I have been given a project called "Assassin" friom my computer science class, and I cannot seem to be able to create the correct methods in order to run this code properly. I am going to paste my current code for this project. If you would like the instructions, please allow me to either email or create another query, as the instructions are on a word document. Please offer assitance if you can, thank you. My current code: import java.io.*; import java.util.*; /** * Class AssassinMain is the main client program for assassin game management. * It reads names from a file names.txt, shuffles them, and uses them to start * the game. The user is asked for the name of the next victim until the game is * over. */ public class AssassinMain { /** * input file name from which to read data */ public static final String INPUT_FILENAME = "names.txt"; /** * true for different results every run; false for predictable results */ public static final boolean RANDOM = false; /** * If not random, use this value to guide the sequence of numbers that will * be generated by the Random object. */ public static final int SEED = 42; public static void main(String[] args) throws FileNotFoundException { // read names into a Set to eliminate duplicates File inputFile = new File(INPUT_FILENAME); if (!inputFile.canRead()) { System.out.println("Required input file not found; exiting.\n" + inputFile.getAbsolutePath()); System.exit(1); } try (Scanner input = new Scanner(inputFile)) { Set names = new TreeSet<>(String.CASE_INSENSITIVE_ORDER); while (input.hasNextLine()) { String name = input.nextLine().trim().intern(); if (name.length() > 0) { names.add(name); } } // transfer to an ArrayList, shuffle and build an AssassinManager ArrayList nameList = new ArrayList<>(names); Random rand = (RANDOM) ? new Random() : new Random(SEED); Collections.shuffle(nameList, rand); AssassinManager manager = new AssassinManager(nameList); // UNCOMMENT ONCE YOU WRITE THE CONSTRUCTOR // prompt the user for victims until the game is over Scanner console = new Scanner(System.in); while (!manager.isGameOver()) { oneKill(console, manager); } // report who won System.out.println("Game was won by " + manager.winner()); // UNCOMMENT ONCE YOU WRITE winner() System.out.println("Final graveyard is as follows:"); System.out.println(manager.graveyard()); // UNCOMMENT ONCE YOU WRITE graveyard() } } /** * Handles the details of recording one victim. Shows the current kill ring * and graveyard to the user, prompts for a name and records the kill if the * name is legal. */ public static void oneKill(Scanner console, AssassinManager manager) { // print both linked lists System.out.println("Current kill ring:"); System.out.println(manager.killRing()); // UNCOMMENT ONCE YOU WRITE killRing() System.out.println("Current graveyard:"); System.out.println(manager.graveyard()); // UNCOMMENT ONCE YOU WRITE graveyard() // prompt for next victim to kill System.out.println(); System.out.print("next victim? "); String name = console.nextLine().trim(); if (manager.graveyardContains(name)) { // UNCOMMENT ONCE YOU WRITE graveyardContains(name) System.out.println(name + " is already dead."); } else if (!manager.killRingContains(name)) { // UNCOMMENT ONCE YOU WRITE killRingContains(name) System.out.println("Unknown person."); } else { manager.kill(name); // UNCOMMENT ONCE YOU WRITE kill(name) } System.out.println(); } }
I have been given a project called "Assassin" friom my computer science class, and I cannot seem to be able to create the correct methods in order to run this code properly. I am going to paste my current code for this project. If you would like the instructions, please allow me to either email or create another query, as the instructions are on a word document. Please offer assitance if you can, thank you.
My current code:

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

While your code does work, there is the problem of the constraints put on us for this project. I will paste them from the instructions document.
Constraints
• You may not construct any arrays, ArrayLists, LinkedLists, Stacks, Queues, or other data
structures; you must use list nodes. You may not modify the list of Strings passed to your constructor.
• If there are n names in the list of Strings passed to your constructor, you should create exactly n new AssassinNode objects in your constructor. As people are killed, you have to move their node from the kill ring to the graveyard by changing references, without creating any new node objects.
• Your constructor will create the initial kill ring of nodes, and then your class may not create any more nodes for the rest of the program. You are allowed to declare as many local variables of type AssassinNode (like current from lecture) as you like. AssassinNode variables are not node objects and therefore don’t count against the limit of n nodes. You should write some of your own testing code. AssassinMain requires every method to be written in order to compile, and it never generates any of the exceptions you have to handle, so it is not exhaustive.
Would it be possible to adjust the code within these parameters? These limitations are the main issue when trying to create a solution.
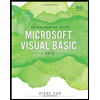
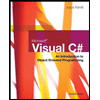
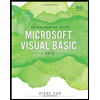
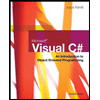