Using the methods pickDir() and exractInt() as shown below, to write a simple automated game: how long does it take a robot to travel from a left line of a field to the right line? Assume field is 15 units apart (metres, kilometres, it doesn't matter), and the robot starts on the left line. // return string with random direction and random distance (within maxDist) public static String pickDir (int maxDist) { Random r = new Random(); // random number generator // return direction + distance return ( "" + "LRUD".charAt( r.nextInt(4) ) + (r.nextInt(maxDist) + 1) ); } // returns integer from string, from pos p1 to p2: str = "D1" - public static int extractInt (String str, int p1, int p2) { return ( Integer.parseInt( str.substring(p1, p2+1) )); } extractInt(str,1,1); Write the main() method that keeps looping until the roboť's random left and right movements have the robot move past the right line, using the following rules: starting travel distance (Trav) is zero (0)–the robot is starting on the left line maximum distance, when picking a random direction, is 9 units robot moves to the left (L) are negative integer distances (-) to the travel distance, and robot moves to the right (R) are positive integer distances (+) to the travel distance robot moves up (U) and down (D) do not affect the left-right travel distance-but they are still displayed keep looping while the robot's travel distance <= 15 units (i.e., stop when travel distance > 15 units) after the loop, display "robot has crossed the right line!"
Using the methods pickDir() and exractInt() as shown below, to write a simple automated game: how long does it take a robot to travel from a left line of a field to the right line? Assume field is 15 units apart (metres, kilometres, it doesn't matter), and the robot starts on the left line. // return string with random direction and random distance (within maxDist) public static String pickDir (int maxDist) { Random r = new Random(); // random number generator // return direction + distance return ( "" + "LRUD".charAt( r.nextInt(4) ) + (r.nextInt(maxDist) + 1) ); } // returns integer from string, from pos p1 to p2: str = "D1" - public static int extractInt (String str, int p1, int p2) { return ( Integer.parseInt( str.substring(p1, p2+1) )); } extractInt(str,1,1); Write the main() method that keeps looping until the roboť's random left and right movements have the robot move past the right line, using the following rules: starting travel distance (Trav) is zero (0)–the robot is starting on the