This is Java Programming Linked List Basics ( insertFront, insertBack, deleteFront & deleteBack) Question: Given the following code in main and output, please write the Java program which allows main to work: public static void main(String[] args) { LinkedList list = new LinkedList(); // Try to delete from an empty list list.deleteFront(); list.displayLL(); list.deleteBack(); list.displayLL(); // insert 3 nodes list.insert(18); list.insert(45); list.insert(12); list.displayLL(); // Here are the main “new” lines, not included in code I gave you list.insertFront(1); list.displayLL(); list.insertBack(999); list.displayLL(); list.deleteFront(); list.displayLL(); list.deleteBack(); list.displayLL(); } // end of main This is Java programming ple
This is Java
Linked List Basics ( insertFront, insertBack, deleteFront & deleteBack)
Question: Given the following code in main and output, please write the Java program which allows main to work:
public static void main(String[] args) {
LinkedList list = new LinkedList();
// Try to delete from an empty list
list.deleteFront();
list.displayLL();
list.deleteBack();
list.displayLL();
// insert 3 nodes
list.insert(18);
list.insert(45);
list.insert(12);
list.displayLL();
// Here are the main “new” lines, not included in code I gave you
list.insertFront(1);
list.displayLL();
list.insertBack(999);
list.displayLL();
list.deleteFront();
list.displayLL();
list.deleteBack();
list.displayLL();
} // end of main
This is Java programming please

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

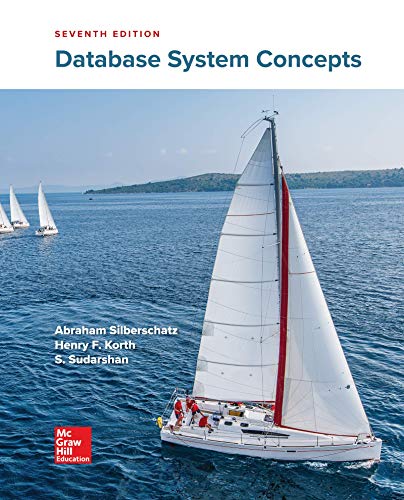
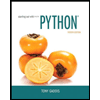
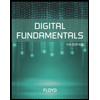
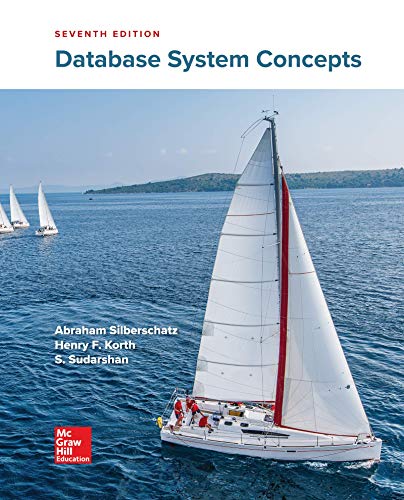
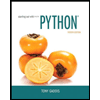
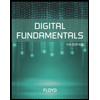
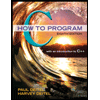
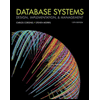
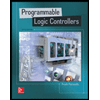