what is the output of this windows form application? using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using MySql.Data.MySqlClient; namespace Windows_form_application_quiz_2 { public partial class Form1 : Form { public Form1() { InitializeComponent(); dg(); } public void dg() { try { MySqlConnection con = new MySqlConnection("datasource=localhost;database=cpedbase;username=root;password="); MySqlDataAdapter adapter = new MySqlDataAdapter("SELECT * FROM studenttbl", con); con.Open(); DataSet ds = new DataSet(); adapter.Fill(ds); dataGridView1.DataSource = ds.Tables[0]; con.Close(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void button1_Click(object sender, EventArgs e) { MySqlConnection con = new MySqlConnection("datasource=localhost;database=cpedbase;username=root;password="); try { MySqlDataAdapter adapter2 = new MySqlDataAdapter("SELECT * FROM studenttbl WHERE StudentID = '" + TBStudID.Text + "'", con); DataSet ds2 = new DataSet(); adapter2.Fill(ds2); var i = ds2.Tables[0].Rows.Count; if (TBStudID.Text == string.Empty || TBLastName.Text == string.Empty || TBFirstName.Text == string.Empty || TBMiddleName.Text == string.Empty || TBCourse.Text == string.Empty || TBYear.Text == string.Empty || TBSchool.Text == string.Empty || TBEmail.Text == string.Empty || TBAddress.Text == string.Empty || TBContact.Text == string.Empty) { MessageBox.Show("Fill up every entry", "Invalid Entry", MessageBoxButtons.OK, MessageBoxIcon.Warning); } else if (i > 0) { MessageBox.Show("Student ID: " +TBStudID.Text+ " already exists", "Duplicate Entry", MessageBoxButtons.OK, MessageBoxIcon.Warning); TBStudID.Text = default; } else { MySqlCommand cmd = new MySqlCommand("INSERT INTO studenttbl VALUES(@StudentID,@LastName,@FirstName,@MiddleName,@Course,@YearLevel,@School,@EmailAddress,@Address,@ContactNumber)", con); cmd.CommandType = CommandType.Text; cmd.Parameters.AddWithValue("@StudentID", TBStudID.Text); cmd.Parameters.AddWithValue("@LastName", TBLastName.Text); cmd.Parameters.AddWithValue("@FirstName", TBFirstName.Text); cmd.Parameters.AddWithValue("@MiddleName", TBMiddleName.Text); cmd.Parameters.AddWithValue("@Course", TBCourse.Text); cmd.Parameters.AddWithValue("@YearLevel", TBYear.Text); cmd.Parameters.AddWithValue("@School", TBSchool.Text); cmd.Parameters.AddWithValue("@EmailAddress", TBEmail.Text); cmd.Parameters.AddWithValue("@Address", TBAddress.Text); cmd.Parameters.AddWithValue("@ContactNumber", TBContact.Text); con.Open(); cmd.ExecuteNonQuery(); con.Close(); MessageBox.Show("Entry Added", "Successful Entry", MessageBoxButtons.OK, MessageBoxIcon.Information); TBStudID.Text = default; TBLastName.Text = default; TBFirstName.Text = default; TBMiddleName.Text = default; TBCourse.Text = default; TBYear.Text = default; TBSchool.Text = default; TBEmail.Text = default; TBAddress.Text = default; TBContact.Text = default; dg(); } } catch(Exception ex) { MessageBox.Show(ex.Message); } } private void TBStudID_KeyPress(object sender, KeyPressEventArgs e) { if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar)) { e.Handled = true; MessageBox.Show("Input must be numeric only","Invalid Entry",MessageBoxButtons.OK, MessageBoxIcon.Warning); } } private void TBContact_KeyPress(object sender, KeyPressEventArgs e) { if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar)) { e.Handled = true; MessageBox.Show("Input must be numeric only", "Invalid Entry", MessageBoxButtons.OK, MessageBoxIcon.Warning); } } } }
what is the output of this windows form application?
