Write a class for the following object. Fraction - an object that represents parts of a whole number. A fraction is created by supplying a numerator and a denominator. Instance related actions 1 add - mutates this fraction by adding the other fraction value 2 subtract - mutates this fraction by subtracting the other fraction value 3. multiply - mutates this fraction by multiplying with the other fraction value 4. divide - mutates this fraction by dividing by the other fraction value 5. tostring - represents the fraction in a form of "numerator/denominator 6. compareto - returns the following values 10 when this fraction is equal with the other fraction 2 1 when this fraction is greater than the other fraction 3. -1 when this fraction is less than the other fraction Example fraction1 = 1/2 fraction2 -3/4 When fraction1addfractiona is called, it will make the value of fraction 1 as S/4. When fraction1suberact(fraction2) is called, it will make the value of fraction 1 as -1/4. When fraction1multiply(fraction2) is caled, it will make the value of fraction 1 as 3/8. When fraction1 divide(fraction2) is called, it will make the value of fraction1a 2/3. When fraction1.compareToffraction2) is called, it will return -1 When fraction2.compareTo(fraction1) is called, it will return 1 Class related actions 1 add - adds 2 fractions resulting to a new fraction 2 subtract - subtracts fraction 2 from fraction 1 resulting to a new fraction 3. multiply - multiplies fraction 1 with fraction 2 resulting to a new fraction 4. divide - divides fraction 1 into fraction 2 pieces S. greaterThen - checks whether fraction 1 is larger than fraction2 6. lessThan - checks whether fraction 1 is smaller than fraction 2 7. equals - checks if both fraction has the same value Example fraction1 - 1/2 fraction2 -3/4 Fraction fractionSum = Fraction addfraction1. fractiona: creates S/4. Fraction fraction Difference = Fraction subtractifraction1 fraction2: creates 1/4. Fraction fractionProduct = Fraction.multiplyfraction1, fraction2: creates 3/8. Fraction fractionQuotient = Fraction.divide(fraction1, fraction2): creates 2/3. System.out.printin(Fraction.greaterThan(fraction1 fraction2): prints false System.out.printin(Fraction.lessThan(fraction1 fraction2l: prints true System.cut printin(Fraction.equals(fraction1 fraction2il: prints false
Write a class for the following object. Fraction - an object that represents parts of a whole number. A fraction is created by supplying a numerator and a denominator. Instance related actions 1 add - mutates this fraction by adding the other fraction value 2 subtract - mutates this fraction by subtracting the other fraction value 3. multiply - mutates this fraction by multiplying with the other fraction value 4. divide - mutates this fraction by dividing by the other fraction value 5. tostring - represents the fraction in a form of "numerator/denominator 6. compareto - returns the following values 10 when this fraction is equal with the other fraction 2 1 when this fraction is greater than the other fraction 3. -1 when this fraction is less than the other fraction Example fraction1 = 1/2 fraction2 -3/4 When fraction1addfractiona is called, it will make the value of fraction 1 as S/4. When fraction1suberact(fraction2) is called, it will make the value of fraction 1 as -1/4. When fraction1multiply(fraction2) is caled, it will make the value of fraction 1 as 3/8. When fraction1 divide(fraction2) is called, it will make the value of fraction1a 2/3. When fraction1.compareToffraction2) is called, it will return -1 When fraction2.compareTo(fraction1) is called, it will return 1 Class related actions 1 add - adds 2 fractions resulting to a new fraction 2 subtract - subtracts fraction 2 from fraction 1 resulting to a new fraction 3. multiply - multiplies fraction 1 with fraction 2 resulting to a new fraction 4. divide - divides fraction 1 into fraction 2 pieces S. greaterThen - checks whether fraction 1 is larger than fraction2 6. lessThan - checks whether fraction 1 is smaller than fraction 2 7. equals - checks if both fraction has the same value Example fraction1 - 1/2 fraction2 -3/4 Fraction fractionSum = Fraction addfraction1. fractiona: creates S/4. Fraction fraction Difference = Fraction subtractifraction1 fraction2: creates 1/4. Fraction fractionProduct = Fraction.multiplyfraction1, fraction2: creates 3/8. Fraction fractionQuotient = Fraction.divide(fraction1, fraction2): creates 2/3. System.out.printin(Fraction.greaterThan(fraction1 fraction2): prints false System.out.printin(Fraction.lessThan(fraction1 fraction2l: prints true System.cut printin(Fraction.equals(fraction1 fraction2il: prints false
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Write a class for the following object.
Fraction - an object that represents parts of a whole number. A fraction is created by supplying a numerator and a denominator.
Instance related actions
1. add - mutates this fraction by adding the other fraction value
2. subtract - mutates this fraction by subtracting the other fraction value
3. multiply - mutates this fraction by multiplying with the other fraction value
4. divide - mutates this fraction by dividing by the other fraction value
5. tostring - represents the fraction in a form of 'numerator/denominator"
6. compareTo - returns the following values
1. O when this fraction is equal with the other fraction
2. 1 when this fraction is greater than the other fraction
3. -1 when this fraction is less than the other fraction
Example
fraction1 = 1/2
fraction2 = 3/4
When fraction1.add(fraction2] is called, it will make the value of fraction 1 as 5/4.
When fraction1.subtract(fraction2) is called, it will make the value of fraction 1 as -1/4.
When fraction1.multiply(fraction2) is called, it will make the value of fraction 1 as 3/8.
When fraction1.divide(fraction2) is called, it will make the value of fraction 1 as 2/3.
When fraction1.compareTo(fraction2) is called, it will return -1.
