This is an object oriented programming problem. You will need to create three python files: main.py and airplane.py. You cannot import any sorting methods in this question. The file airplane.py contains an object called class Airplane that stores the ID number of a single airplane (named planelD) and the number of passengers (named numPassengers), the priority of the flight (named flightPriority) and the time of the flight (named flightTime) of that single airplane. The object has a constructor that assigns string planelD, integer numPassengers, integer flightPriority and integer flightTime with the values passed to it. For simplicity assue time is an integer number of this form: 2:30PM is recorded as integer 1430 using 24-hour time. Assume priority is an integer number between 0 and 10 with O being the highest priority and 10 the lowest priority. Write the methods named getPriority(), getTime() and getPlanelD() which return the corresponding class variable. The file main.py contains the main program. Do the following: 1. Create a list called planesList that stores Airplane objects. Initialize the list to empty. This variable represents a lineup of airplanes at a particular runway as they wait their turn to fly. 2. Write a function called addPlane(ID.passengerCount,priority,time) that instantiates and adds an Airplane object to planesList sorted by lineup position number. The list's index number is the airplane's lineup position on the runway as it waits its turn to fly. Index 0 is first in line, with index 1 being after that, etc. Each airplane must have a unique lineup position number. The position of the airplane is determined by this formula: earlier timed airplanes go before later timed airplanes, unless the times are the same, then the priority determines which goes first, unless the priority is the same, then it is ordered by last one first. 3. Call the function addPlan() four times adding 4 different airplanes to the list. 4. Print planesList to the screen in their flight position orde. Your program does not ask for input from the user. Everything is hard coded.
This is an object oriented programming problem. You will need to create three python files: main.py and airplane.py. You cannot import any sorting methods in this question. The file airplane.py contains an object called class Airplane that stores the ID number of a single airplane (named planelD) and the number of passengers (named numPassengers), the priority of the flight (named flightPriority) and the time of the flight (named flightTime) of that single airplane. The object has a constructor that assigns string planelD, integer numPassengers, integer flightPriority and integer flightTime with the values passed to it. For simplicity assue time is an integer number of this form: 2:30PM is recorded as integer 1430 using 24-hour time. Assume priority is an integer number between 0 and 10 with O being the highest priority and 10 the lowest priority. Write the methods named getPriority(), getTime() and getPlanelD() which return the corresponding class variable. The file main.py contains the main program. Do the following: 1. Create a list called planesList that stores Airplane objects. Initialize the list to empty. This variable represents a lineup of airplanes at a particular runway as they wait their turn to fly. 2. Write a function called addPlane(ID.passengerCount,priority,time) that instantiates and adds an Airplane object to planesList sorted by lineup position number. The list's index number is the airplane's lineup position on the runway as it waits its turn to fly. Index 0 is first in line, with index 1 being after that, etc. Each airplane must have a unique lineup position number. The position of the airplane is determined by this formula: earlier timed airplanes go before later timed airplanes, unless the times are the same, then the priority determines which goes first, unless the priority is the same, then it is ordered by last one first. 3. Call the function addPlan() four times adding 4 different airplanes to the list. 4. Print planesList to the screen in their flight position orde. Your program does not ask for input from the user. Everything is hard coded.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
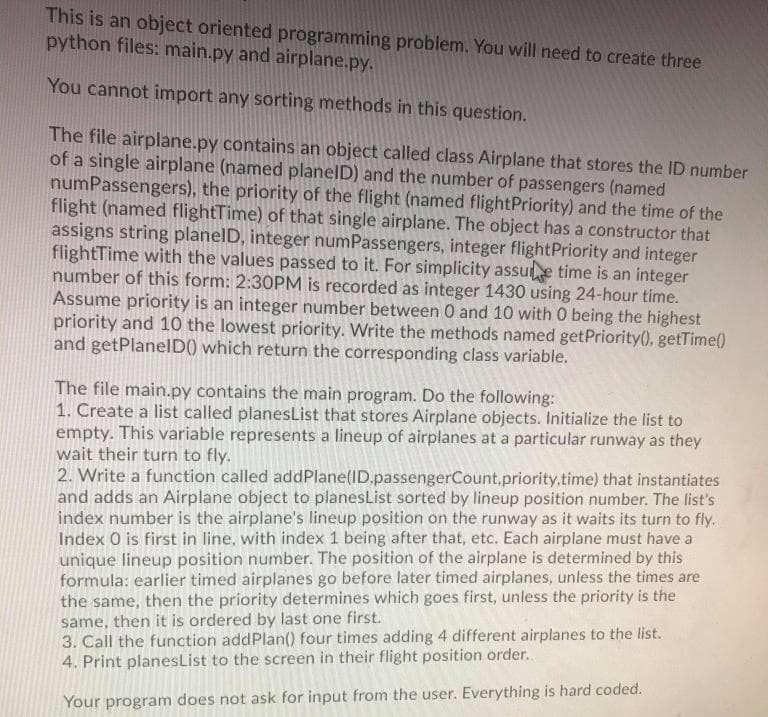
Transcribed Image Text:This is an object oriented programming problem. You will need to create three
python files: main.py and airplane.py.
