ties: The vector is three-dimensional, with default value equal to <0,0,0>. The + operator can be used between two vectors in order to perform vector addition. The * operator can be used between a vector and a number in order to perform vector scalar multiplication. Using print() on a vector will print its component values. See example below. The Vector class has a method cross_product() where a.cross_product(b) performs a cross product between vectors a and b and returns the resulting vector. The Vector class has a method dot_product() where a.dot_product(b) performs a dot product between vectors a and b and returns the resulting value. The Vector class has a method magnitude() where a.magnitude() returns the magnitude of vector a. You may use the math module for this exercise and nothing else. Example usage from vector import Vector v1 = Vector(1, 1, 0) # Initialize vector <1,1,0> v2 = Vector(2, 1, 3) # Initialize vector <2,1,3> print(v1) # Should print "<1,1,0>" print(v2) # Should print "<2,1,3>" v3 = v1 + v2 # v3 is <3,2,3> v4 = v1 * 3 # v4 is <3,3,0> v5 = v2 * 2 # v5 is <4,2,6> v1.cross_product(v2) # returns <3,-3,-1> v1.dot_product(v2) # returns 3
ties: The vector is three-dimensional, with default value equal to <0,0,0>. The + operator can be used between two vectors in order to perform vector addition. The * operator can be used between a vector and a number in order to perform vector scalar multiplication. Using print() on a vector will print its component values. See example below. The Vector class has a method cross_product() where a.cross_product(b) performs a cross product between vectors a and b and returns the resulting vector. The Vector class has a method dot_product() where a.dot_product(b) performs a dot product between vectors a and b and returns the resulting value. The Vector class has a method magnitude() where a.magnitude() returns the magnitude of vector a. You may use the math module for this exercise and nothing else. Example usage from vector import Vector v1 = Vector(1, 1, 0) # Initialize vector <1,1,0> v2 = Vector(2, 1, 3) # Initialize vector <2,1,3> print(v1) # Should print "<1,1,0>" print(v2) # Should print "<2,1,3>" v3 = v1 + v2 # v3 is <3,2,3> v4 = v1 * 3 # v4 is <3,3,0> v5 = v2 * 2 # v5 is <4,2,6> v1.cross_product(v2) # returns <3,-3,-1> v1.dot_product(v2) # returns 3
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter16: Searching, Sorting And Vector Type
Section: Chapter Questions
Problem 24SA
Related questions
Question
Create a module vector in a file called vector.py. The module contains the class Vector which has the following properties:
- The vector is three-dimensional, with default value <x,y,z> equal to <0,0,0>.
- The + operator can be used between two
vectors in order to perform vector addition. - The * operator can be used between a vector and a number in order to perform vector scalar multiplication.
- Using print() on a vector will print its component values. See example below.
- The Vector class has a method cross_product() where a.cross_product(b) performs a cross product between vectors a and b and returns the resulting vector.
- The Vector class has a method dot_product() where a.dot_product(b) performs a dot product between vectors a and b and returns the resulting value.
- The Vector class has a method magnitude() where a.magnitude() returns the magnitude of vector a.
You may use the math module for this exercise and nothing else.
Example usage
from vector import Vector
v1 = Vector(1, 1, 0) # Initialize vector <1,1,0>
v2 = Vector(2, 1, 3) # Initialize vector <2,1,3>
print(v1) # Should print "<1,1,0>"
print(v2) # Should print "<2,1,3>"
v3 = v1 + v2 # v3 is <3,2,3>
v4 = v1 * 3 # v4 is <3,3,0>
v5 = v2 * 2 # v5 is <4,2,6>
v1.cross_product(v2) # returns <3,-3,-1>
v1.dot_product(v2) # returns 3
v1.magnitude() # returns 1.4142135623730951
use python
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
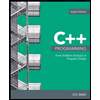
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
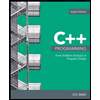
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning