C++ Provide a different implementation of ChoiceQuestion. Instead of storing the choices in a vector, the add_choice function should add the choice to the question text. For this purpose, an add_line function has been added to the Question class. Complete the following file: choicequestion.cpp Use the following files: choicequestion.h #ifndef CHOICEQUESTION_H #define CHOICEQUESTION_H #include using namespace std; #include "question.h" class ChoiceQuestion : public Question { public: /** Constructs a choice question with a given text and no choices. @param question_text the text of this question */ ChoiceQuestion(string question_text); /** Adds an answer choice to this question. @param choice the choice to add @param correct true if this is the correct choice, false otherwise */ void add_choice(string choice, bool correct); private: int num_choices; }; #endif question.h #ifndef QUESTION_H #define QUESTION_H #include using namespace std; class Question { public: /** Constructs a question with empty question and answer. */ Question(); /** @param line the line of text to add to this question */ void add_line(string line); /** @param correct_response the answer for this question */ void set_answer(string correct_response); /** @param response the response to check @return true if the response was correct, false otherwise */ virtual bool check_answer(string response) const; /** Displays this question. */ virtual void display() const; private: string text; string answer; }; #endif questiondemo.cpp
C++
Provide a different implementation of ChoiceQuestion. Instead of storing the choices in a
Complete the following file:
choicequestion.cpp
Use the following files:
choicequestion.h
#ifndef CHOICEQUESTION_H #define CHOICEQUESTION_H #include <string> using namespace std; #include "question.h" class ChoiceQuestion : public Question { public: /** Constructs a choice question with a given text and no choices. @param question_text the text of this question */ ChoiceQuestion(string question_text); /** Adds an answer choice to this question. @param choice the choice to add @param correct true if this is the correct choice, false otherwise */ void add_choice(string choice, bool correct); private: int num_choices; }; #endif
question.h
#ifndef QUESTION_H #define QUESTION_H #include <string> using namespace std; class Question { public: /** Constructs a question with empty question and answer. */ Question(); /** @param line the line of text to add to this question */ void add_line(string line); /** @param correct_response the answer for this question */ void set_answer(string correct_response); /** @param response the response to check @return true if the response was correct, false otherwise */ virtual bool check_answer(string response) const; /** Displays this question. */ virtual void display() const; private: string text; string answer; }; #endif
questiondemo.cpp
#include <iostream> #include <string> #include <sstream> using namespace std; #include "question.h" #include "choicequestion.h" int main() { string response; cout << boolalpha; // Make a quiz with two questions const int QUIZZES = 2; Question* quiz[QUIZZES]; quiz[0] = new Question; quiz[0]->add_line("Who was the inventor of C++?"); quiz[0]->set_answer("Bjarne Stroustrup"); ChoiceQuestion* cq_pointer = new ChoiceQuestion( "In which country was the inventor of C++ born?"); cq_pointer->add_choice("Australia", false); cq_pointer->add_choice("Denmark", true); cq_pointer->add_choice("Korea", false); cq_pointer->add_choice("United States", false); quiz[1] = cq_pointer; // Check answers for all questions for (int i = 0; i < QUIZZES; i++) { quiz[i]->display(); cout << "Your answer: "; getline(cin, response); cout << response << endl; cout << quiz[i]->check_answer(response) << endl; cout << endl; } return 0; }
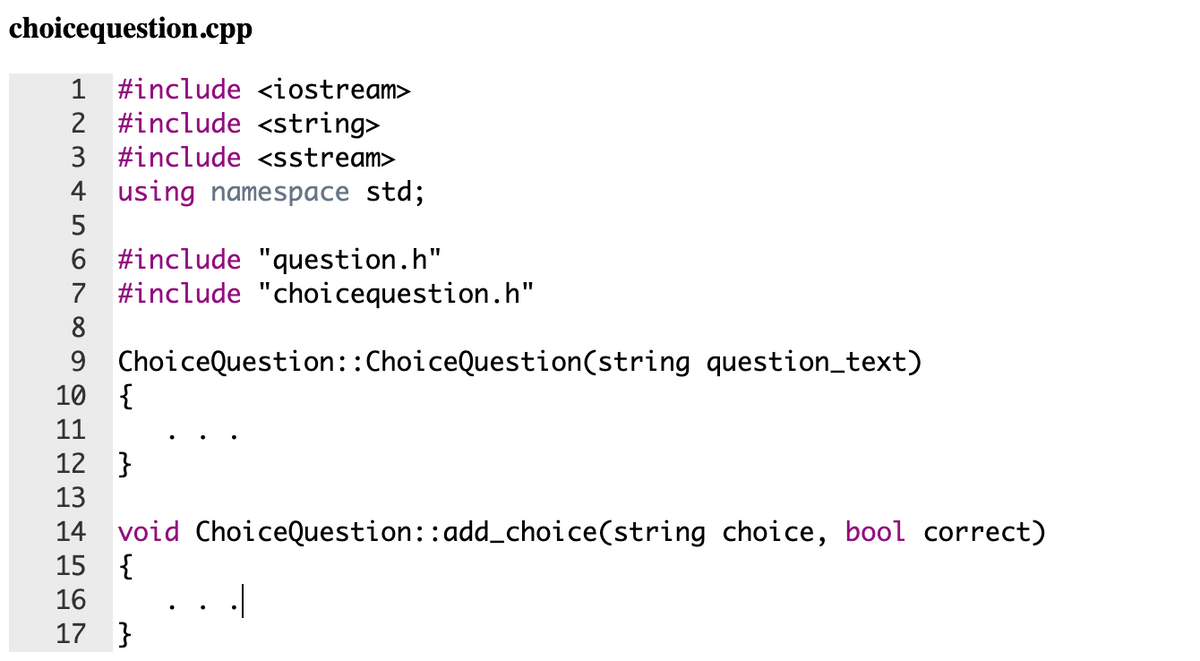

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

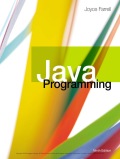
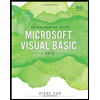
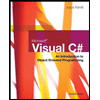
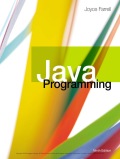
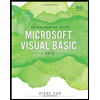
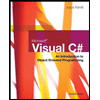
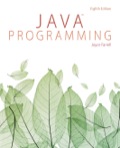
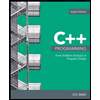