*USING JAVA* Now, follow the instructions below to create a new program named AriannasAppliances that will ask the user to enter the quarterly sales revenues for each of five appliance types sold by AriannasAppliances. It will then compute the annual sales revenues for each appliance type, total annual sales revenues for the company, and the percentage of the total sales revenues for each appliance type. Open Eclipse. Create a New -> Java project. Name it Chap4-AriannasAppliances and click Finish and OK. Next, create a New -> Class. Name it AriannasAppliances and be sure to check the first checkbox under "Which method stubs would you like to create?" and click Finish. Delete the green comment text in the file "AriannasAppliances.java" Add a comment at the top // (your name) Add another comment at the top // Project 4 Add code to the file "AriannasAppliances.java" to create a program that will do the following: use a for-loop to ask the user to enter the quarterly revenues for each appliance type write this information to a file named "AriannasAppliances2022Revenues.txt" as shown below (5 values per line) close the file, this will cause Java to save this file in your project folder Use a while-loop to read back the data from the file "AriannasAppliances2022Revenues.txt" and tally the revenues generated by each appliance type, use while ( inputFile.hasNext() ) Tally the total revenues for the year Report the revenues and the percentage of the total revenues for each appliance type, and the total revenues for the year as shown below *Requirement 1: Use the printf statement to format your monetary values to 2 decimal places. (see Chapter 3 for help as needed) *Requirement 2: Test your program to be sure it runs properly and produces all of the output shown below Be sure to check your code against the code submission checklist before submitting to Blackboard. Write the data to the file using the following format: (quarter1) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales (quarter2) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales (quarter3) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales (quarter4) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales After writing to the file, it should look like this (no text or commas, just the 5 values per line): Your program should produce the following output: ****** Welcome to Ariannas Appliances ****** This program will determine the annual sales revenues for Ariannas Appliances. It will report the sales revenues for each appliance, percentage of total revenues generated by each appliance, and the total sales revenues for 2022. Enter the revenue sales for 2022 Quarter 1 Dishwashers: 15000 Washers: 16000 Dryers: 17000 Refridgerators: 18000 Ovens: 19000 Enter the revenue sales for 2022 Quarter 2 Dishwashers: 10000 Washers: 11000 Dryers: 12000 Refridgerators: 13000 Ovens: 14000 Enter the revenue sales for 2022 Quarter 3 Dishwashers: 20000 Washers: 21000 Dryers: 22000 Refridgerators: 23000 Ovens: 24000 Enter the revenue sales for 2022 Quarter 4 Dishwashers: 18000 Washers: 19000 Dryers: 20000 Refridgerators: 21000 Ovens: 22000 ***** Ariannas Appliances 2022 ***** Dishwasher Revenues: $63,000.00 (17.75%) Washer Revenues: $67,000.00 (18.87%) Dryer Revenues: $71,000.00 (20.00%) Refridgerator Revenues: $75,000.00 (21.13%) Oven Revenues: $79,000.00 (22.25%) Ariannas Appliances Total Sales Revenues for 2022: $355,000.00
*USING JAVA*
Now, follow the instructions below to create a new
-
-
- Open Eclipse.
- Create a New -> Java project. Name it Chap4-AriannasAppliances and click Finish and OK.
- Next, create a New -> Class. Name it AriannasAppliances and be sure to check the first checkbox under "Which method stubs would you like to create?" and click Finish.
- Delete the green comment text in the file "AriannasAppliances.java"
- Add a comment at the top // (your name)
- Add another comment at the top // Project 4
- Add code to the file "AriannasAppliances.java" to create a program that will do the following:
- use a for-loop to ask the user to enter the quarterly revenues for each appliance type
- write this information to a file named "AriannasAppliances2022Revenues.txt" as shown below (5 values per line)
- close the file, this will cause Java to save this file in your project folder
- Use a while-loop to read back the data from the file "AriannasAppliances2022Revenues.txt" and tally the revenues generated by each appliance type, use while ( inputFile.hasNext() )
- Tally the total revenues for the year
- Report the revenues and the percentage of the total revenues for each appliance type, and the total revenues for the year as shown below
-
*Requirement 1: Use the printf statement to format your monetary values to 2 decimal places. (see Chapter 3 for help as needed)
*Requirement 2: Test your program to be sure it runs properly and produces all of the output shown below
Be sure to check your code against the code submission checklist before submitting to Blackboard.
Write the data to the file using the following format:
(quarter1) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales
(quarter2) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales
(quarter3) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales
(quarter4) dishwasherSales washerSales dryerSales refridgeratorSales ovenSales
After writing to the file, it should look like this (no text or commas, just the 5 values per line):
Your program should produce the following output:
****** Welcome to Ariannas Appliances ******
This program will determine the annual sales revenues for Ariannas Appliances.
It will report the sales revenues for each appliance, percentage of total revenues generated by each appliance,
and the total sales revenues for 2022.
Enter the revenue sales for 2022 Quarter 1
Dishwashers: 15000
Washers: 16000
Dryers: 17000
Refridgerators: 18000
Ovens: 19000
Enter the revenue sales for 2022 Quarter 2
Dishwashers: 10000
Washers: 11000
Dryers: 12000
Refridgerators: 13000
Ovens: 14000
Enter the revenue sales for 2022 Quarter 3
Dishwashers: 20000
Washers: 21000
Dryers: 22000
Refridgerators: 23000
Ovens: 24000
Enter the revenue sales for 2022 Quarter 4
Dishwashers: 18000
Washers: 19000
Dryers: 20000
Refridgerators: 21000
Ovens: 22000
***** Ariannas Appliances 2022 *****
Dishwasher Revenues: $63,000.00 (17.75%)
Washer Revenues: $67,000.00 (18.87%)
Dryer Revenues: $71,000.00 (20.00%)
Refridgerator Revenues: $75,000.00 (21.13%)
Oven Revenues: $79,000.00 (22.25%)
Ariannas Appliances Total Sales Revenues for 2022: $355,000.00

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

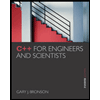
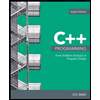
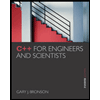
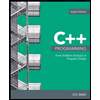