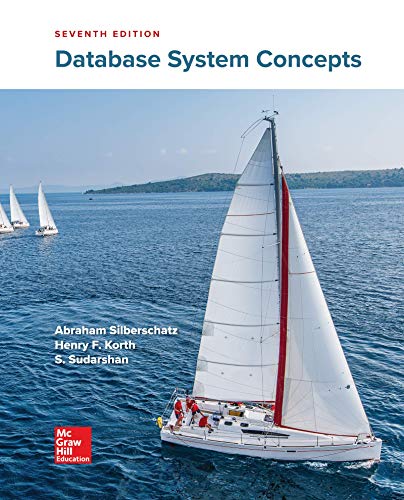
In this code:
#include <iostream>
#include <string>
using namespace std;
// Node struct to store data for each song in the playlist
struct Node
{
string data;
Node * next;
};
// Function to create a new node and return its address
Node * getNewNode(string song)
{
Node * newNode = new Node();
newNode -> data = song;
newNode -> next = NULL;
return newNode;
}
// Function to insert a new node at the head of the linked list
void insertAtHead(Node ** head, string song)
{
Node * newNode = getNewNode(song);
if ( * head == NULL) {
* head = newNode;
return;
}
newNode -> next = * head;
* head = newNode;
}
// Function to insert a new node at the tail of the linked list
void insertAtTail(Node ** head, string song)
{
Node * newNode = getNewNode(song);
if ( * head == NULL) {
* head = newNode;
return;
}
Node * temp = * head;
while (temp -> next != NULL)
{
temp = temp -> next;
}
temp -> next = newNode;
}
// Function to remove a node from the linked list
void removeNode(Node ** head, string song)
{
if ( * head == NULL) {
return;
}
Node * temp = * head;
// If the song to be removed is at the head
if (temp != NULL && temp -> data == song) {
* head = temp -> next;
free(temp);
return;
}
// If the song to be removed is not at the head
while (temp -> next != NULL) {
if (temp -> next -> data == song) {
break;
}
temp = temp -> next;
}
// If the song was not present in the linked list
if (temp -> next == NULL) {
return;
}
// Unlink the node from the linked list
Node * next = temp -> next -> next;
free(temp -> next);
temp -> next = next;
}
// Function to print the contents of the linked list
void printList(Node * head) {
while (head != NULL) {
cout << head -> data << " ";
head = head -> next;
}
cout << endl;
}
// Function to play the songs in the playlist in a loop
void playSongs(Node * head) {
if (head == NULL) {
cout << "Playlist is empty!" << endl;
return;
}
cout << "Playing songs in the playlist:" << endl;
Node * temp = head;
while (temp != NULL) {
cout << temp -> data << endl;
temp = temp -> next;
}
}
int main() {
Node * head = NULL;
insertAtHead( & head, "Song1");
insertAtHead( & head, "Song2");
insertAtTail( & head, "Song3");
insertAtTail( & head, "Song4");
removeNode( & head, "Song2");
printList(head);
playSongs(head);
return 0;
}
how to put a traversing the playlist and allow for common operations on a playlist such as: insert, remove, next, previous, play all songs

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- T/F: All Linked Lists must have head node.arrow_forwardWhat happens when a programmer attempts to access a node's data fields when the node variable refers to None? How do you guard against it? *PYTHONarrow_forward#include <iostream> using namespace std; #define SIZE 5 //creating the queue using array int A[SIZE]; int front = -1; int rear = -1; //function to check if the queue is empty bool isempty() { if(front == -1 && rear == -1) return true; else return false; } //function to enter elements in queue void enqueue ( int value ) { //if queue is full if ((rear + 1)%SIZE == front) cout<<"Queue is full \n"; else { //now the first element is inserted if( front == -1) front = 0; //inserting element at rear end rear = (rear+1)%SIZE; A[rear] = value; } } //function to remove elements from queue void dequeue ( ) { if( isempty() ) cout<<"Queue is empty\n"; else //only one element if( front == rear ) front = rear = -1; else front = ( front + 1)%SIZE; } //function to show the element at front void showfront() { if( isempty()) cout<<"Queue is empty\n"; else cout<<"element at front is:"<<A[front]; } //function to display the queue void…arrow_forward
- Use the following node definition for this problem.struct NodeInt32{int32_t value; NodeInt32* next;} Write a function which searches a non-empty linked list for a target value. Its exact signature should be: NodeInt32* find(NodeInt32* head, int32_t target); The function should return the first node whose value equals target. If the target is not found in the list, then the function should return NULL.arrow_forwardplease complate code in fill in the blanksarrow_forwardProject 2: Singly-Linked List The purpose of this assignment is to assess your ability to: ▪Implement sequential search algorithms for linked list structures ▪Implement sequential abstract data types using linked data ▪Analyze and compare algorithms for efficiency using Big-O notation For this assignment, you will implement a singly-linked node class. Use your singly-linked node to implement a singly-linked list class that maintains its elements in ascending order. The SinglyLinkedList class is defined by the following data: ▪A node pointer to the front and the tail of the list Implement the following methods in your class: ▪A default constructor list<T> myList ▪A copy constructor list<T> myList(aList) ▪Access to first elementmyList.front() ▪Access to last elementmyList.back() ▪Insert value myList.insert(val) ▪Remove value at frontmyList.pop_front() ▪Remove value at tailmyList.pop_back() ▪Determine if emptymyList.empty() ▪Return # of elementsmyList.size() ▪Reverse order of…arrow_forward
