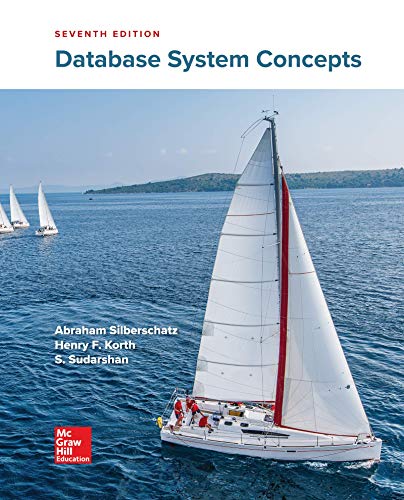
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Use Java please.
At the beginning of the program, implement a selection menu that prompts the user to select between two options (the two options being "7 guesses" and "user guesses" shown in the two pictures below).
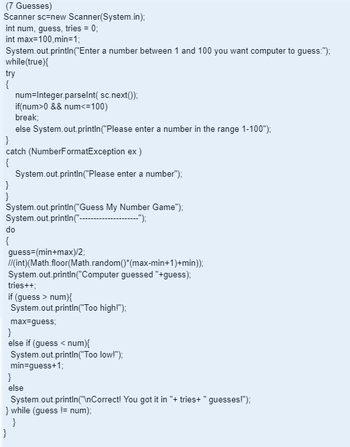
Transcribed Image Text:## Number Guessing Game Code Explanation
### Overview
The following Java code implements a simple number guessing game. The program prompts the user to select a number between 1 and 100. It then uses a binary search strategy to guess the number, displaying whether each guess is too high or too low until the correct number is identified.
### Code Breakdown
1. **Initialization:**
```java
Scanner sc = new Scanner(System.in);
int num, guess, tries = 0;
int max = 100, min = 1;
```
- **Scanner Creation:** A `Scanner` object is created to read user input.
- **Variables:** `num` to store the user's number, `guess` for the computer's guess, and `tries` to count the number of guesses. `max` and `min` set the range for guessing.
2. **User Input:**
```java
System.out.println("Enter a number between 1 and 100 you want computer to guess:");
while (true) {
num = Integer.parseInt(sc.next());
if (num > 0 && num <= 100)
break;
else
System.out.println("Please enter a number in the range 1-100");
}
```
- **Prompt User:** Asks the user to enter a number between 1 and 100.
- **Validation Loop:** Ensures the number is within the specified range. Continues prompting if the input is invalid.
3. **Guessing Logic:**
```java
System.out.println("Guess My Number Game");
System.out.println("-------------------");
do {
guess = (min + max) / 2;
System.out.println("Computer guessed " + guess);
tries++;
if (guess > num) {
System.out.println("Too high!");
max = guess;
} else if (guess < num) {
System.out.println("Too low!");
min = guess + 1;
} else {
System.out.println("\nCorrect! You got it in " + tries + " guesses!");
}
} while (guess != num);
```
- **Binary Search Approach:** The guess is calculated as the midpoint of the current `min` and `max`.
- **Guess Feedback:** Prints whether the guess is "Too high" or "Too low" and
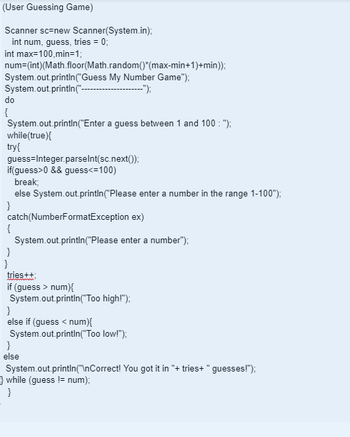
Transcribed Image Text:# User Guessing Game
This Java program is designed as a simple number-guessing game. It challenges users to guess a randomly generated number between 1 and 100. The program provides feedback if the user's guess is too high, too low, or correct.
### Code Explanation:
- **Initialization:**
- A `Scanner` object `sc` is created for capturing user input.
- Variables `num`, `guess`, and `tries` are initialized, with `num` storing the randomly generated number, `guess` capturing user inputs, and `tries` counting the number of attempts made.
- **Random Number Generation:**
- The random number is generated using `Math.random()` and stored in `num`. The range is set between `min` (1) and `max` (100).
- **Game Loop:**
- The program starts by displaying "Guess My Number Game" and prompts the user to enter a guess between 1 and 100.
- A `do-while` loop ensures the game continues until the correct number is guessed.
- **Input Handling:**
- Inside a `while(true)` loop, user inputs are taken and parsed as integers.
- A `try-catch` block manages exceptions if the input is not a number.
- **Condition Checks:**
- If the guess is out of range (not between 1 and 100), the user is asked to enter a valid number.
- After every valid input, `tries` is incremented.
