This assignment requires the extension of your graph code to apply it to movement through a “world”. The world will be a weighted, directed graph, with nodes for the start position and target(s), and other nodes containing blocks, diversions, boosts and portals. For example, in a cat world, a dog may block you, toys may take your attention, food may give you more energy and portals may prove that cats are pan-dimensional beings. This structure could also be used to implement Snakes and Ladders, or other games. Your task is to build a representation of the world and explore the possible routes through the world and rank them. Sample input files will be available – with various scenarios in a cat world. Your program should be called gameofcatz.py/java, and have three starting options: * No command line arguments : provides usage information * "-i" : interactive testing environment * "-s" : simulation mode (usage: gameofcatz –s infile savefile) When the program starts in interactive mode, it should show the following main menu: (1) Load input file (2) Node operations (find, insert, delete, update) (3) Edge operations (find, add, remove, update) (4) Parameter tweaks (adjust mapping of codes to penalty/boost features, see sample input file) (5) Display graph (weighted adjacency matrix, option to save) (6) Display world (your choice of representation, does not need to be graphical, should include counts of features, option to save) (7) Generate routes (8) Display routes (ranked, option to save) (9) Save network You can structure the menu/UI differently, just make sure at least those feature options are included. When running in simulation mode, you will give the input files on the command line, then output the ranked paths to a file. Once you have a working program, you will showcase your program, and reflect on its performance. This investigation will be written up as The Report. 3 Submission You should submit a single file, which should be zipped (.zip) or tarred (.tar.gz). Check that you can decompress it on the lab computers. These are also the computers on which your work will be tested, so make sure that your work runs there. The file must be named DSA_Assignment_ where the is replaced by your student id. There should be no spaces in the file name; use underscores as shown. The file must contain the following: * Your code. This means all .java/.py files needed to run your program. Do include code provided to you as part of the assignment if that is required to run your program. * README file including short descriptions of all files and dependencies, and information on how to run the program. * Your unit test harnesses. One of the easiest ways for us to be sure that your code works is to make sure that you’ve tested it properly. Our test harnesses may not work for some of your classes and you may have classes that we’re not specifically asking for, so make it easy for us to test your work. A test harness for class X should be called UnitTestX. * Project Report for your code, as described in Section 2.1. * Java Students: Do not include .class files or anything else that we do not need. We will recompile .java files to ensure that what we’re testing is what we’re reading. We will use javac *.java to compile your files and run the unit tests by their expected names.
This assignment requires the extension of your graph code to apply it to movement through a “world”. The world will be a weighted, directed graph, with nodes for the start position and target(s), and other nodes containing blocks, diversions, boosts and portals. For example, in a cat world, a dog may block you, toys may take your attention, food may give you more energy and portals may prove that cats are pan-dimensional beings. This structure could also be used to implement Snakes and Ladders, or other games. Your task is to build a representation of the world and explore the possible routes through the world and rank them.
Sample input files will be available – with various scenarios in a cat world.
Your program should be called gameofcatz.py/java, and have three starting options:
* No command line arguments : provides usage information
* "-i" : interactive testing environment
* "-s" : simulation mode (usage: gameofcatz –s infile savefile)
When the program starts in interactive mode, it should show the following main menu:
(1) Load input file
(2) Node operations (find, insert, delete, update)
(3) Edge operations (find, add, remove, update)
(4) Parameter tweaks (adjust mapping of codes to penalty/boost features, see sample input file)
(5) Display graph (weighted adjacency matrix, option to save)
(6) Display world (your choice of representation, does not need to be graphical, should include counts of features, option to save)
(7) Generate routes
(8) Display routes (ranked, option to save)
(9) Save network
You can structure the menu/UI differently, just make sure at least those feature options are included.
When running in simulation mode, you will give the input files on the command line, then output the ranked paths to a file.
Once you have a working program, you will showcase your program, and reflect on its performance. This investigation will be written up as The Report.
3 Submission
You should submit a single file, which should be zipped (.zip) or tarred (.tar.gz). Check that you can decompress it on the lab computers. These are also the computers on which your work will be tested, so make sure that your work runs there. The file must be named DSA_Assignment_ where the is replaced by your student id. There should be no spaces in the file name; use underscores as shown.
The file must contain the following:
* Your code. This means all .java/.py files needed to run your program. Do include code provided to you as part of the assignment if that is required to run your program.
* README file including short descriptions of all files and dependencies, and information on how to run the program.
* Your unit test harnesses. One of the easiest ways for us to be sure that your code works is to make sure that you’ve tested it properly. Our test harnesses may not work for some of your classes and you may have classes that we’re not specifically asking for, so make it easy for us to test your work. A test harness for class X should be called UnitTestX.
* Project Report for your code, as described in Section 2.1.
* Java Students: Do not include .class files or anything else that we do not need. We will recompile .java files to ensure that what we’re testing is what we’re reading. We will use javac *.java to compile your files and run the unit tests by their expected names.