- LAB: Playlist (output linked list) Given main(), complete the SongNode class to include the function PrintSongInfo(). Then write the PrintPlaylist() function in main.cpp to print all songs in the playlist. DO NOT print the head node, which does not contain user-input values. Ex: If the input is: Stomp! 380 The Brothers Johnson The Dude 337 Quincy Jones You Don't Own Me 151 Lesley Gore -1 the output is: LIST OF SONGS ------------- Title: Stomp! Length: 380 Artist: The Brothers Johnson Title: The Dude Length: 337 Artist: Quincy Jones Title: You Don't Own Me Length: 151 Artist: Lesley Gorearrow_forwardTepis Linked List By using stt Usc this declaration of the Node clas3: atzuct Node { ant infoJ Node next Node *p, r, *g: Question 1 nitiol Setup Exercise Final Configuration Use o single cssignment Exomple stotement to make the p=p->next->data, variable p refer to the Node with info 2 Use a single ossignment Code: stotement to cssignment statement must refer to both variables p and q. Use o single Ossignment stotement to make the| Code: variable a refer to the / Node with info T. Use o single ossianment | Code: statement to make the variable r refer to the Node with info 2. Use a single ossignment Code: stotement to set the info referred to by p equal to the info of the Node referred to by ryou must access this info through r; do not refer to the of the Node character '3' directly)arrow_forward#ifndef NODES_LLOLL_H#define NODES_LLOLL_H #include <iostream> // for ostream namespace CS3358_SP2023_A5P2{ // child node struct CNode { int data; CNode* link; }; // parent node struct PNode { CNode* data; PNode* link; }; // toolkit functions for LLoLL based on above node definitions void Destroy_cList(CNode*& cListHead); void Destroy_pList(PNode*& pListHead); void ShowAll_DF(PNode* pListHead, std::ostream& outs); void ShowAll_BF(PNode* pListHead, std::ostream& outs);} #endif #include "nodes_LLoLL.h"#include "cnPtrQueue.h"#include <iostream>using namespace std; namespace CS3358_SP2023_A5P2{ // do breadth-first (level) traversal and print data void ShowAll_BF(PNode* pListHead, ostream& outs) { cnPtrQueue queue; CNode* currentNode; queue.push(lloLLPtr->getHead()); while (!queue.empty()) { currentNode = queue.front(); queue.pop(); if…arrow_forward
- program Linked List: modify the following program to make a node containing data values of int, char, and string. #include <iostream> using namespace std; struct node { int data; struct Node *next; }; struct Node* head = nullptr;//or Null or 0; void insert(int new_data) { struct Node* new_node=(struct Node*) new(struct Node); new_mode->data=new_data; new_mode->next=head; head=new_node; } void display() { struct Node* ptr; ptr=head; while(ptr ! = NULL) { cout<<ptr->data<<""; ptr=ptr->next; } } int main() { insert{2}; display{}; return0; }arrow_forwardT LO Assume that the nodeType defined as the following struct nodeType int info; nodetype * link; node Type "List, "first, "current; *"last, "p, "temp; List 11 34 000 - 100 →NULL 47 99 4. 2. d. last first current Write C++ code to delete the last node of the list and also deallocate the memory occupied by this node. After deleting the node make last point to the last node of the list and the link of the last node must be nullptr. You can declare additional pointer variable if it is needed. Click Save and Submit to saue and submit. Click Save All Ansuers to save all answers. Save and Submit English (United States) Focus 168% sp 目 tv 15 D0O 000 F4 F3 F5 F7 F8 F11 %24 5. 9 4.arrow_forwardTree Traversal Coding: How do I code the following in C program? // ====== BEGIN INSERT FUNCTION DEFS TO WALK TREE ========= // define 3 functions - preorder, inorder, postorder to walk tree, printing out data (char) // associated with each node visited: void preorder (node* np) {} void inorder (node* np) {} void postorder (node* np) {} walk.c file with the rest of the code given. #include <stdio.h> #include <stdlib.h> #include <stdbool.h> #include <string.h> #define MAX_STRING 200 // ========================== NODE/TREE DEFINITIONS ========================== // define node structure typedef struct nd { int data; struct nd* left; struct nd* right; } node; // "new" function to create a node, set data value to d and children to NULL node* newNode(int d) { node* np; np = (node*)malloc(sizeof(node)); if (np != NULL) { np->data = d; np->left = NULL; np->right = NULL; } return(np); } // declare root of our binary tree…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
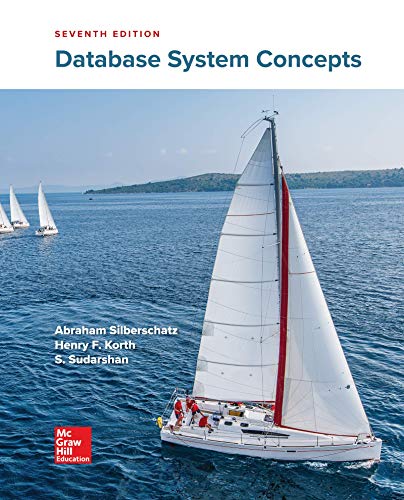
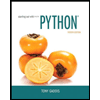
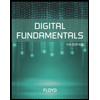
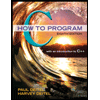
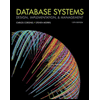
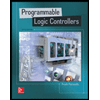