left line maximum distance, when picking a random direction, is 9 units robot moves to the left (L) are negative integer distances (-) to the travel distance, and robot moves to the right (R) are positive integer distances (+) to the travel distance robot moves up (U) and down (D) do not affect the left-right travel distance-but they are still displayed keep looping while the robot's travel distance <= 15 units (i.e., stop when travel distance > 15 units) after the loop, display "robot has crossed the right line!"
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please check the image for the question and please provide explanation and comments :
an example run:
Robot Travelling
Trav: 0
Rand: L1
Trav: -1
Rand: R3
Trav: 2
Rand: L1
Trav: 1
Rand: D4
Trav: 1
Rand: R8
Trav: 9
Rand: U3
Trav: 9
Rand: L3
Trav: 6
Rand: U1
Trav: 6
Rand: R6
Trav: 12
Rand: D1
Trav: 12
Rand: L2
Trav: 10
Rand: R8
Trav: 18
Done—robot has crossed the right line!
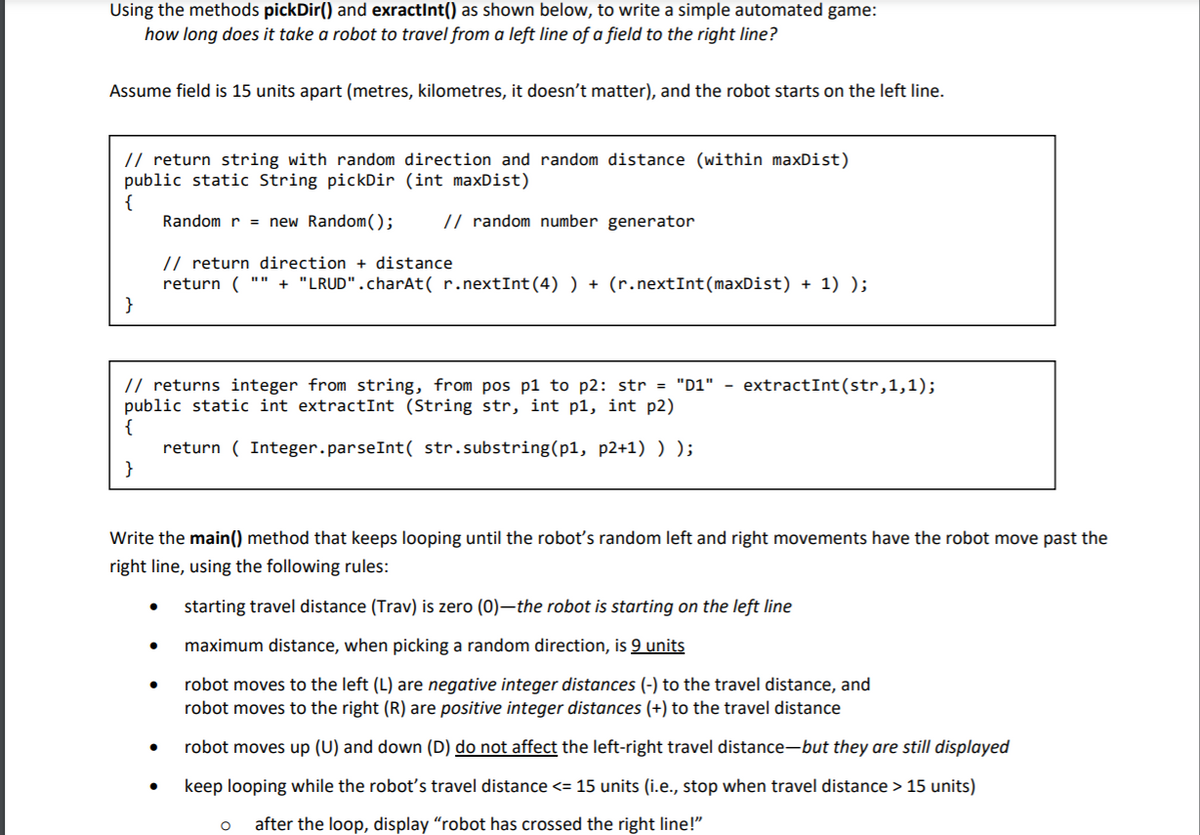
Transcribed Image Text:Using the methods pickDir() and exractInt() as shown below, to write a simple automated game:
how long does it take a robot to travel from a left line of a field to the right line?
Assume field is 15 units apart (metres, kilometres, it doesn't matter), and the robot starts on the left line.
// return string with random direction and random distance (within maxDist)
public static String pickDir (int maxDist)
{
Random r = new Random();
// random number generator
// return direction + distance
return ( "" + "LRUD".charAt( r.nextInt(4) ) + (r.nextInt(maxDist) + 1) );
}
// returns integer from string, from pos p1 to p2: str = "D1" -
public static int extractInt (String str, int p1, int p2)
{
return ( Integer.parseInt( str.substring(p1, p2+1) ) );
}
extractInt(str,1,1);
Write the main() method that keeps looping until the roboť's random left and right movements have the robot move past the
right line, using the following rules:
starting travel distance (Trav) is zero (0)–the robot is starting on the left line
maximum distance, when picking a random direction, is 9 units
robot moves to the left (L) are negative integer distances (-) to the travel distance, and
robot moves to the right (R) are positive integer distances (+) to the travel distance
robot moves up (U) and down (D) do not affect the left-right travel distance-but they are still displayed
keep looping while the robot's travel distance <= 15 units (i.e., stop when travel distance > 15 units)
after the loop, display "robot has crossed the right line!"
