What is the value of finalAmount after executing the following code? ( Assume each class is defined in a separate java file). public class CashRegister {
What is the value of finalAmount after executing the following code? ( Assume each class is defined in a separate java file). public class CashRegister {
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
java
![### Question 7
**Problem Statement:**
What is the value of `finalAmount` after executing the following code? (Assume each class is defined in a separate java file).
**Code Explanation:**
```java
public class CashRegister {
private String cashierName;
private double initialBalance;
private String transactionNum;
public void setInitialBalance(double inBalance) {
initialBalance = inBalance;
}
public double getInitialBalance() {
return initialBalance;
}
public void addBonus(double bonusPer, double amount) {
initialBalance += initialBalance * bonusPer + amount;
}
}
```
The `CashRegister` class contains:
- Three private instance variables: `cashierName`, `initialBalance`, and `transactionNum`.
- A public method `setInitialBalance(double inBalance)` to set the initial balance.
- A public method `getInitialBalance()` to retrieve the initial balance.
- A public method `addBonus(double bonusPer, double amount)` to add a bonus to the `initialBalance` based on a percentage (`bonusPer`) and an additional fixed amount (`amount`).
```java
public class Store {
public static void main(String[] args) {
CashRegister cr = new CashRegister();
cr.setInitialBalance(100);
cr.addBonus(0.2, 10);
double finalAmount = cr.getInitialBalance();
}
}
```
The `Store` class contains the `main` method that:
1. Creates an instance of `CashRegister` named `cr`.
2. Sets the initial balance of `cr` to 100 using `setInitialBalance`.
3. Adds a bonus with `bonusPer` of 0.2 and an additional amount of 10 using `addBonus`.
4. Retrieves the final balance using `getInitialBalance` and stores it in `finalAmount`.
**Calculation of `finalAmount` step-by-step:**
1. **Initial balance** is set to 100.
2. **Bonus calculation** in `addBonus` method:
- `initialBalance * bonusPer = 100 * 0.2 = 20`
- `initialBalance += 20 + 10`
- `initialBalance = 100 + 20 + 10 = 130`
Therefore, the value of `finalAmount` after executing the code is:
**Final Amount: 130.0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F63a2ecd4-d40e-4f58-bfeb-302941b5ae7f%2F4f353c02-87f1-418b-bc19-fb9771bd8f96%2Fdn9lv3_processed.png&w=3840&q=75)
Transcribed Image Text:### Question 7
**Problem Statement:**
What is the value of `finalAmount` after executing the following code? (Assume each class is defined in a separate java file).
**Code Explanation:**
```java
public class CashRegister {
private String cashierName;
private double initialBalance;
private String transactionNum;
public void setInitialBalance(double inBalance) {
initialBalance = inBalance;
}
public double getInitialBalance() {
return initialBalance;
}
public void addBonus(double bonusPer, double amount) {
initialBalance += initialBalance * bonusPer + amount;
}
}
```
The `CashRegister` class contains:
- Three private instance variables: `cashierName`, `initialBalance`, and `transactionNum`.
- A public method `setInitialBalance(double inBalance)` to set the initial balance.
- A public method `getInitialBalance()` to retrieve the initial balance.
- A public method `addBonus(double bonusPer, double amount)` to add a bonus to the `initialBalance` based on a percentage (`bonusPer`) and an additional fixed amount (`amount`).
```java
public class Store {
public static void main(String[] args) {
CashRegister cr = new CashRegister();
cr.setInitialBalance(100);
cr.addBonus(0.2, 10);
double finalAmount = cr.getInitialBalance();
}
}
```
The `Store` class contains the `main` method that:
1. Creates an instance of `CashRegister` named `cr`.
2. Sets the initial balance of `cr` to 100 using `setInitialBalance`.
3. Adds a bonus with `bonusPer` of 0.2 and an additional amount of 10 using `addBonus`.
4. Retrieves the final balance using `getInitialBalance` and stores it in `finalAmount`.
**Calculation of `finalAmount` step-by-step:**
1. **Initial balance** is set to 100.
2. **Bonus calculation** in `addBonus` method:
- `initialBalance * bonusPer = 100 * 0.2 = 20`
- `initialBalance += 20 + 10`
- `initialBalance = 100 + 20 + 10 = 130`
Therefore, the value of `finalAmount` after executing the code is:
**Final Amount: 130.0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
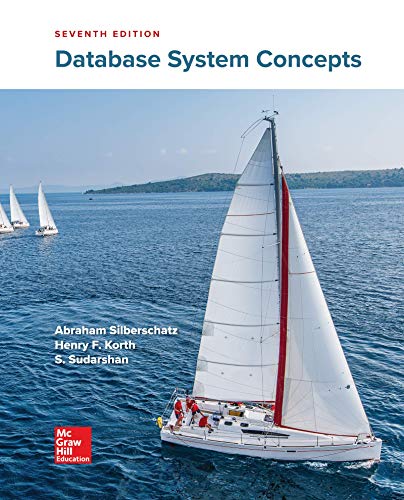
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
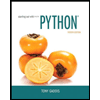
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
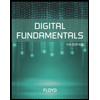
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
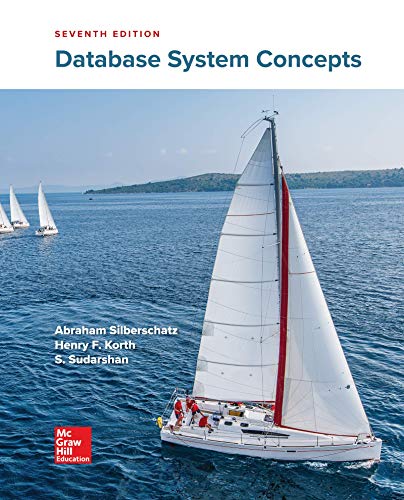
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
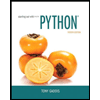
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
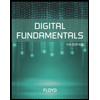
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
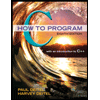
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
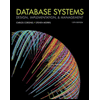
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
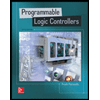
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education