You cannot import any sorting methods in this question.
The file airplane.py contains an object called class Airplane that stores the ID number
of a single airplane (named planelD) and the number of passengers (named
numPassengers), the priority of the flight (named flightPriority) and the time of the
flight (named flightTime) of that single airplane. The object has a constructor that
assigns string planelD, integer numPassengers, integer flightPriority and integer
flightTime with the values passed to it. For simplicity assue time is an integer
number of this form: 2:30PM is recorded as integer 1430 using 24-hour time.
Assume priority is an integer number between 0 and 10 with 0 being the highest
priority and 10 the lowest priority. Write the methods named getPriority(), getTime()
and getPlanelD() which return the corresponding class variable.
The file main.py contains the main program. Do the following:
1. Create a list called planesList that stores Airplane objects. Initialize the list to
empty. This variable represents a lineup of airplanes at a particular runway as they
wait their turn to fly.
2. Write a function called addPlane(ID.passengerCount,priority.time) that instantiates
and adds an Airplane object to planesList sorted by lineup position number. The list's
index number is the airplane's lineup position on the runway as it waits its turn to fly.
Index O is first in line, with index 1 being after that, etc. Each airplane must have a
unique lineup position number. The position of the airplane is determined by this
formula: earlier timed airplanes go before later timed airplanes, unless the times are
the same, then the priority determines which goes first, unless the priority is the
same, then it is ordered by last one first.
3. Call the function addPlan() four times adding 4 different airplanes to the list.
4. Print planesList to the screen in their flight position order.
Your program does not ask for input from the user. Everything is hard coded.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
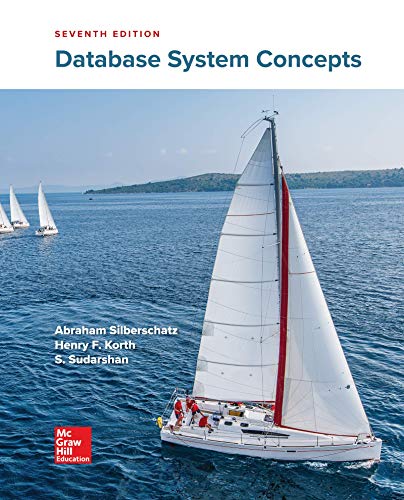
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
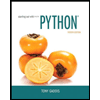
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
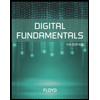
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
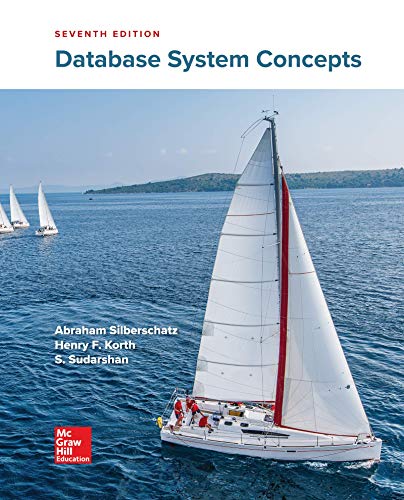
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
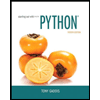
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
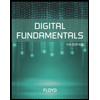
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
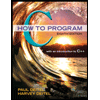
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
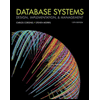
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
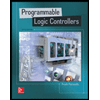
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education