- **Feedback:**
- If the user’s guess is higher than the generated number, "Too high!" is printed.
- If the guess is lower, "Too low!" is printed.
- If the guess is correct, the program congratulates the user and displays the number of guesses made.
This structure provides a simple yet instructive example of loop control, input validation, and exception handling in Java.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- USING JAVA: Write a program that analyzes an object falling for 10 seconds. It should contain main and two additional methods. One of the additional methods should return the distance an object falls in meters when passed the current second as an argument. See the formula needed below. The third method should convert meters to feet. You can look up the conversion factor needed online. The main method should use one loop to call the other methods and generate a table as shown below. The table should be displayed in formatted columns with decimals as shown.arrow_forwardAny help with this first problem would be really helpful! Thank you so much!! 1. Write the following exercises using Java code. Add comments in each program to explain what your code is doing. A. Write a program that generates two random integers, both in the range 50 to 100, inclusive. Use the Math class. Print both integers and then display the positive difference between the two integers, but use a selection. Do not use the absolute value method of the Math class. B. Duke Shirts sells Java t-shirts for $24.95 each, but discounts are possible for quantities as follows: 1 or 2 shirts, no discount and total shipping is $10.003-5 shirts, discount is 10% and total shipping is $8.006-10 shirts, discount is 20% and total shipping is $5.0011 or more shirts, discount is 30% and shipping is free Write a Java program that prompts the user for the number of shirts required. The program should then print the extended price of the shirts, the shipping charges, and the total cost of the…arrow_forwardcan you please write it in java.util.scanner form Write a program that prompts the user to enter a number within the range of 1 through 10. The program should display the Roman numeral version of that number. If the number is outside the range of 1 through 10, the program should display an error message. but use switch statement for the program.arrow_forward
- In java: ( no stringbuild or chart.append) the program must produce a neatly labeled bar chart depicting the relative values to each other. Note that there is no interactive input and no use of command line arguments. The program at this stage uses values programmed directly into it. The program output must include the original values as well as the bars. The example below shows the relative number of performances of some Broadway musicals (in picture) You choose the width of the chart. In the example above, it's 60, but it can be different. The largest value in your set should use the entire width, the rest of the bars will be proportionately shorter. Since you're assigning the values in the program, you know the maximum and no special logic is required to find it You must compute and display the "scale" of the chart (i.e. how many units one bar symbol represents) Each bar is labeled with the entity and value You may choose any symbol for your bars 3. In…arrow_forwardPlease help me with this question below using javaarrow_forwardit was an exam questions from last semester. Can you please fill up the tables on 2nd screenshot along side state diagram and its boolean expression as mentioned in the questions?arrow_forward
- While Loop (PLEASE COMPLETE IN JAVA)Instructions Write a program that finds out the perimeter of a multi-sided shape. Perimeter of a multi-sided shape can be found by adding all of the sides together.The user should enter each number at the command prompt. The user will indicate that he or she isfinished entering the number by entering the number -1.Example:This program will sum a series of numbers.Enter the next number (enter -1 when finished)> 2Enter the next number (enter -1 when finished)> 2Enter the next number (enter -1 when finished)> 1Enter the next number (enter -1 when finished)> 1Enter the next number (enter -1 when finished)> -1The sum of your numbers is 6arrow_forwardPython please: If the score is between 90 and 100, the grade is A, and the teacher comment is 'Exceptional work' for 100 only 'Excellent work' for anything other than 100 If the score is between 80 and 89, the grade is B, and the teacher comment is 'Very Good, can do better' If the score is between 70 and 79, the grade is C, and the teacher comment is 'Good, but need to work harder' If the score is between 60 and 69, the grade is D, and there are no teacher comments. If the score is below 60, the grade is F, and the teacher comment is 'Fail, need to appear for a retest' For example if the input (student test score) is 100 the output is The Student grade is A and the teacher comment is 'Exceptional work'arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
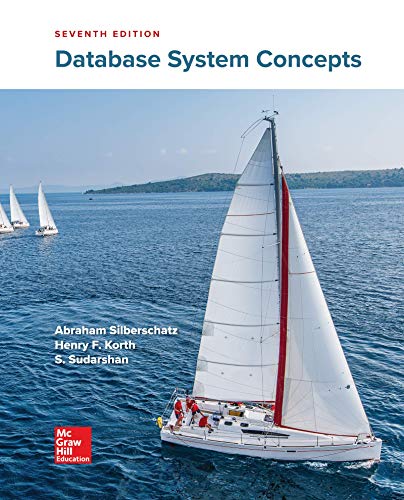
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
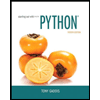
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
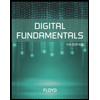
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
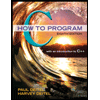
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
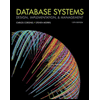
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
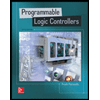
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education