using C ++ (0) add 'public', 'private', 'friend' separate into .h and .cpp files "Vec.cpp" "Vec.h" "main.cpp" (1) Vec operator+(Vec a, Vec b) [1,2,3, 4] + [4,5,6, 8] = [5,7,9, 12] (2) operator* 1) Vec operator*(Vec a, int n) [1,2,3] * 4 = [4,8,12] 2) Vec operator*(int n, Vec a) 4 * [1,2,3] = [4,8,12] 3) int operator*(Vec a, Vec b) (check dot product) [1,2,3] * [2,3,4] = 1*2+2*3+3*4 = 20 (3) operator[] (cout << v.at(3);) cout << v[3] << endl; v[2] = 3; (4) operator<,>,<=,>=,==,!= [1,2,3] == [1,2,3] [1,0] < [1,0,1] < [1,1] < [1,2,3] < [1,2,3,5] < [1,2,4] < [1,2,6] < [1,3] < hallo < hell < hello < hf e.g. bool operator<(Vec a, Vec b) { ... } (5) ostream& operator<<(...) cout << v << endl; output: [ 3, 1, 4, 2 ] */ Vec operator+(Vec a, Vec b) { if ( a.size() != b.size() ) { cout << "[ERR] two vector's size are different!" << endl; Vec c; return c; } Vec c; for ( int i = 0; i < a.size(); i++ ) { c.push_back(a.at(i) + b.at(i)); } return c; } Vec operator*(Vec a, int n ) { Vec c; for ( int i = 0; i < a.size(); i++ ) { c.push_back(a.at(i)*n); } return c; } bool operator<(Vec a, Vec b) { int min_size = min(a.size(), b.size()); for ( int i = 0; i < min_size; i++ ) { if ( a.at(i) < b.at(i) ) return true; } } int main() { return 0; } following steps #include using namespace std; class Vec{ private: int x; int z; int y; public: Vec() { x=0; z=0; y=0; } Vec (int a, int b, int c) { x=a; z=b; y=c; } void printVec() { cout<<"["<
using C ++
(0) add 'public', 'private', 'friend'
separate into .h and .cpp files
"Vec.cpp" "Vec.h" "main.cpp"
(1) Vec operator+(Vec a, Vec b)
[1,2,3, 4] + [4,5,6, 8] = [5,7,9, 12]
(2) operator*
1) Vec operator*(Vec a, int n)
[1,2,3] * 4 = [4,8,12]
2) Vec operator*(int n, Vec a)
4 * [1,2,3] = [4,8,12]
3) int operator*(Vec a, Vec b) (check dot product)
[1,2,3] * [2,3,4] = 1*2+2*3+3*4 = 20
(3) operator[] (cout << v.at(3);)
cout << v[3] << endl;
v[2] = 3;
(4) operator<,>,<=,>=,==,!=
[1,2,3] == [1,2,3]
[1,0] < [1,0,1] < [1,1] < [1,2,3] < [1,2,3,5] < [1,2,4] < [1,2,6] < [1,3]
< hallo < hell < hello < hf
e.g. bool operator<(Vec a, Vec b) { ... }
(5) ostream& operator<<(...)
cout << v << endl; output: [ 3, 1, 4, 2 ]
*/
Vec operator+(Vec a, Vec b)
{
if ( a.size() != b.size() )
{
cout << "[ERR] two
Vec c;
return c;
}
Vec c;
for ( int i = 0; i < a.size(); i++ )
{
c.push_back(a.at(i) + b.at(i));
}
return c;
}
Vec operator*(Vec a, int n )
{
Vec c;
for ( int i = 0; i < a.size(); i++ )
{
c.push_back(a.at(i)*n);
}
return c;
}
bool operator<(Vec a, Vec b)
{
int min_size = min(a.size(), b.size());
for ( int i = 0; i < min_size; i++ )
{
if ( a.at(i) < b.at(i) ) return true;
}
}
int main()
{
return 0;
}
following steps
#include <iostream>
using namespace std;
class Vec{
private:
int x;
int z;
int y;
public:
Vec()
{
x=0;
z=0;
y=0;
}
Vec (int a, int b, int c)
{
x=a;
z=b;
y=c;
}
void printVec()
{
cout<<"["<<x<<","<<z<<","<<y<<"]"<<endl;
}
Vec operator +(Vec &op2);
Vec operator *(Vec &op2);
};
Vec Vec::operator+(Vec &op2)
{
op2.x= x + op2.x;
op2.z= z + op2.z;
op2.y= y+ op2.y;
return op2;
}
Vec Vec::operator*(Vec &op2)
{
op2.x= x *4;
return op2;
}
int main() {
Vec obj1(4,6,8),obj2(9,8,6), obj3, obj4;
obj3= obj1+obj2;
obj3.printVec();
obj4.printVec();
return 0;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

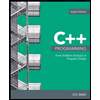
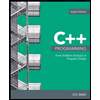