When fraction 2.compareTo(fraction1) is called, it will return 1.
Class related actions
1. add - adds 2 fractions resulting to a new fraction
2. subtract - subtracts fraction 2 from fraction 1 resulting to a new fraction
3. multiply - multiplies fraction 1 with fraction 2 resulting to a new fraction
4. divide - divides fraction 1 into fraction 2 pieces
5. greaterThen - checks whether fraction 1 is larger than fraction2
6. lessThan - checks whether fraction 1 is smaller than fraction 2
7. equals - checks if both fraction has the same value
Example
fraction1 = 1/2
fraction2 = 3/4
Fraction fractionSum = Fraction.add(fraction1, fraction2): // creates 5/4.
Fraction fractionDifference = Fraction.subtract(fraction1, fraction 2): // creates 1/4.
Fraction fractionProduct = Fraction.multiply(fraction1, fraction2); // creates 3/8.
Fraction fractionQuotient = Fraction.divide(fraction1, fraction2); / creates 2/3.
System.out.printIn(Fraction.greaterThan(fraction1,fraction 2)): / prints false
System.out.printIn(Fraction.lessThan(fraction1,fraction2]): // prints true
System.out.printIn(Fraction.equals(fraction1,fraction2)): / prints false](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9816f2e9-bbbb-45d3-90e7-7a0c9c957c7e%2Fd91645ee-7779-41cb-8193-c523f5eaabdc%2Fwy66hln_processed.png&w=3840&q=75)
Transcribed Image Text:Write a class for the following object.
Fraction - an object that represents parts of a whole number. A fraction is created by supplying a numerator and a denominator.
Instance related actions
1. add - mutates this fraction by adding the other fraction value
2. subtract - mutates this fraction by subtracting the other fraction value
3. multiply - mutates this fraction by multiplying with the other fraction value
4. divide - mutates this fraction by dividing by the other fraction value
5. tostring - represents the fraction in a form of 'numerator/denominator"
6. compareTo - returns the following values
1. O when this fraction is equal with the other fraction
2. 1 when this fraction is greater than the other fraction
3. -1 when this fraction is less than the other fraction
Example
fraction1 = 1/2
fraction2 = 3/4
When fraction1.add(fraction2] is called, it will make the value of fraction 1 as 5/4.
When fraction1.subtract(fraction2) is called, it will make the value of fraction 1 as -1/4.
When fraction1.multiply(fraction2) is called, it will make the value of fraction 1 as 3/8.
When fraction1.divide(fraction2) is called, it will make the value of fraction 1 as 2/3.
When fraction1.compareTo(fraction2) is called, it will return -1.
When fraction 2.compareTo(fraction1) is called, it will return 1.
Class related actions
1. add - adds 2 fractions resulting to a new fraction
2. subtract - subtracts fraction 2 from fraction 1 resulting to a new fraction
3. multiply - multiplies fraction 1 with fraction 2 resulting to a new fraction
4. divide - divides fraction 1 into fraction 2 pieces
5. greaterThen - checks whether fraction 1 is larger than fraction2
6. lessThan - checks whether fraction 1 is smaller than fraction 2
7. equals - checks if both fraction has the same value
Example
fraction1 = 1/2
fraction2 = 3/4
Fraction fractionSum = Fraction.add(fraction1, fraction2): // creates 5/4.
Fraction fractionDifference = Fraction.subtract(fraction1, fraction 2): // creates 1/4.
Fraction fractionProduct = Fraction.multiply(fraction1, fraction2); // creates 3/8.
Fraction fractionQuotient = Fraction.divide(fraction1, fraction2); / creates 2/3.
System.out.printIn(Fraction.greaterThan(fraction1,fraction 2)): / prints false
System.out.printIn(Fraction.lessThan(fraction1,fraction2]): // prints true
System.out.printIn(Fraction.equals(fraction1,fraction2)): / prints false
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 9 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
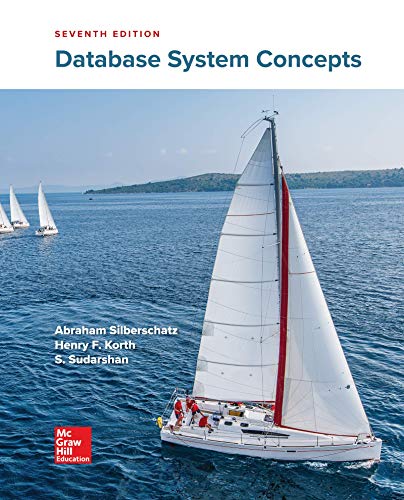
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
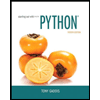
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
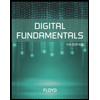
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
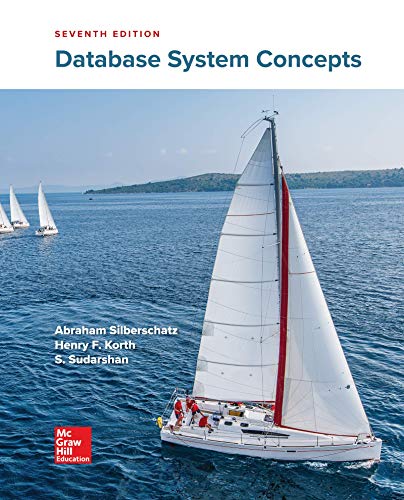
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
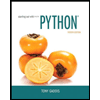
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
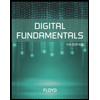
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
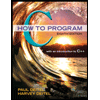
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
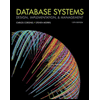
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
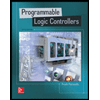
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education