Write a C++ program with the following functions, with the correct number of arguments for each function, as well as any other functions used in class. void fillUpArray ( argument1, argument2) This function should read in a text file called "theVillagers.txt" (You may download it on Canvas). "theVillagers.txt" is a text file of numbers in ascending order, ranging anywhere from 1 to 2500, nonrepeating. Each number is separated by a new line character. The very first number in the text file is a number that refers to how many entries are in the rest of the text file. This function should populate an array with all the remaining entries in the text file, but it should NOT declare any arrays itself and it should not reference any global variables. void getInput(argument1, argument2) This function should read in a text file of the user's choosing into a given array. It should discard excess input. You may assume that there are always 2000 entries in this text file being entered. void processOne(argument1, argument2, argument3, argument4) This function should use one array as input and perform that many search queries on the other array using sequential search. Nothing is done with the results. void processTwo(argument1, argument2, argument3, argument4) This function should use one array as input and perform that many search queries on the other array using binary search. Nothing is done with the results. void printArray (argument1, argument2) This function should print out the contents of an array, with a space between each item. You should use the concept of partially filled arrays by declaring an array of some arbitrarily large size, and having a variable "numberUsed" that will eventually store the number of values in the array. Do this in the main function, and then make the appropriate function calls to accomplish the following task: After the array is populated, the program should then output the array and make the appropriate calls to get input from the user, and test the input array on both sequential and binary search. You should use the chrono library features as discussed in a previous lecture to time how long each process takes. Make sure your program runs at least twice, to see if there are fluctuations in time. Example Output: Here are the array contents: 1 2 3 4 5 6 7 8 9 25 40 55 (…) Enter a text file for input: input.txt
-Write a C++ program with the following functions, with the correct number of arguments for each function, as well as any other functions used in class.
- void fillUpArray ( argument1, argument2) This function should read in a text file called "theVillagers.txt" (You may download it on Canvas). "theVillagers.txt" is a text file of numbers in ascending order, ranging anywhere from 1 to 2500, nonrepeating. Each number is separated by a new line character. The very first number in the text file is a number that refers to how many entries are in the rest of the text file. This function should populate an array with all the remaining entries in the text file, but it should NOT declare any arrays itself and it should not reference any global variables.
- void getInput(argument1, argument2) This function should read in a text file of the user's choosing into a given array. It should discard excess input. You may assume that there are always 2000 entries in this text file being entered.
- void processOne(argument1, argument2, argument3, argument4) This function should use one array as input and perform that many search queries on the other array using sequential search. Nothing is done with the results.
- void processTwo(argument1, argument2, argument3, argument4) This function should use one array as input and perform that many search queries on the other array using binary search. Nothing is done with the results.
- void printArray (argument1, argument2) This function should print out the contents of an array, with a space between each item.
- You should use the concept of partially filled arrays by declaring an array of some arbitrarily large size, and having a variable "numberUsed" that will eventually store the number of values in the array. Do this in the main function, and then make the appropriate function calls to accomplish the following task:
- After the array is populated, the program should then output the array and make the appropriate calls to get input from the user, and test the input array on both sequential and binary search. You should use the chrono library features as discussed in a previous lecture to time how long each process takes.
- Make sure your program runs at least twice, to see if there are fluctuations in time.
Example Output:
Here are the array contents:
1 2 3 4 5 6 7 8 9 25 40 55 (…)
Enter a text file for input: input.txt
Sequential Search took 2.345 seconds.
Binary Search took 1.231 seconds.
I have done half. I need help with 2,3,and 4. I do not know what it means.Thank you!
#include <iostream>
#include <string>
#include <fstream>
#include <chrono>
using namespace std;
//FUNCTION DECLARATIONS:
void fillUpArrayV(int theArrayV[],int &NumberUsed);
void getInputV(int inputArray[],int &inputNumberUsed);
void printArray(int theArray[],int NumberUsed);
void processOne(int theArray[], int NumberUsed, int inputArray[], int inputNumberUsed);
int sequentialSearch(int theArray_V[],int NumberUsed,int input_Array_V[]);
int binarySearch(int theArrayV[],int NumberUsed,int input_Array[]);
int main()
{
int theArray[2500];
int NumberUsed=0;
int inputArray[2000];
int inputNumberUsed=0;
fillUpArrayV(theArray,NumberUsed);
printArray(theArray,NumberUsed);
getInputV(inputArray,inputNumberUsed);
sequentialSearch(theArray,inputNumberUsed,inputArray);
processOne(theArray,NumberUsed,inputArray,inputNumberUsed);
return 0;
}
void fillUpArrayV(int theArray[],int &NumberUsed)
{
ifstream inputFile ("theVillagers.txt");
if (inputFile.is_open())
{
inputFile>> NumberUsed ;
for(int i=0;i<NumberUsed;i++)
{
if ( i == NumberUsed )
break ;
inputFile>> theArray[i];
}
}
else if (inputFile.fail())
{
cout << "Cannot open file.\n";
exit(1);
}
}
void printArray(int theArray[],int NumberUsed)
{
{
cout << "Here are the array contents: ";
for(int i = 0 ; i < NumberUsed ; i++ )
cout << theArray[i] << " ";
cout<<endl<<endl;
}
}
void getInputV(int inputArray[],int &inputNumberUsed)
{
string input_file_string;
ifstream input_file;
cout<<"Enter a text file for input: ";
cin>>input_file_string;
if(input_file_string=="theVillagers.txt")
{
input_file.open("theVillagers.txt");
{
cout<<"YAY OPENED!"<<endl;
input_file>> inputNumberUsed ;
for(int i=0;i<inputNumberUsed;i++)
{
if ( i == inputNumberUsed )
break ;
input_file>> inputArray[i];
}
}
}
if(input_file.fail())
{
cout<<"Unable to open file."<<endl;
exit(1);
}
}
void processOne(int theArray[], int NumberUsed, int inputArray[], int inputNumberUsed) {
for (int c = 0;
c < 3; c++) //repeating the queries 3 times (or even more) can help with processors that are too fast
{
for (int i = 0; i < inputNumberUsed; i++) {
int index = sequentialSearch(theArray,NumberUsed,inputArray[]);
if (index == -1); //do nothing with the output
}
}
}
int sequentialSearch(int theArray_V[],int NumberUsed,int input_Array_V[])
{
for(int i=0;i<NumberUsed;i++)
{
if(theArray_V[i]==input_Array_V[i])
{
cout<<"Sequential Search: ";
return i;
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

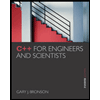
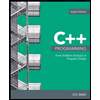
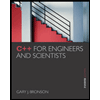
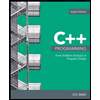