Given main(), complete the SongNode class to include the function PrintSongInfo(). Then write the PrintPlaylist() function in main.cpp to print all songs in the playlist. DO NOT print the dummy head node. main.cc #include #include "SongNode.h" // TODO: Write PrintPlaylist() function int main() { SongNode* headNode; SongNode* currNode; SongNode* lastNode; string songTitle; string songLength; string songArtist; // Front of nodes list headNode = new SongNode(); lastNode = headNode; // Read user input until -1 entered getline(cin, songTitle); while (songTitle != "-1") { getline(cin, songLength); getline(cin, songArtist); currNode = new SongNode(songTitle, songLength, songArtist); lastNode->InsertAfter(currNode); lastNode = currNode; getline(cin, songTitle); } // Print linked list cout << "LIST OF SONGS" << endl; cout << "-------------" << endl; PrintPlaylist(headNode); return 0; } SongNode.h #include "iostream" #include using namespace std; class SongNode { private: string songTitle; string songLength; string songArtist; SongNode* nextNodeRef; // Reference to the next node public: SongNode() { songTitle = ""; songLength = ""; songArtist = ""; nextNodeRef = NULL; } // Constructor SongNode(string songTitleInit, string songLengthInit, string songArtistInit); // Constructor SongNode(string songTitleInit, string songLengthInit, string songArtistInit, SongNode* nextLoc); // insertAfter void InsertAfter(SongNode* nodeLoc); // Get location pointed by nextNodeRef SongNode* GetNext(); // Prints song information void PrintSongInfo(); }; SongNode.cpp #include "SongNode.h" // Constructor SongNode::SongNode(string songTitleInit, string songLengthInit, string songArtistInit) { this->songTitle = songTitleInit; this->songLength = songLengthInit; this->songArtist = songArtistInit; this->nextNodeRef = NULL; } // Constructor SongNode::SongNode(string songTitleInit, string songLengthInit, string songArtistInit, SongNode* nextLoc) { this->songTitle = songTitleInit; this->songLength = songLengthInit; this->songArtist = songArtistInit; this->nextNodeRef = nextLoc; } // insertAfter void SongNode::InsertAfter(SongNode* nodeLoc) { SongNode* tmpNext; tmpNext = this->nextNodeRef; this->nextNodeRef = nodeLoc; nodeLoc->nextNodeRef = tmpNext; } // Get location pointed by nextNodeRef SongNode* SongNode::GetNext() { return this->nextNodeRef; } // TODO: Write PrintSongInfo() function PLEASE DO IN TO DO AND WRITE CODE C++. WRITE TEST INPUT SAME Expected output PLEASE
Given main(), complete the SongNode class to include the function PrintSongInfo(). Then write the PrintPlaylist() function in main.cpp to print all songs in the playlist. DO NOT print the dummy head node.
