There is no arrayTree or testTreeADT description!!!!! 1. Problem Description You are to develop a program that plays the perfect game of TicTacToe. It should never lose. Your project can be done in three steps of increasing complexity. Develop the Tree ADT as an array-based concrete implementation. For the program, there is no code to start with; you are to develop everything. However, the UML design and test drivers for the Tree ADT have been supplied so you can test your implementation. You can review the sample output below to get an idea of the interaction. Create the Array-based Tree ADT. Run it with TestTreeADT.java to insure it works.
There is no arrayTree or testTreeADT description!!!!! 1. Problem Description You are to develop a program that plays the perfect game of TicTacToe. It should never lose. Your project can be done in three steps of increasing complexity. Develop the Tree ADT as an array-based concrete implementation. For the program, there is no code to start with; you are to develop everything. However, the UML design and test drivers for the Tree ADT have been supplied so you can test your implementation. You can review the sample output below to get an idea of the interaction. Create the Array-based Tree ADT. Run it with TestTreeADT.java to insure it works.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
There is no arrayTree or testTreeADT description!!!!!
1. Problem Description
You are to develop a
project can be done in three steps of increasing complexity.
Develop the Tree ADT as an array-based concrete
implementation.
For the program, there is no code to start with; you are to develop everything. However, the UML
design and test drivers for the Tree ADT have been supplied so you can test your implementation. You can
review the sample output below to get an idea of the interaction.
Create the Array-based Tree ADT. Run it with TestTreeADT.java to insure it works.
![2 public class TestTreeADT {
public static void main(String[] args) {
ArrayTree<Integer> al = new ArrayTree<Integer>(2, 25);
ArrayTree<Integer> a2 = new ArrayTree<Integer>(5, 55);
int val - 1;
30
4
80
System.out.println("*** Test ArrayTree ADT ***\n");
9.
testcase("al.isEmpty ()", al.isEmpty() ? 1: 0, 1);
10
11
int pos = al.addRoot(val++);
for (int i = 0; i < 10; i++) {
a1.addChild(pos, 0, val++);
a1.addChild(pos, 1, val++);
12
13
14
15
16
postt;
}
17
18
testcase("al.root()", a1.root(), 0);
testcase ("al.parent ()", al.parent(7), 3);
testcase ("al.child()", al.child(10, 1), 22);
testcase("al.size()", al.size(), 21);
testcase("al.isEmpty ()", al.isEmpty() ? 1 : 0, 0);
testcase ("al.get()", al.get(3), 4);
testcase("al.getChild()", a1.getChild(6, 1), 15);
System.out.println("Small Tree:
19
20
21
22
23
24
25
+ al +"\n");
26
27
28
testcase("a2.isEmpty()", a2.isEmpty() ? 1: 0, 1);
29
30
a2.addRoot (val++);
pos =
for (int i = 0; i < 10; it++) {
for (int j = 0; j < 5; j++) {
a2. addChild(pos, j, val++);
}
31
32
33
34
35
36
pos++;
37
38
testcase ("a2.root()", a2.root(), ®);
testcase("a2.parent ()", a2.parent(27), 5);
testcase("a2.child()", a2.child(14, 4), 75);
testcase("a2.size()", a2.size(), 51);
testcase ("a2.isEmpty()", a2.isEmpty () ? 1: 0, 0);
testcase("a2.get()", a2.get(18), 40);
testcase ("a2.getChild()", a2.getChild(9, 0), 68);
System.out.println("Large Tree:
}
39
40
41
42
43
44
45
46
47
48
49e
+ a2);
private static void testcase (String description, int actual, int expected) {
== expected) {
if (actual
System.out.println("Pass:
} else {
System.out.println("Fail:
50
+ description);
51
52
+ description +
, еxpected
+ expected +
+ actual);
53
» got
54
55
56 }](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcb678de0-37ec-48b5-a333-b25767368096%2Fe47b99b4-3682-420d-bb92-e774ada26f21%2Fiqb8w24_processed.jpeg&w=3840&q=75)
Transcribed Image Text:2 public class TestTreeADT {
public static void main(String[] args) {
ArrayTree<Integer> al = new ArrayTree<Integer>(2, 25);
ArrayTree<Integer> a2 = new ArrayTree<Integer>(5, 55);
int val - 1;
30
4
80
System.out.println("*** Test ArrayTree ADT ***\n");
9.
