Benford’s Law involves looking at the first digit of a series of numbers. For example, suppose that you were to use a random number generator to generate integers in the range of 100 to 999 and you looked at how often the number begins with 1, how often it begins with 2, and so on. Any decent random number generator would spread the answers out evenly among the 9 regions, so we’d expect to see each digit about one-ninth of the time (11.1%). But with a lot of real-world data, we see a very different distribution. The expected distribution under Benford’s Law is shown below: 1: 30.1%, 2: 17.6%, 3: 12.5%, 4: 9.7%, 5: 7.9%, 6: 6.7%, 7: 5.8%, 8: 5.1%, 9: 4.6% You should use this distribution in your program. You are being provided with a text file giving population data for 247 countries and territories. Your program should read in the data in this file and extract the information needed to keep count of how often each first digit is found. It may be useful to know that the ASCII value for the character ‘1’ is 49. For this assignment, you are to design a Java class named Benford that uses an ArrayList object to store counts of first digits. A Benford object will have only one field, the ArrayList object. You will need the following class methods (at a minimum – you may add as many helper methods as you wish): • A constructor to construct Benford objects • A class method named readCounts() that takes a file name as a parameter and that reads and stores data from a text file into a Benford object. • A class method named benfordPercents() that uses the data in the Benford object to fill an array of double values giving the percentage counts for each initial digit based on the raw counts contained in the Benford object. You are also to design a client class named BenfordPlot that displays the data in the Benford object graphically. The output should be similar to the examples shown below, except your plot should be titled “Population of Countries.” The “^” symbol in the plot below indicated the expected Benford value. The second plot shown below displays a distribution that does NOT follow Benford’s Law Please include the following files: • Benford.java (your Benford class code) • BenfordPlot.java (your client code that using the DrawingPanel code to produce a graphical display of the data contained in a Benford object). • In a comment at the top of your client code (after your name, etc.), include your opinion as to whether or not the data follow Benford’s Law. Design and implementation guidelines: • Javadoc comment all class files and methods • Use validation and generate and handle exceptions as appropriate • Try to write well-structured code - use methods to eliminate redundancy and break large methods into smaller, logical subproblems
What is Benford’s Law?
Benford’s Law involves looking at the first digit of a series of numbers. For example, suppose that you were to use a random number generator to generate integers in the range of 100 to 999 and you looked at how often the number begins with 1, how often it begins with 2, and so on. Any decent random number generator would spread the answers out evenly among the 9 regions, so we’d expect to see each digit about one-ninth of the time (11.1%). But with a lot of real-world data, we see a very different distribution.
The expected distribution under Benford’s Law is shown below:
1: 30.1%, 2: 17.6%, 3: 12.5%, 4: 9.7%, 5: 7.9%, 6: 6.7%, 7: 5.8%, 8: 5.1%, 9: 4.6%
You should use this distribution in your program. You are being provided with a text file giving population data for 247 countries and territories. Your program should read in the data in this file and extract the information needed to keep count of how often each first digit is found. It may be useful to know that the ASCII value for the character ‘1’ is 49.
For this assignment, you are to design a Java class named Benford that uses an ArrayList object to store counts of first digits. A Benford object will have only one field, the ArrayList object.
You will need the following class methods (at a minimum – you may add as many helper methods as you wish):
• A constructor to construct Benford objects
• A class method named readCounts() that takes a file name as a parameter and that reads
and stores data from a text file into a Benford object.
• A class method named benfordPercents() that uses the data in the Benford object to fill an array of double values giving the percentage counts for each initial digit based on the raw counts contained in the Benford object.
You are also to design a client class named BenfordPlot that displays the data in the Benford object graphically. The output should be similar to the examples shown below, except your plot should be titled “Population of Countries.” The “^” symbol in the plot below indicated the
expected Benford value. The second plot shown below displays a distribution that does NOT follow Benford’s Law
Please include the following files:
• Benford.java (your Benford class code)
• BenfordPlot.java (your client code that using the DrawingPanel code to produce a graphical display of the data contained in a Benford object).
• In a comment at the top of your client code (after your name, etc.), include your opinion as to whether or not the data follow Benford’s Law.
Design and implementation guidelines:
• Javadoc comment all class files and methods
• Use validation and generate and handle exceptions as appropriate
• Try to write well-structured code - use methods to eliminate redundancy and break large methods into smaller, logical subproblems

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

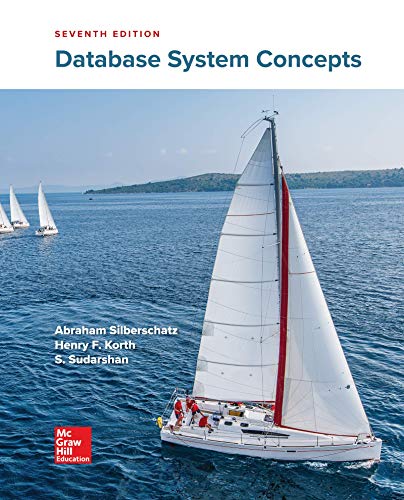
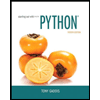
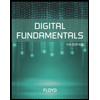
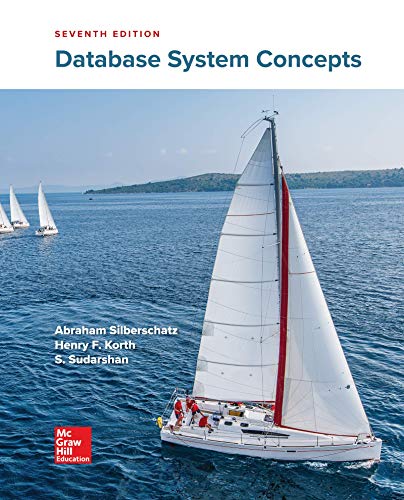
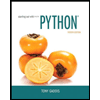
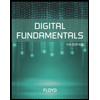
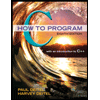
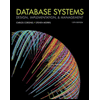
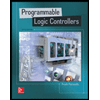