process the text file and perform the following. Print the total number of words in the file. (When using java_test.txt, I calculate the number of words, including number, as 254.) Print the total number of different words (case sensitive, meaning “We” and “we” are two different words) in the file. (When using java_test.txt, I calculate the number of different words, including number, as 168.) Print all words in ascending order (based on the ASCII code) without duplication. Write a pattern match method to find the location(s) of a specific word. This method should return all line number(s) and location(s) of the word(s) found in the file. Print all line(s) with line number(s) where the word is found by invoking the method…arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** The text files are located in Hypergrade. Also for test case 1 first display Please enter a string to convert to Morse code:\n then you press Enter it should print out \n. Then for test case 2 it should display Please enter a string to convert to Morse code:\n then you type abc it should print out .- -... -.-. \n For test case 3 first display Please enter a string to convert to Morse code:\n then you type This is a sample string 1234.ENTER it should print put - .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n. This program down below does not pass the test cases as shown in the screenshot I have provided the correct test case as a screenshot too. Please modify it or create a new program so it paases the test cases. Thank you! For test case 1 it wants only Please enter a string to convert to Morse…arrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forward
- In this assignment, you will create a Java program to search recursively for files in a directory.• The program must take two command line parameters. First parameter is the folder to search for. Thesecond parameter is the filename to look for, which may only be a partial name.• If incorrect number of parameters are given, your program should print an error message and show thecorrect format.• Your program must search recursively in the given directory for the files whose name contains the givenfilename. Once a match is found, your program should print the full path of the file, followed by a newline.• You can implement everything in the main class. You need define a recursive method such as:public static search(File sourceFolder, String filename)For each subfolder in sourceFolder, you recursively call the method to search.• A sample run of the program may look like this://The following command line example searches any file with “Assignment” in its name%java FuAssign7.FuAssignment7…arrow_forwardThis is for Advanced Java Programming Write a test program that reads words from a text file and displays all non-duplicate words in ascending order and then in descending order. - The file is to be referenced in the program, not needing to be used as a command line reference. - The word file is to be titled collection_of_words.txt and included in your submission. Write test code that ensures the code functions correctly.arrow_forwardJAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage PLEASE FIX AND MODIFY THIS JAVA SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASES. RIGHT NOW IT SAYS 0 OUT 3 PASSED. THE PICTURES THAT I PROVIDED PROOF THAT WHEN I UPLOAD IT TO HYPERGRADES IT FAILS TEST CASSES. THANK YOU. import java.io.*;import java.util.Scanner;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Please enter the file name: "); while (true) { String fileName = scanner.nextLine(); File file = new File(fileName); if (file.exists() &&…arrow_forward
- JAVA PPROGRAM ASAP Please Modify this program ASAP BECAUSE IT IS HOMEWORK ASSIGNMENT so it passes all the test cases. It does not pass the test cases when I upload it to Hypergrade. Because RIGHT NOW IT PASSES 0 OUT OF 1 TEST CASES. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;public class Main { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); String fileName; System.out.println("Please enter the file name or type QUIT to exit:"); fileName = keyboard.nextLine().trim(); while (!fileName.equalsIgnoreCase("QUIT")) { File file = new File(fileName); if (file.exists()) { int wordCount = countWords(file); System.out.println("Total number of words: " + wordCount + "\n);…arrow_forwardPython Please. and the data file is: 2121211143534722arrow_forwardI am trying to figure out why the sum at the end of my Java code is not working correctly. It keeps showing as zero and I do not know what the issue is. Here is the program requirements Write a program in a single file that: Main: Creates 10 random doubles, all between 1 and 11, Calls a method that writes 10 random doubles to a text file, one number per line. Calls a method that reads the text file and displays all the doubles and their sum accurate to two decimal places. SAMPLE OUTPUT 10.62691196041722.7377903389094555.4279257388651281.37420580654725091.18587002624988364.1803912764852284.9109699989306755.7108582343439587.7908570073730523.1806714736219543 The total is 47.13 Here is my code: import java.util.*;import java.io.*; public class AssignmentTwo { public static void main(String[] args) {//main method double randNum [] = new double [10]; for(int i = 0; i< randNum.length; i++) { randNum[i] = (double)(Math.random()* (10) + 1);…arrow_forward
- JAVA PPROGRAM ASAP Please Modify this program ASAP BECAUSE IT IS HOMEWORK ASSIGNMENT so it passes all the test cases. It does not pass the test cases when I upload it to Hypergrade. Because RIGHT NOW IT PASSES 1 OUT OF 7 TEST CASES. I have provided the failed the test cases as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;public class Main { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); String fileName; while (true) { System.out.println("Please enter the file name or type QUIT to exit:"); fileName = keyboard.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; // Exit the loop when "QUIT" is entered } File file = new File(fileName); if (file.exists()) { int wordCount = countWords(file);…arrow_forwardJAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage PLEASE FIX AND MODIFY THIS JAVA SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASES. RIGHT NOW IT SAYS 0 OUT 3 PASSED. THE PICTURES THAT I PROVIDED PROOF THAT WHEN I UPLOAD IT TO HYPERGRADES IT FAILS TEST CASSES. THANK YOU. import java.io.*;import java.util.Scanner;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Please enter the file name: "); while (true) { String fileName = scanner.nextLine(); File file = new File(fileName); if (file.exists() &&…arrow_forwardJava Proram ASAP There is an extra /n in the program in test case 1-3 and after Please re-enter the file name or type QUIT to exit:\n quitENTER in test case 4 there needs to be nothing as shown in the screenshot. The text files are located in Hypergrade. Please modify this code below so it passes the test cases. Also I have the correct test case as a screenshot. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { String filePath = new File("").getAbsolutePath(); filePath = filePath.concat("/"); filePath = filePath.concat(input); File file = new File(filePath); if…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
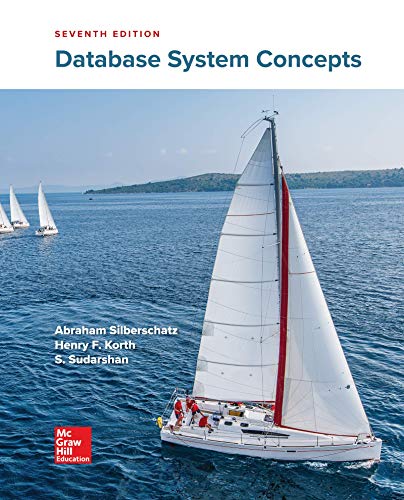
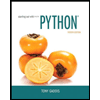
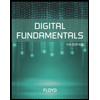
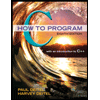
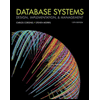
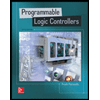