testcase("al.isEmpty ()", al.isEmpty() ? 1: 0, 1);
10
11
int pos = al.addRoot(val++);
for (int i = 0; i < 10; i++) {
a1.addChild(pos, 0, val++);
a1.addChild(pos, 1, val++);
12
13
14
15
16
postt;
}
17
18
testcase("al.root()", a1.root(), 0);
testcase ("al.parent ()", al.parent(7), 3);
testcase ("al.child()", al.child(10, 1), 22);
testcase("al.size()", al.size(), 21);
testcase("al.isEmpty ()", al.isEmpty() ? 1 : 0, 0);
testcase ("al.get()", al.get(3), 4);
testcase("al.getChild()", a1.getChild(6, 1), 15);
System.out.println("Small Tree:
19
20
21
22
23
24
25
+ al +"\n");
26
27
28
testcase("a2.isEmpty()", a2.isEmpty() ? 1: 0, 1);
29
30
a2.addRoot (val++);
pos =
for (int i = 0; i < 10; it++) {
for (int j = 0; j < 5; j++) {
a2. addChild(pos, j, val++);
}
31
32
33
34
35
36
pos++;
37
38
testcase ("a2.root()", a2.root(), ®);
testcase("a2.parent ()", a2.parent(27), 5);
testcase("a2.child()", a2.child(14, 4), 75);
testcase("a2.size()", a2.size(), 51);
testcase ("a2.isEmpty()", a2.isEmpty () ? 1: 0, 0);
testcase("a2.get()", a2.get(18), 40);
testcase ("a2.getChild()", a2.getChild(9, 0), 68);
System.out.println("Large Tree:
}
39
40
41
42
43
44
45
46
47
48
49e
+ a2);
private static void testcase (String description, int actual, int expected) {
== expected) {
if (actual
System.out.println("Pass:
} else {
System.out.println("Fail:
50
+ description);
51
52
+ description +
, еxpected
+ expected +
+ actual);
53
» got
54
55
56 }
![ArrayTree
-array[): E
-count: int
-size: int
-order: int
+ArrayTree(order: int, capacity: int)
+ArrayTree()
+root(): int
+parent(p: int): int
+child(p: parent, c: int): int
+size(): int
+isEmpty(): boolean
2. Additional Instructions
2.1. Basic Level: Array Tree ADT
Develop an ArrayTree class to meet the design shown here. It should not implement +addRoot(e: E): int
the Tree<E> interface we discussed in class.
+get(pos: int): E
The behavior of each method should be clear from our discussion in class. While the
class is generic (element is type E), the positions are all primitive integers. The default +addChild(parent: int, child: int, e: E): int
order is 2 and the default capacity is 1000. toString() should print a summary of +getChild(parent: int, child: int): E
the data elements as shown in the sample output below:
+toString(): String
Running your ArrayTree with TestTreeADT.java should result in the following output. Note: it's ok that you get
one Fail in testing a2. You should understand why that is correct as you implement the TreeADT.
** Test ArrayTree ADT **.
Pass: al.isEmpty()
Pass: al.root()
Pass: al.parent()
Pass: al.child()
Pass: al.size()
Pass: al.isEmpty()
Pass: al.get()
Pass: al.getChild()
Small Tree: [ArrayTree: order=2, count=21, size-25, array=(1 2 3 4 5 67 89 10 11 12 13 14 15 16
17 18 19 20 21 - - -- })
Pass: a2.isEmpty()
Pass: a2.root()
Pass: a2.parent()
Fail: a2.child(), expected 75, got -1
Pass: a2.size()
Pass: a2.isEmpty()
Pass: a2.get()
Pass: a2.getChild()
Large Tree: [ArrayTree: order-5, count-51, size-55, array-(22 23 24 25 26 27 28 29 30 31 32 33 34
35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
68 69 70 71 72 - - -- }]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcb678de0-37ec-48b5-a333-b25767368096%2Fe47b99b4-3682-420d-bb92-e774ada26f21%2F47ek0f_processed.jpeg&w=3840&q=75)
Transcribed Image Text:ArrayTree
-array[): E
-count: int
-size: int
-order: int
+ArrayTree(order: int, capacity: int)
+ArrayTree()
+root(): int
+parent(p: int): int
+child(p: parent, c: int): int
+size(): int
+isEmpty(): boolean
2. Additional Instructions
2.1. Basic Level: Array Tree ADT
Develop an ArrayTree class to meet the design shown here. It should not implement +addRoot(e: E): int
the Tree<E> interface we discussed in class.
+get(pos: int): E
The behavior of each method should be clear from our discussion in class. While the
class is generic (element is type E), the positions are all primitive integers. The default +addChild(parent: int, child: int, e: E): int
order is 2 and the default capacity is 1000. toString() should print a summary of +getChild(parent: int, child: int): E
the data elements as shown in the sample output below:
+toString(): String
Running your ArrayTree with TestTreeADT.java should result in the following output. Note: it's ok that you get
one Fail in testing a2. You should understand why that is correct as you implement the TreeADT.
** Test ArrayTree ADT **.
Pass: al.isEmpty()
Pass: al.root()
Pass: al.parent()
Pass: al.child()
Pass: al.size()
Pass: al.isEmpty()
Pass: al.get()
Pass: al.getChild()
Small Tree: [ArrayTree: order=2, count=21, size-25, array=(1 2 3 4 5 67 89 10 11 12 13 14 15 16
17 18 19 20 21 - - -- })
Pass: a2.isEmpty()
Pass: a2.root()
Pass: a2.parent()
Fail: a2.child(), expected 75, got -1
Pass: a2.size()
Pass: a2.isEmpty()
Pass: a2.get()
Pass: a2.getChild()
Large Tree: [ArrayTree: order-5, count-51, size-55, array-(22 23 24 25 26 27 28 29 30 31 32 33 34
35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
68 69 70 71 72 - - -- }]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
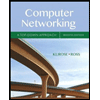
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
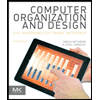
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
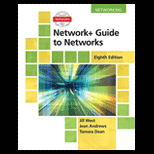
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
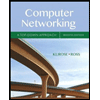
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
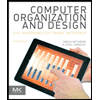
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
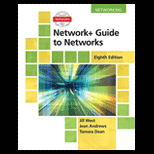
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
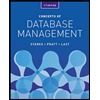
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
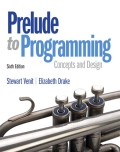
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
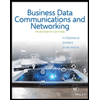
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY