Please use the starter code below to answer the question in the screenshot. Please make sure to put the code in the solution and screenshot of the successful program run. public class Stack_Queue_Driver { // You would like a nice representation of a stack public static void main(String[] args) { // FOR OPTION 1 ArrayStack myStack = new ArrayStack(); myStack.push(1); myStack.push(2); myStack.push(3); myStack.push(4); myStack.push(5); myStack.push(6); myStack.push(7); myStack.push(8); myStack.push(9); System.out.println("My stack:"); displayS(myStack); System.out.println(); ArrayStack reversed = reverseStack(myStack); System.out.println("My reversed stack:"); displayS(reversed); System.out.println(); //----------------------- // FOR OPTION 2 ArrayQueue myQueue = new ArrayQueue(); myQueue.add(3); myQueue.add(5); myQueue.add(2); myQueue.add(1); myQueue.add(4); System.out.println("My queue:"); displayQ(myQueue); System.out.println(); ArrayQueue sortedQ = sortQueue(myQueue); System.out.println("My sorted queue:"); displayQ(sortedQ); System.out.println(); } // OPTION 1: Your code here to reverse the Stack: // Your code should work on a stack of any size. private static ArrayStack reverseStack(ArrayStack as) { // *** your code here *** return as; // you may change the return value } // You would like a nice representation of a stack // to be displayed to the console. This method is provided // and should work once reverseStack is implemented. private static void displayS(ArrayStack as) { // TODO Auto-generated method stub int numItems = as.size(); String toDisplay = "bottom~"; ArrayStack tempStack = reverseStack(as); for (int i = 0; i < numItems; i++) { toDisplay += tempStack.pop() + "~"; } for (int i = 0; i < toDisplay.length(); i++) { System.out.print(toDisplay.charAt(i)); } System.out.println("top"); } //--------------------------------------------------- // OPTION 2: Your code here to sort the Queue: // Your code should work on a queue of any size. // NOTE: I consider this to be the trickier problem. private static ArrayQueue sortQueue(ArrayQueue myQueue) { // TODO Auto-generated method stub return null; } // You would like a nice representation of a queue // to be displayed to the console. This method is provided // and should work once sortQueue is implemented. private static void displayQ(ArrayQueue aq) { // TODO Auto-generated method stub int numItems = aq.size(); System.out.print("front~"); for (int i = 0; i < numItems; i++) { int curr = aq.remove(); System.out.print(curr + "~"); aq.add(curr); } System.out.print("back"); System.out.println(); }
Please use the starter code below to answer the question in the screenshot. Please make sure to put the code in the solution and screenshot of the successful program run.
public class Stack_Queue_Driver {
// You would like a nice representation of a stack
public static void main(String[] args) {
// FOR OPTION 1
ArrayStack myStack = new ArrayStack();
myStack.push(1);
myStack.push(2);
myStack.push(3);
myStack.push(4);
myStack.push(5);
myStack.push(6);
myStack.push(7);
myStack.push(8);
myStack.push(9);
System.out.println("My stack:");
displayS(myStack);
System.out.println();
ArrayStack reversed = reverseStack(myStack);
System.out.println("My reversed stack:");
displayS(reversed);
System.out.println();
//-----------------------
// FOR OPTION 2
ArrayQueue<Integer> myQueue = new ArrayQueue<Integer>();
myQueue.add(3);
myQueue.add(5);
myQueue.add(2);
myQueue.add(1);
myQueue.add(4);
System.out.println("My queue:");
displayQ(myQueue);
System.out.println();
ArrayQueue<Integer> sortedQ = sortQueue(myQueue);
System.out.println("My sorted queue:");
displayQ(sortedQ);
System.out.println();
}
// OPTION 1: Your code here to reverse the Stack:
// Your code should work on a stack of any size.
private static ArrayStack reverseStack(ArrayStack as) {
// *** your code here ***
return as; // you may change the return value
}
// You would like a nice representation of a stack
// to be displayed to the console. This method is provided
// and should work once reverseStack is implemented.
private static void displayS(ArrayStack as) {
// TODO Auto-generated method stub
int numItems = as.size();
String toDisplay = "bottom~";
ArrayStack tempStack = reverseStack(as);
for (int i = 0; i < numItems; i++) {
toDisplay += tempStack.pop() + "~";
}
for (int i = 0; i < toDisplay.length(); i++) {
System.out.print(toDisplay.charAt(i));
}
System.out.println("top");
}
//---------------------------------------------------
// OPTION 2: Your code here to sort the Queue:
// Your code should work on a queue of any size.
// NOTE: I consider this to be the trickier problem.
private static ArrayQueue<Integer> sortQueue(ArrayQueue<Integer> myQueue) {
// TODO Auto-generated method stub
return null;
}
// You would like a nice representation of a queue
// to be displayed to the console. This method is provided
// and should work once sortQueue is implemented.
private static void displayQ(ArrayQueue<Integer> aq) {
// TODO Auto-generated method stub
int numItems = aq.size();
System.out.print("front~");
for (int i = 0; i < numItems; i++) {
int curr = aq.remove();
System.out.print(curr + "~");
aq.add(curr);
}
System.out.print("back");
System.out.println();
}
}
![• You are only allowed to use integer arrays and ArrayStack data structures. You can step through the array from
index 0 to n only. Given an array of numbers from 1 to 9, in order, reverse the array so that the numbers are now in
decreasing order.
You have been given an ArrayQueue to which the following numbers have been added: 3, 5, 2, 1, 4. Using only the
commands to add and remove values from the queue, sort the ArrayQueue (you can use variables to store some
information and you may create more ArrayQueue structures, but no other data storage structures). I consider this
the harder of the two tasks.
The output of the program with both problems solved should be:
<terminated> Stack_Queue_Solutions [Java Application]
OPTION 1
My stack:
bottom-1~2~3~4~5~6~7~8~9~top
My reversed stack:
bottom-9~8~7~6~5~4~3~2~1~top
OPTION 2
My queue:
front~3~5~2~1~4~back
My sorted queue:
front~1~2~3~4~5~back](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4cd974de-0ca5-43c4-9c5f-a02c9b7b2d9a%2F65f479da-4101-4c7e-a127-1d8668b97475%2F7d16j9_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

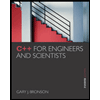
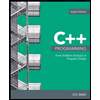
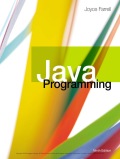
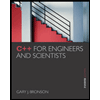
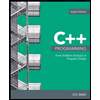
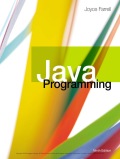
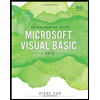