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using MySql.Data.MySqlClient;
namespace Windows_form_application_quiz_2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
dg();
}
public void dg()
{
try
{
MySqlConnection con = new MySqlConnection("datasource=localhost;
MySqlDataAdapter adapter = new MySqlDataAdapter("SELECT * FROM studenttbl", con);
con.Open();
DataSet ds = new DataSet();
adapter.Fill(ds);
dataGridView1.DataSource = ds.Tables[0];
con.Close();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void button1_Click(object sender, EventArgs e)
{
MySqlConnection con = new MySqlConnection("datasource=localhost;database=cpedbase;username=root;password=");
try
{
MySqlDataAdapter adapter2 = new MySqlDataAdapter("SELECT * FROM studenttbl WHERE StudentID = '" + TBStudID.Text + "'", con);
DataSet ds2 = new DataSet();
adapter2.Fill(ds2);
var i = ds2.Tables[0].Rows.Count;
if (TBStudID.Text == string.Empty || TBLastName.Text == string.Empty || TBFirstName.Text == string.Empty || TBMiddleName.Text == string.Empty || TBCourse.Text == string.Empty || TBYear.Text == string.Empty || TBSchool.Text == string.Empty || TBEmail.Text == string.Empty || TBAddress.Text == string.Empty || TBContact.Text == string.Empty)
{
MessageBox.Show("Fill up every entry", "Invalid Entry", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
else if (i > 0)
{
MessageBox.Show("Student ID: " +TBStudID.Text+ " already exists", "Duplicate Entry", MessageBoxButtons.OK, MessageBoxIcon.Warning);
TBStudID.Text = default;
}
else
{
MySqlCommand cmd = new MySqlCommand("INSERT INTO studenttbl VALUES(@StudentID,@LastName,@FirstName,@MiddleName,@Course,@YearLevel,@School,@EmailAddress,@Address,@ContactNumber)", con);
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@StudentID", TBStudID.Text);
cmd.Parameters.AddWithValue("@LastName", TBLastName.Text);
cmd.Parameters.AddWithValue("@FirstName", TBFirstName.Text);
cmd.Parameters.AddWithValue("@MiddleName", TBMiddleName.Text);
cmd.Parameters.AddWithValue("@Course", TBCourse.Text);
cmd.Parameters.AddWithValue("@YearLevel", TBYear.Text);
cmd.Parameters.AddWithValue("@School", TBSchool.Text);
cmd.Parameters.AddWithValue("@EmailAddress", TBEmail.Text);
cmd.Parameters.AddWithValue("@Address", TBAddress.Text);
cmd.Parameters.AddWithValue("@ContactNumber", TBContact.Text);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
MessageBox.Show("Entry Added", "Successful Entry", MessageBoxButtons.OK, MessageBoxIcon.Information);
TBStudID.Text = default;
TBLastName.Text = default;
TBFirstName.Text = default;
TBMiddleName.Text = default;
TBCourse.Text = default;
TBYear.Text = default;
TBSchool.Text = default;
TBEmail.Text = default;
TBAddress.Text = default;
TBContact.Text = default;
dg();
}
}
catch(Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void TBStudID_KeyPress(object sender, KeyPressEventArgs e)
{
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar))
{
e.Handled = true;
MessageBox.Show("Input must be numeric only","Invalid Entry",MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
}
private void TBContact_KeyPress(object sender, KeyPressEventArgs e)
{
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar))
{
e.Handled = true;
MessageBox.Show("Input must be numeric only", "Invalid Entry", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
}
}
}

Step by step
Solved in 2 steps

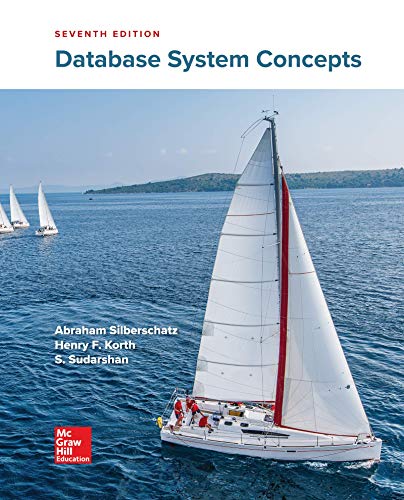
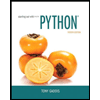
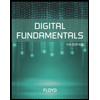
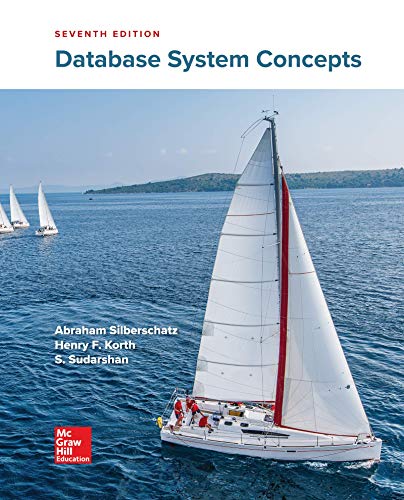
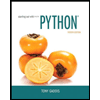
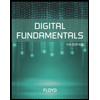
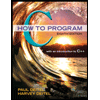
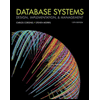
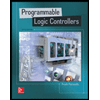