main.cc
#include <iostream>
#include "SongNode.h"
// TODO: Write PrintPlaylist() function
int main() {
SongNode* headNode;
SongNode* currNode;
SongNode* lastNode;
string songTitle;
string songLength;
string songArtist;
// Front of nodes list
headNode = new SongNode();
lastNode = headNode;
// Read user input until -1 entered
getline(cin, songTitle);
while (songTitle != "-1") {
getline(cin, songLength);
getline(cin, songArtist);
currNode = new SongNode(songTitle, songLength, songArtist);
lastNode->InsertAfter(currNode);
lastNode = currNode;
getline(cin, songTitle);
}
// Print linked list
cout << "LIST OF SONGS" << endl;
cout << "-------------" << endl;
PrintPlaylist(headNode);
return 0;
}
SongNode.h
#include "iostream"
#include <string>
using namespace std;
class SongNode {
private:
string songTitle;
string songLength;
string songArtist;
SongNode* nextNodeRef; // Reference to the next node
public:
SongNode() {
songTitle = "";
songLength = "";
songArtist = "";
nextNodeRef = NULL;
}
// Constructor
SongNode(string songTitleInit, string songLengthInit, string songArtistInit);
// Constructor
SongNode(string songTitleInit, string songLengthInit, string songArtistInit, SongNode* nextLoc);
// insertAfter
void InsertAfter(SongNode* nodeLoc);
// Get location pointed by nextNodeRef
SongNode* GetNext();
// Prints song information
void PrintSongInfo();
};
SongNode.cpp
#include "SongNode.h"
// Constructor
SongNode::SongNode(string songTitleInit, string songLengthInit, string songArtistInit) {
this->songTitle = songTitleInit;
this->songLength = songLengthInit;
this->songArtist = songArtistInit;
this->nextNodeRef = NULL;
}
// Constructor
SongNode::SongNode(string songTitleInit, string songLengthInit, string songArtistInit, SongNode* nextLoc) {
this->songTitle = songTitleInit;
this->songLength = songLengthInit;
this->songArtist = songArtistInit;
this->nextNodeRef = nextLoc;
}
// insertAfter
void SongNode::InsertAfter(SongNode* nodeLoc) {
SongNode* tmpNext;
tmpNext = this->nextNodeRef;
this->nextNodeRef = nodeLoc;
nodeLoc->nextNodeRef = tmpNext;
}
// Get location pointed by nextNodeRef
SongNode* SongNode::GetNext() {
return this->nextNodeRef;
}
// TODO: Write PrintSongInfo() function
PLEASE DO IN TO DO AND WRITE CODE C++. WRITE TEST INPUT SAME Expected output PLEASE
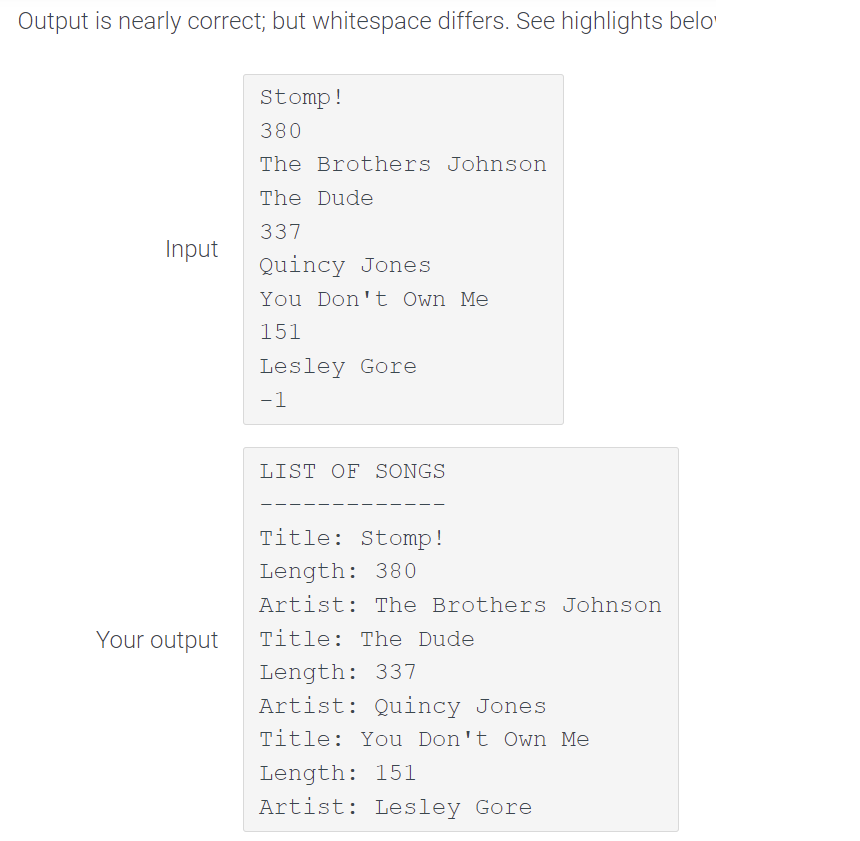
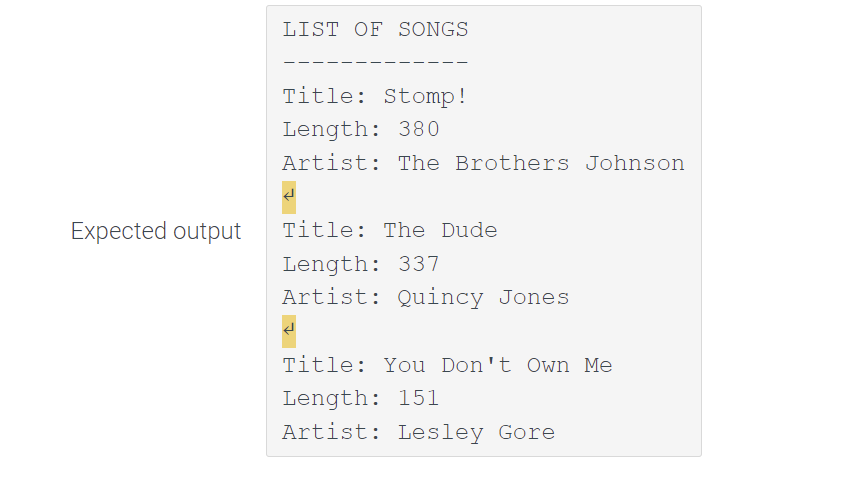

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

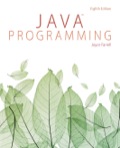
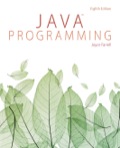