Use C++ Programming language. The header file below defines a class for a simple hash table: hashMap.h Download hashMap.h Write a driver program to test the class with the following data: (520, "3100 Main St, Houston TX "); (37, "2200 Hayes Rd, Austin TX"); (226, "1775 West Airport St, San Antonio TX"); (273, "3322 Walnut Bend, Houston TX"); (491, "5778 Alabama, Waco TX"); (94, "3333 New St, Paris TX"); Make sure to test the find function for exiting and non-existing data 2. Modify the type of member key in class HashEntry from int to a string (this is useful is the key is e.g. the phone number or e-mail address). Use the string hash function discussed in class (see ppt notes) or search online for alternative functions that work on strings. 3. Modify the collision strategy of class HashMap to do separate chaining instead of linear probing. Be sure to modify the display function so that items in the bucket chains are also displayed in a pre-defined order for debugging purpose. 4. Add a delete function to class HashMap. Note: You may NOT use the C++ STL map data structure to implement the hash table in this assignment. Deliverables: a) Indicate which tasks you completed. b) Since the tasks above are cumulative, you only need to submit your code for the last task as indicated in (a). c) Submit screenshots of your program execution showing your program meets the specs of the last task completed.
Use C++ Programming language.
The header file below defines a class for a simple hash table:
- hashMap.h Download hashMap.h
- Write a driver program to test the class with the following data:
(520, "3100 Main St, Houston TX ");
(37, "2200 Hayes Rd, Austin TX");
(226, "1775 West Airport St, San Antonio TX");
(273, "3322 Walnut Bend, Houston TX");
(491, "5778 Alabama, Waco TX");
(94, "3333 New St, Paris TX");
Make sure to test the find function for exiting and non-existing data
2. Modify the type of member key in class HashEntry from int to a string (this is useful is the key is e.g. the phone number or e-mail address). Use the string hash function discussed in class (see ppt notes) or search online for alternative functions that work on strings.
3. Modify the collision strategy of class HashMap to do separate chaining instead of linear probing. Be sure to modify the display function so that items in the bucket chains are also displayed in a pre-defined order for debugging purpose.
4. Add a delete function to class HashMap.
Note: You may NOT use the C++ STL map data structure to implement the hash table in this assignment.
Deliverables:
a) Indicate which tasks you completed.
b) Since the tasks above are cumulative, you only need to submit your code for the last task as indicated in (a).
c) Submit screenshots of your program execution showing your program meets the specs of the last task completed.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

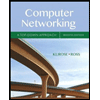
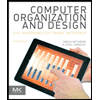
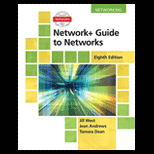
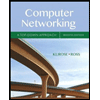
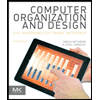
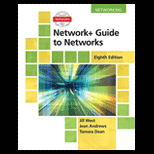
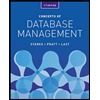
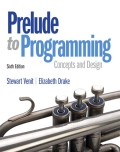
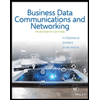