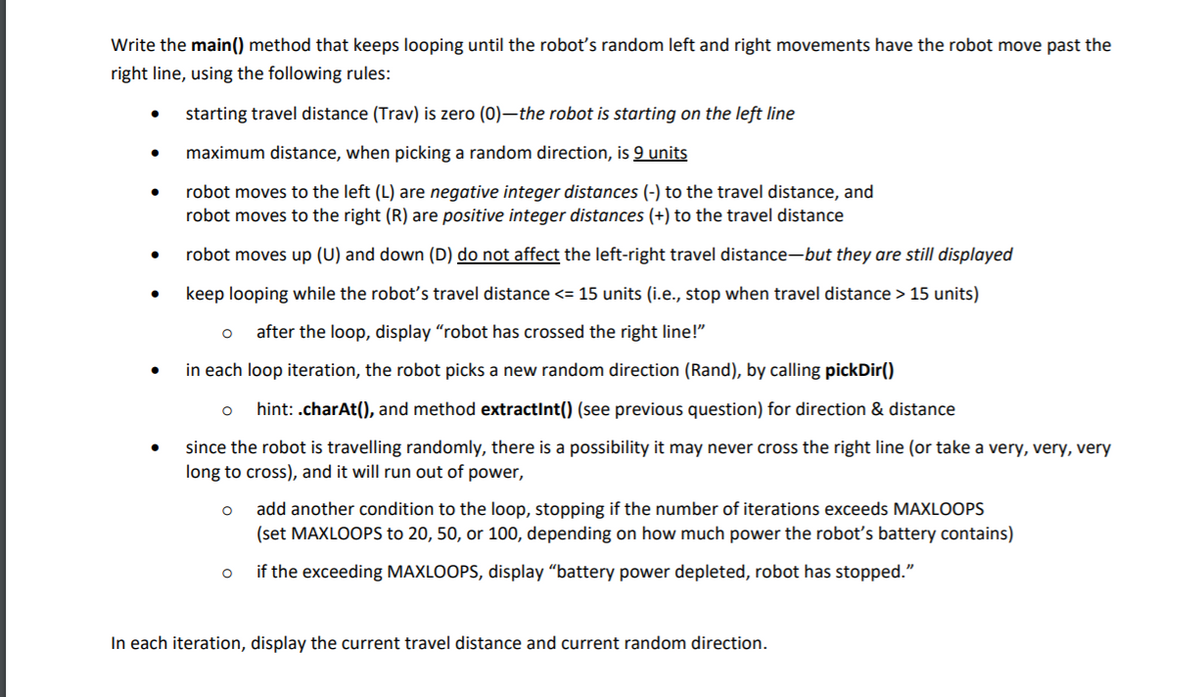
Transcribed Image Text:Write the main() method that keeps looping until the roboť's random left and right movements have the robot move past the
right line, using the following rules:
starting travel distance (Trav) is zero (0)–the robot is starting on the left line
maximum distance, when picking a random direction, is 9 units
robot moves to the left (L) are negative integer distances (-) to the travel distance, and
robot moves to the right (R) are positive integer distances (+) to the travel distance
robot moves up (U) and down (D) do not affect the left-right travel distance-but they are still displayed
keep looping while the robot's travel distance <= 15 units (i.e., stop when travel distance > 15 units)
after the loop, display "robot has crossed the right line!"
in each loop iteration, the robot picks a new random direction (Rand), by calling pickDir()
hint: .charAt(), and method extractInt() (see previous question) for direction & distance
since the robot is travelling randomly, there is a possibility it may never cross the right line (or take a very, very, very
long to cross), and it will run out of power,
add another condition to the loop, stopping if the number of iterations exceeds MAXLOOPS
(set MAXLOOPS to 20, 50, or 100, depending on how much power the robotť's battery contains)
if the exceeding MAXLOOPS, display "battery power depleted, robot has stopped."
In each iteration, display the current travel distance and current random direction.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
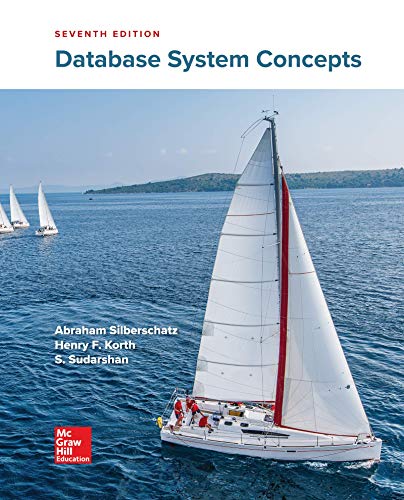
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
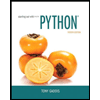
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
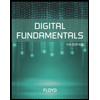
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
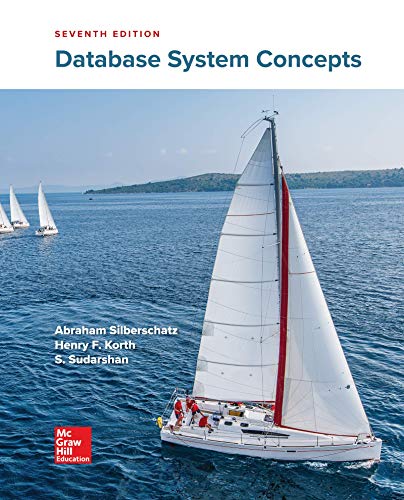
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
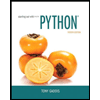
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
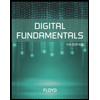
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
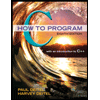
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
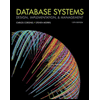
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
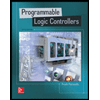
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education