Create a Dog and a Cat class, each of which inherit from Animal. Each class should have a constructor which initialses their name and a hello method. The cat's hello method should return the string "Meow" and the dog's hello method should return the string "Woof". The Animal class is public abstract class Animal { public Animal(String n) name = n; } abstract String hello(); public String greeting() { return hello() + ", I am " + name; } // private private String name; } Your classes will be used in the following Main class. public class Main { public static void main(String () args) { Animal cat = new Cat("Angel"); Animal dog = new Dog("Fido"); System.out.println(cat.greeting()); System.out.println(dog.greeting()); } Note that your Cat and Dog classes must not supply a greeting method. They should use the greeting method from the Animal class.
Create a Dog and a Cat class, each of which inherit from Animal. Each class should have a constructor which initialses their name and a hello method. The cat's hello method should return the string "Meow" and the dog's hello method should return the string "Woof". The Animal class is public abstract class Animal { public Animal(String n) name = n; } abstract String hello(); public String greeting() { return hello() + ", I am " + name; } // private private String name; } Your classes will be used in the following Main class. public class Main { public static void main(String () args) { Animal cat = new Cat("Angel"); Animal dog = new Dog("Fido"); System.out.println(cat.greeting()); System.out.println(dog.greeting()); } Note that your Cat and Dog classes must not supply a greeting method. They should use the greeting method from the Animal class.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 2PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
![Create a Dog and a Cat class, each of which inherit from Animal. Each class should have a constructor which initialses their name and a hello method.
The cat's hello method should return the string "Meow" and the dog's hello method should return the string "Woof".
The Animal class is
public abstract class Animal
{
public Animal(String n)
{
name = n;
}
abstract String hello();
public String greeting()
{
return hello() + ", I am
+ name;
}
// private
private String name;
}
Your classes will be used in the following Main class.
public class Main
{
public static void main(String [] args)
{
Animal cat = new Cat("Angel");
Animal dog
= new Dog("Fido");
System.out.println(cat.greeting());
System.out.println(dog.greeting());
}
}
Note that your Cat and Dog classes must not supply a greeting method. They should use the greeting method from the Animal class.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1ef79fcc-aafc-4275-9b05-b685f97ef655%2F7128624b-be2a-4d10-bacf-bb8bf6c1dd0a%2Fn5z65aq_processed.png&w=3840&q=75)
Transcribed Image Text:Create a Dog and a Cat class, each of which inherit from Animal. Each class should have a constructor which initialses their name and a hello method.
The cat's hello method should return the string "Meow" and the dog's hello method should return the string "Woof".
The Animal class is
public abstract class Animal
{
public Animal(String n)
{
name = n;
}
abstract String hello();
public String greeting()
{
return hello() + ", I am
+ name;
}
// private
private String name;
}
Your classes will be used in the following Main class.
public class Main
{
public static void main(String [] args)
{
Animal cat = new Cat("Angel");
Animal dog
= new Dog("Fido");
System.out.println(cat.greeting());
System.out.println(dog.greeting());
}
}
Note that your Cat and Dog classes must not supply a greeting method. They should use the greeting method from the Animal class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
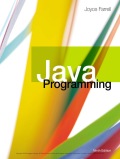
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
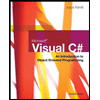
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
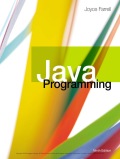
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
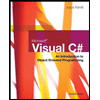
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,