Extend the “Circle” class to have the following features. Two additional data members (integers) to store the x and y coordinate of the center of the Circle. A parameterized constructor. Set() and Get() methods for each of the data members. A function to display the data members of the class. A function enlargeCirlce(int); which increases the radius of the circle by the factor passed as input to the For example, if “c” is an object of class Circle, the statement c.enlargeCircle(2); should double the radius of the circle. A function moveHorizontal(int); which should move the center of the circle in the horizontal direction. For example, if “c” is a circle with center at (5,10), moveHorizontal(3); should move the center to position (8,10). A fuction moveVertical(int); similar to the moveHorizontal(int); function.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Task 1:
Extend the “Circle” class to have the following features.
- Two additional data members (integers) to store the x and y coordinate of the center of the Circle.
- A parameterized constructor.
- Set() and Get() methods for each of the data members.
- A function to display the data members of the class.
- A function enlargeCirlce(int); which increases the radius of the circle by the factor passed as input to the For example, if “c” is an object of class Circle, the statement c.enlargeCircle(2); should double the radius of the circle.
- A function moveHorizontal(int); which should move the center of the circle in the horizontal direction. For example, if “c” is a circle with center at (5,10), moveHorizontal(3); should move the center to position (8,10).
- A fuction moveVertical(int); similar to the moveHorizontal(int); function.
In the main program, create two objects of class Circle. Find which of the two circles is smaller (by comparing their radii) and enlarge the smaller circle by a factor of 2.
Code:
#include<iostream>
using namespace std;
//Class which holds properties and methods of a class
class Circle {
private:
int radius;
public:
//Parameterized constructor that creates an object
Circle(int r=0) {radius=r;}
//Member function to find area
int area(){
return 22*radius*radius/7;
}
//Member function to find circumference
int circumference(){
return 2*22*radius/7;
}
//Member function to print results
void print(int ar, int cf)
{
cout<<"Area of circle with radius "<<radius<<" is "<<ar<<" and circumference of circle is "<<cf<<endl;
}
};
int main()
{
Circle c1(49),c2(61); //Creating two objects of class circle
int ar1=c1.area();
int cf1=c1.circumference();
c1.print(ar1,cf1);
int ar2=c2.area();
int cf2=c2.circumference();
c2.print(ar2,cf2);
return 0;
}

Step by step
Solved in 2 steps

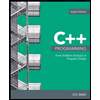
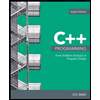