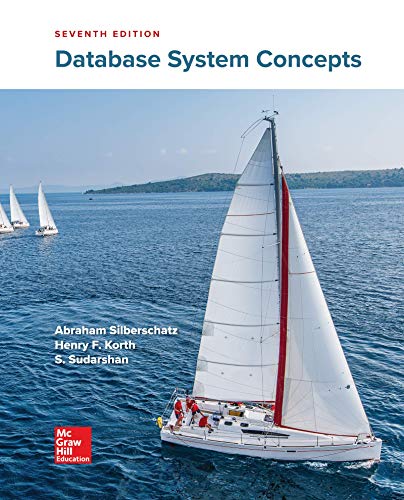
Based on the information given and the class declaration you wrote, write the class implementation (i.e. Cube.cpp) that includes the implementation code of all methods, including the constructor.
#include <string>
#include <cmath>
#include <iostream>
class Cube {
private:
std::string name;
double side;
std::string color;
public:
Cube(std::string name, double side, std::string color);
void setName(std::string newName);
void setSide(double newSide);
void setColor(std::string newColor);
double getVolume();
void volumeIncrease(double percent);
void print();
};
Cube::Cube(std::string name, double side, std::string color) {
this->name = name;
this->side = side;
this->color = color;
}
void Cube::setName(std::string newName) {
this->name = newName;
}
void Cube::setSide(double newSide) {
this->side = newSide;
}
void Cube::setColor(std::string newColor) {
this->color = newColor;
}
double Cube::getVolume() {
return pow(side, 3);
}
void Cube::volumeIncrease(double percent) {
double currentVolume = pow(side, 3);
double newVolume = currentVolume * (1 + percent/100);
double newSide = cbrt(newVolume);
setSide(newSide);
}
void Cube::print() {
std::cout << "Cube Name: " << name << std::endl;
std::cout << "Side Length: " << side << std::endl;
std::cout << "Color: " << color << std::endl;
std::cout << "Volume: " << getVolume() << std::endl;
}
int main() {
Cube cube1("MyCube", 5, "Red");
cube1.print();
cube1.volumeIncrease(2.5);
cube1.print();
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

- 2. Add a constructor for Animal class shown. The constructor should pass in a string parameter named "sound". 1 using System; 2 3 public class Animal 4 { 5 public string Sound { get; set; } public void Speak() { Console.Writeline("The dog says " + Sound); } 10 11 } 12 13 public class Program 14 { public static void Main() { 15 16 17 18 } 19 } A 00arrow_forwardclass A {protected int x1,y1,z; public: A(a, b,c):x1(a+2),y1(b-1),z(c+2) { for(i=0; i<5;i++) x1++;y1++;z++;}}; class B {protected: int x,y; public: B(a,b):x(a+1),y(b+2) { for(i=0; i<5;i++) x+=2; y+=1;}}; class D:public B, virtual public A { private: int a,b; public: D(k,m,n): a(k+n), B(k,m),b(n+2),A(k,m,n) { a=a+1;b=b+1;}}; int main() (Dob(4,2,5);} what the values of x1,y1 and zarrow_forwardJAVA PROGRAM For this program, you are tasked to implement the Beverage class which has the following private properties: name - a string value volume - this is an integer number which represents its current remaining volume in mL isChilled - this is a boolean field which is set to true if the drink is chilled It should have the following methods: isEmpty() - returns true if the volume is already 0 {toString() - returns the details of the object in the following format: {name} ({volume}mL) {"is still chilled" | "is not chilled anymore"}.Example returned strings: Beer (249mL) is still chilled Water (500mL) is not chilled anymore A constructor method with the following signature: public Beverage(name, volume, isChilled) Getter methods for all the 3 properties. Then, create two final subclasses that inherit from this Beverage class. The first one is the Water class which has the additional private property, type, which is a String and can only be either "Purified", "Regular",…arrow_forward
- Java Program This assignment requires one project with two classes. Class Employee Class Employee- I will attach the code for this: //Import the required packages. import java.text.DecimalFormat; import java.text.NumberFormat; //Define the employee class. class Employee { //Define the data members. private String id, lastName, firstName; private int salary; //Create the constructor. public Employee(String id, String lastName, String firstName, int salary) { this.id = id; this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Define the getter methods. public String getId() { return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to…arrow_forwardGiven a class Circle as below: class Circle{ private int radius; double center_x; double center_y; Circle(){ .//some code here } Circle(double x, double y, int radius){ .//some code here } int getRadius(){ return radius; } }; Which of the following line is incorrect? Circle myCircle = new Circle(10,20, 5); I/~~~~~line A System.out.println("radius is: "+myCircle.radius); //--~~~line B Circle yourCircle = new Circle(); //-~~~~line C System.out.println("radius is: "+yourCircle.getRadius()); //---~~line Darrow_forwardJava- Suppose that Vehicle is a class and Car is a new class that extends Vehicle. Write a description of which kind of assignments are permitted between Car and Vehicle variables.arrow_forward
- Java Program This assignment requires one project with two classes. Class Employee Class Employee- I will attach the code for this: //Import the required packages. import java.text.DecimalFormat; import java.text.NumberFormat; //Define the employee class. class Employee { //Define the data members. private String id, lastName, firstName; private int salary; //Create the constructor. public Employee(String id, String lastName, String firstName, int salary) { this.id = id; this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Define the getter methods. public String getId() { return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to…arrow_forwardPlease type the code for the following problems in Pythonarrow_forwardIn this assignment, you will make two classes,Student and Instructor, that inherit from a superclass Person. The implementation of class Person is given below. public class Person{private String name;private int age; public Person(){name="";age=0;}/**Create a person with a given name and age.@param name the name@param age the age*/public Person(String name, int age){this.name = name;this.age = age;} /**Get the name.@return the name*/public String getName(){ return name;}/**Change the name.@param name the name*/public void setName(String name){ this.name = name;}/**Get the age.@return the age*/public int getAge(){ return age;}/**Change the age.@param age the age*/public void setAge(){ this.age = age;}/**Convert person to string form.*/public String toString(){return "Name: " + name + "\t" + "Age: " + age;}} You will also need to write a test program to test the methods you write for these two classes. The implementation details are described as follows. Stage 1:In the first file…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
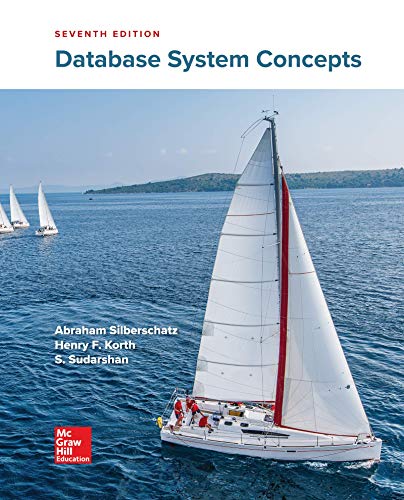
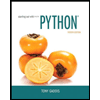
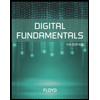
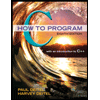
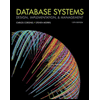
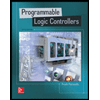