This lab will exercise your understanding of some of the concepts covered in Chapters 8 and 9: functions; scope; c++ READ THE FOLLOWING CAREFULLY TO GET FULL VALUE FROM THE PRACTICE. 1. Rewrite the processes into functions by performing the following(using the following program below): 2. Create int function(s) for opening the input and output files. These opens may be in the same function. This function MUST RETURN an int value to indicate the success or failure of the open(s). Test this value and take appropriate action after the function call. This function MUST have the following parameters, in any order: a. input file stream b. output file stream 3. Create a void function to read the data file. This function MUST have the following parameters, in any order: a. input file stream b. OUT parameter to return the total of all values c. OUT parameter to return the number of values read 4. Create a value returning function (I'm leaving it up to YOU to choose the correct type) to return the avg of the numbers read. This function MUST have the following parameters, in any order: a. IN parameter to pass in the total of the numbers read b. IN parameter to pass in the number of values read 5. Create void function(s) for closing the input and output files. These closes may be in the same function. This function MUST have the following parameters, in any order. a. input file stream b. output file stream 6. Move the global variables (defined before main in reWriteThis.cpp) and place them within the appropriate functions. Some will be needed with the related function, some will still be needed in main. 7. FOR PRACTICE, do NOT use the same variable names as ARGUMENTS in the function call and PARAMETERS in the function heading definition. 8. You MUST use function prototypes (before main) and you MUST define your functions AFTER main. 9. The console (cout) and file writes remain in main. Using the following program : #include #include #include #include // for system("pause") using namespace std; // global variables; are used anywhere within the program without re-definition string ifileName, ofileName; ifstream inData; ofstream outData; int numValues, total, i, num; float avg; int main () { ifileName = "inFile.txt"; inData.open(ifileName.c_str()); if (!inData) { cout << "Error opening file: " << ifileName << ". Exiting program..." << endl; return 0; } inData >> numValues; for (i = 0; i < numValues; i++) { inData >> num; total += num; } avg = float(total) / float(numValues); ofileName = "outFile.txt"; outData.open(ofileName.c_str()); if (!outData) { cout << "Error opening file: " << ofileName << ". Exiting program..." << endl; return 0; } cout << "You entered " << numValues << " numbers totaling " << total << " with a average of " << avg << endl; outData << "You entered " << numValues << " numbers totaling " << total << " with a average of " << avg << endl; inData.close(); outData.close(); // system("pause"); }
This lab will exercise your understanding of some of the
concepts covered in Chapters 8 and 9: functions; scope; c++
READ THE FOLLOWING CAREFULLY TO GET FULL VALUE FROM THE PRACTICE.
1. Rewrite the processes into functions by performing the following(using the following program below):
2. Create int function(s) for opening the input and output files. These
opens may be in the same function. This function MUST RETURN an int value
to indicate the success or failure of the open(s). Test this value and
take appropriate action after the function call. This function MUST have
the following parameters, in any order:
a. input file stream
b. output file stream
3. Create a void function to read the data file. This function MUST have the
following parameters, in any order:
a. input file stream
b. OUT parameter to return the total of all values
c. OUT parameter to return the number of values read
4. Create a value returning function (I'm leaving it up to YOU to choose
the correct type) to return the avg of the numbers read. This function
MUST have the following parameters, in any order:
a. IN parameter to pass in the total of the numbers read
b. IN parameter to pass in the number of values read
5. Create void function(s) for closing the input and output files. These
closes may be in the same function. This function MUST have the following
parameters, in any order.
a. input file stream
b. output file stream
6. Move the global variables (defined before main in reWriteThis.cpp)
and place them within the appropriate functions. Some will be
needed with the related function, some will still be needed in main.
7. FOR PRACTICE, do NOT use the same variable names as ARGUMENTS in the
function call and PARAMETERS in the function heading definition.
8. You MUST use function prototypes (before main) and you MUST define
your functions AFTER main.
9. The console (cout) and file writes remain in main.
Using the following program :
#include <iostream>
#include <string>
#include <fstream>
#include<cstdlib> // for system("pause")
using namespace std;
// global variables; are used anywhere within the program without re-definition
string ifileName, ofileName;
ifstream inData;
ofstream outData;
int numValues, total, i, num;
float avg;
int main () {
ifileName = "inFile.txt";
inData.open(ifileName.c_str());
if (!inData) {
cout << "Error opening file: " << ifileName << ". Exiting program..." << endl;
return 0;
}
inData >> numValues;
for (i = 0; i < numValues; i++) {
inData >> num;
total += num;
}
avg = float(total) / float(numValues);
ofileName = "outFile.txt";
outData.open(ofileName.c_str());
if (!outData) {
cout << "Error opening file: " << ofileName << ". Exiting program..." << endl;
return 0;
}
cout << "You entered " << numValues << " numbers totaling " << total << " with a average of " << avg << endl;
outData << "You entered " << numValues << " numbers totaling " << total << " with a average of " << avg << endl;
inData.close();
outData.close();
// system("pause");
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

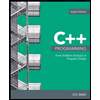
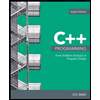