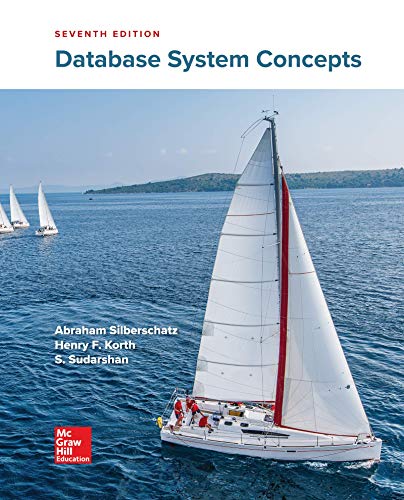
*Please using JAVA only*
Objective
The primary objective of this program is to learn to implement binary search trees and to combine their functionalities with linked lists.
Program Description
In a multiplayer game, players' avatars are placed in a large game scene, and each avatar has its information in the game. Write a program to manage players' information in a multiplayer game using a Binary Search (BS) tree for a multiplayer game. A node in the BS tree represents each player. Each player should have an ID number, avatar name, and stamina level. The players will be arranged in the BS tree based on their ID numbers. If there is only one player in the game scene, it is represented by one node (root) in the tree. Once another player enters the game scene, a new node will be created and inserted in the BS tree based on the player ID number. Players during the gameplay will receive hits that reduce their stamina. If the players lose all their stamina, they vanish from the game, and they should be deleted from the tree. You are required to write the following methods:
-
Write a function to add a new player by adding a new node to the tree.
-
Write a function to search for a specific player by their ID.
-
Write a function that prints all the players' names with their specified IDs and stamina
levels.
-
Write a function that prints the number of players that existed in the game.
-
Write a function that updates the stamina level for a specific player when they receive
hits from another player.
-
Write a function that removes a particular player from the game by removing their
node from the tree.
The program deals with three files. Two input files and one output file. The description of these files is as follow:
o The first input file (intialInformation.txt) contains the important information for the program which includes the number of players, the IDs, and the name for each player.
o The information in this file is arranged as follow:
o The first line contains the number of players.
o The following lines contain the players’ IDs and names.
o The commands for the system are found in the second file called commands.txt. The commands in this file are as follow:
o STARTUP: This command will use the first input file (intialInformation.txt) to generate the BS tree by creating a node for each player in the tree.
o ADD_PLAYER: This command has two values that represent the player ID and the player’s name who entered the game scene. The command will create a node in the tree that represents that player in the game. The default value for the stamina level will be 15.
o DISPLAY_PLAYER_INFO:Thiscommandhasonevaluewhichistheplayer ID. It will output the name and the stamina level for the specified player. If the
4
specified player is not found, it will output the “Not found any player with ID
number <ID >” message.
o DISPLAY_ALL_PLAYERS: This command will output all the players in the
game scene with the players’ IDs, names and stamina levels. (Similar to the
output in the ouptput.txt file).
o NUM_OF_PLAYERS: This command will print the total number of players
in the game scene.
o UPDATE_PLAYER_INFO: This command has one value which is the
player ID. It will decrease the stamina level for the specified player by 5 and it will output “the player <name> received a hit and the stamina level reduced by 5” message. If the stamina level for the specified player reaches zero, then the player will be removed from the game, and it will output the “The stamina level for the player <name> reaches zero and <name> left the game!” message. If the specified player is not found, it will output the “Not found any player with ID number <ID >” message.
o DELETE_PLAYER: This command has one value that represents the player’s ID who will leave the game. The command will search for this player in the tree then remove their node from the BS tree, and it will output the “Theplayer <name> left the game” message. If the specified player is not found it will output the “Not found any player with ID number <ID >” message.
o QUIT: This command will stop the program.
o Theoutputoftheprogramshouldbewrittentothefilenameitoutput.txt,whichcontent
should be similar to the contents of the file provided to you.
Implementation
For this program, you will create the following classes:
-
● PlayerNode.java: This class will be used to create objects of type player and it will store the player information.
-
● GameTree.java: All the methods will be implemented in this class.
-
● MainProgram.java: This is the class that will contain the main.
Sample Input & Output File
We have provided you a sample for two input files and one output file.
Image of the output
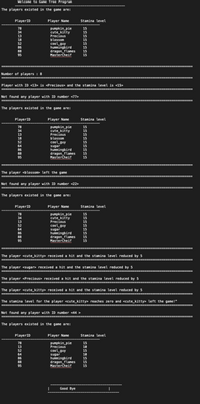

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Program 3: Binary Search Tree Program Objective The primary objective of this program is to learn to implement binary search trees and to combine their functionalities with linked lists. Program Description In a multiplayer game, players' avatars are placed in a large game scene, and each avatar has its information in the game. Write a program to manage players' information in a multiplayer game using a Binary Search (BS) tree for a multiplayer game. A node in the BS tree represents each player. Each player should have an ID number, avatar name, and stamina level. The players will be arranged in the BS tree based on their ID numbers. If there is only one player in the game scene, it is represented by one node (root) in the tree. Once another player enters the game scene, a new node will be created and inserted in the BS tree based on the player ID number. Players during the gameplay will receive hits that reduce their stamina. If the players lose all their stamina, they vanish from the…arrow_forwardComplete the following ():a. A stack is used by the system when a function call is madeb. A stack can become full during program executionarrow_forwardThis data structure provides a variety of benefits over others, such as a linked list or tree.arrow_forward
- Java data strucarrow_forwardC++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the main code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS int main(int argc, char** argv) { SLLStack* stack = new SLLStack(); int test; int length; string str; char top; bool flag = true; cin >> test; switch (test) { case 0: getline(cin, str); length = str.length(); for(int i = 0; i < length; i++){ if(str[i] == '{' || str[i] == '(' || str[i] == '['){ stack->push(str[i]); } else if (str[i] == '}' || str[i] == ')' || str[i] == ']'){ if(!stack->isEmpty()){ top = stack->top(); if(top == '{' && str[i] == '}' || top == '(' && str[i] == ')' ||…arrow_forwardStack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy constructor for the above class. Write Destructor for the above class. Now write a global function show stack which…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
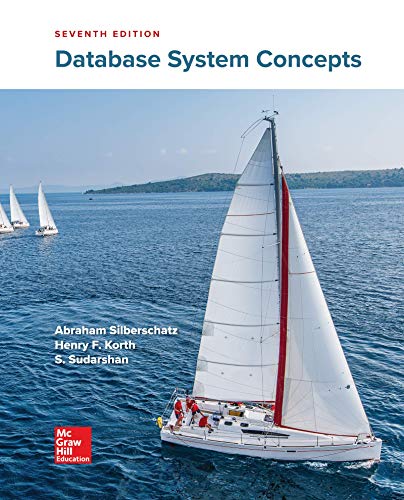
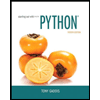
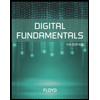
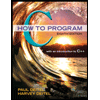
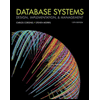
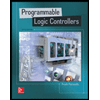