This is the question - Write an application that displays a series of at least eight student ID numbers (that you have stored in an array) and asks the user to enter a test letter grade for the student. Create an Exception class named GradeException that contains a static public array of valid grade letters (A, B, C, D, F, and I) that you can use to determine whether a grade entered from the application is valid. In your application, throw a GradeException if the user does not enter a valid letter grade. Catch the GradeException, and then display the message Invalid grade. In addition, store an I (for Incomplete) for any student for whom an exception is caught. At the end of the application, display all the student IDs and grades. Code I was given, I will attach a screenshot of the errors- public class GradeException extends Exception { public GradeException(String string) { } } import java.util.*; public class TestGrade { public static void main(String args[]) throws Exception { Scanner input = new Scanner(System.in); int[] ids = { 1234, 1245, 1267, 1278, 2345, 1256, 3456, 3478, 4567, 5678 }; char[] grades = new char[10]; String gradeString = "ABCDFi"; final int HIGHLIMIT = 100; String inString, outString = ""; int flag = 0; for (int x = 0; x < ids.length; ++x) { System.out.println("Enter letter grade for student id number: " + ids[x]); inString = input.next().toUpperCase(); grades[x] = inString.charAt(0); if (!gradeString.contains(grades[x] + "")) { try { throw new GradeException(); } catch (GradeException e) { System.out.println("Invalid grade"); grades[x]='I'; } } } for (int x = 0; x < ids.length; ++x) outString = outString + "ID #" + ids[x] + " Grade " + grades[x] + "\n"; System.out.println(outString); } }
This is the question -
Write an application that displays a series of at least eight student ID numbers (that you have stored in an array) and asks the user to enter a test letter grade for the student.
Create an Exception class named GradeException that contains a static public array of valid grade letters (A, B, C, D, F, and I) that you can use to determine whether a grade entered from the application is valid. In your application, throw a GradeException if the user does not enter a valid letter grade. Catch the GradeException, and then display the message Invalid grade. In addition, store an I (for Incomplete) for any student for whom an exception is caught. At the end of the application, display all the student IDs and grades.
Code I was given, I will attach a screenshot of the errors-
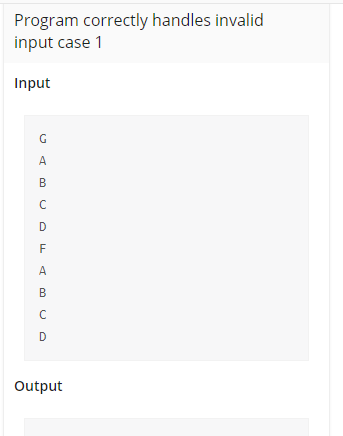
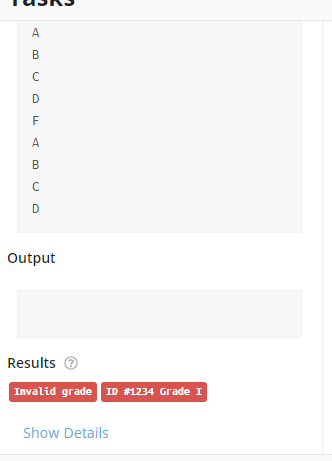

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

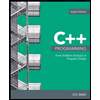
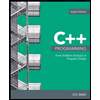