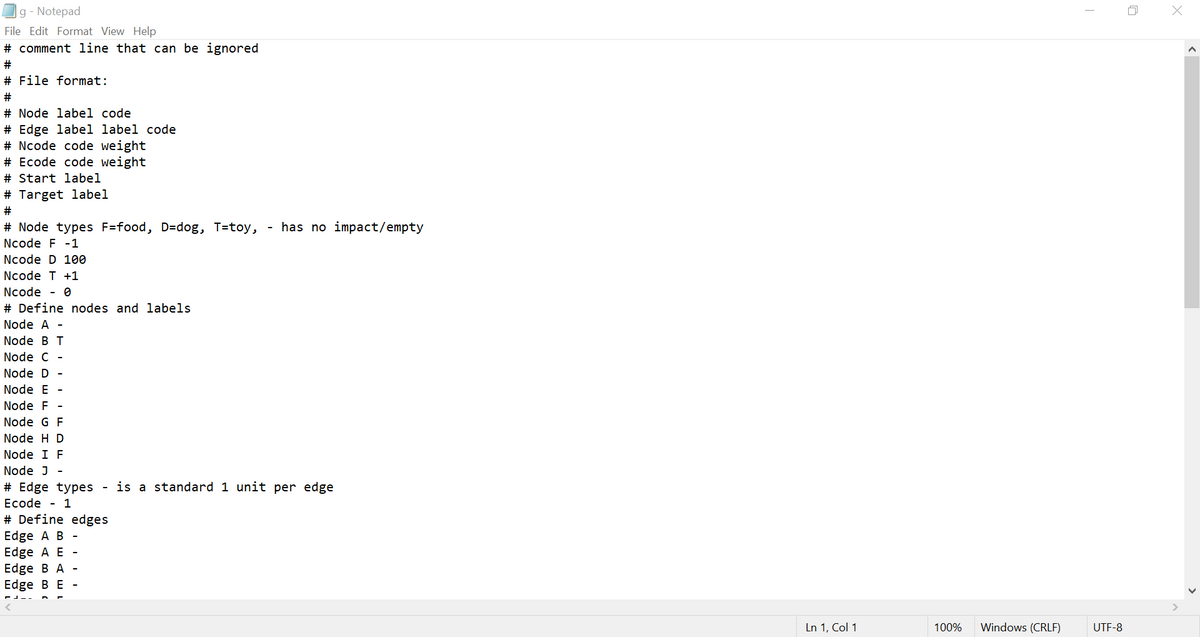
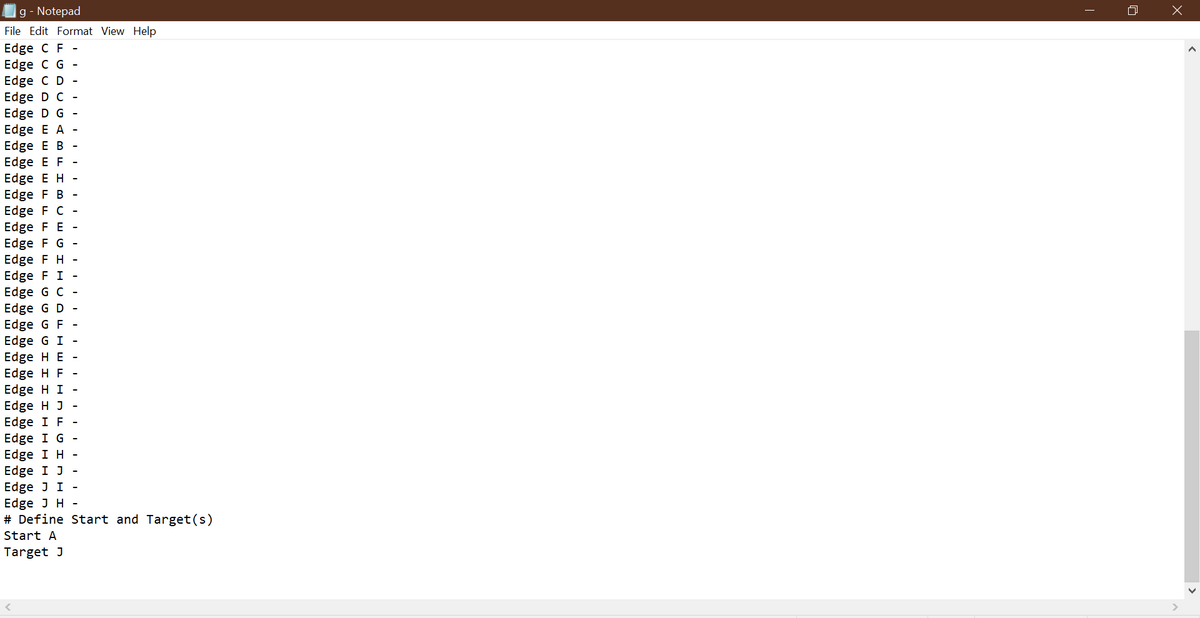

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

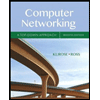
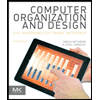
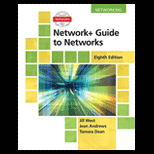
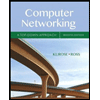
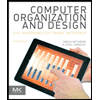
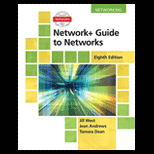
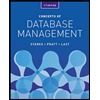
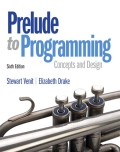
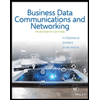