: Write a console-based C# app that uses the concept of inheritance to model motor vehicles as follows: 1. Define an abstract class named MotorVehicles that has the following four abstract methods: a. void driveForward() b. void stop() c. void driveBackward() d. void turn(string direction) 2. This abstract class should: • have the following properties: o make (string) o model (string) o year (int) o weight (float or double) • have a default constructor • have a 4-argument constructor • should override the Equal() method o Two MotorVehicle objects are equal if and only if they have the same make, the same model, the same year, and the same weight • should override the toString() method 3. Derive the following 2 subclasses from the MotorVehicle class: a. A class named Truck. This class should i. have the following property • cargo_bed_size (float or double) ii. a constructor that conforms to the rules of constructors in the context of inheritance iii. a method named printTruck() that just prints “This is a Truck object” iv. overridden methods for the 4 methods in the abstract parent class • The method implementations should just print simple messages that have the word Truck v. should override the Equal() method • Two Truck objects are equal if and only if they have the same properties as in the parent class plus the same cargo_bed_size vi. should override the toString() method Page 4 of 4 b. A class named SportsCar. This class should i. have the following property • zero_to_60_time (float or double) [this is measure of how fast a car accelerates] ii. a constructor that conforms to the rules of constructors in the context of inheritance iii. a method named printSportsCar() that just prints “This is a SportsCar object” iv. overridden methods for the 4 methods in the abstract parent class • The method implementations should just print simple messages that have the word SportsCar. v. should override the Equal() method • Two SportsCar objects are equal if and only if they have the same properties as in the parent class plus the same zero_to_60_time vi. should override the toString() method 4. Define a test class named TestMotorVehicles that has a Main() method. This class should create an array of MotorVehicles. The array size should be 4. Create two objects of type Truck and two objects of type SportsCar and populate the array with these 4 objects. Loop through the array and print the contents of the array on separate lines.
: Write a console-based C# app that uses the concept of inheritance to model motor vehicles as follows: 1. Define an abstract class named MotorVehicles that has the following four abstract methods: a. void driveForward() b. void stop() c. void driveBackward() d. void turn(string direction) 2. This abstract class should: • have the following properties: o make (string) o model (string) o year (int) o weight (float or double) • have a default constructor • have a 4-argument constructor • should override the Equal() method o Two MotorVehicle objects are equal if and only if they have the same make, the same model, the same year, and the same weight • should override the toString() method 3. Derive the following 2 subclasses from the MotorVehicle class: a. A class named Truck. This class should i. have the following property • cargo_bed_size (float or double) ii. a constructor that conforms to the rules of constructors in the context of inheritance iii. a method named printTruck() that just prints “This is a Truck object” iv. overridden methods for the 4 methods in the abstract parent class • The method implementations should just print simple messages that have the word Truck v. should override the Equal() method • Two Truck objects are equal if and only if they have the same properties as in the parent class plus the same cargo_bed_size vi. should override the toString() method Page 4 of 4 b. A class named SportsCar. This class should i. have the following property • zero_to_60_time (float or double) [this is measure of how fast a car accelerates] ii. a constructor that conforms to the rules of constructors in the context of inheritance iii. a method named printSportsCar() that just prints “This is a SportsCar object” iv. overridden methods for the 4 methods in the abstract parent class • The method implementations should just print simple messages that have the word SportsCar. v. should override the Equal() method • Two SportsCar objects are equal if and only if they have the same properties as in the parent class plus the same zero_to_60_time vi. should override the toString() method 4. Define a test class named TestMotorVehicles that has a Main() method. This class should create an array of MotorVehicles. The array size should be 4. Create two objects of type Truck and two objects of type SportsCar and populate the array with these 4 objects. Loop through the array and print the contents of the array on separate lines.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 9E: Write a program named SalespersonDemo that instantiates objects using classes named Real...
Related questions
Question
: Write a console-based C# app that uses the concept of
inheritance to model motor vehicles as follows:
1. Define an abstract class named MotorVehicles that has the following four
abstract methods:
a. void driveForward()
b. void stop()
c. void driveBackward()
d. void turn(string direction)
2. This abstract class should:
• have the following properties:
o make (string)
o model (string)
o year (int)
o weight (float or double)
• have a default constructor
• have a 4-argument constructor
• should override the Equal() method
o Two MotorVehicle objects are equal if and only if they have the
same make, the same model, the same year, and the same
weight
• should override the toString() method
3. Derive the following 2 subclasses from the MotorVehicle class:
a. A class named Truck. This class should
i. have the following property
• cargo_bed_size (float or double)
ii. a constructor that conforms to the rules of constructors in the
context of inheritance
iii. a method named printTruck() that just prints “This is a Truck
object”
iv. overridden methods for the 4 methods in the abstract parent
class
• The method implementations should just print simple
messages that have the word Truck
v. should override the Equal() method
• Two Truck objects are equal if and only if they have the
same properties as in the parent class plus the same
cargo_bed_size
vi. should override the toString() method
Page 4 of 4
b. A class named SportsCar. This class should
i. have the following property
• zero_to_60_time (float or double) [this is measure of
how fast a car accelerates]
ii. a constructor that conforms to the rules of constructors in the
context of inheritance
iii. a method named printSportsCar() that just prints “This is a
SportsCar object”
iv. overridden methods for the 4 methods in the abstract parent
class
• The method implementations should just print simple
messages that have the word SportsCar.
v. should override the Equal() method
• Two SportsCar objects are equal if and only if they have
the same properties as in the parent class plus the same
zero_to_60_time
vi. should override the toString() method
4. Define a test class named TestMotorVehicles that has a Main() method.
This class should create an array of MotorVehicles. The array size should be
4. Create two objects of type Truck and two objects of type SportsCar and
populate the array with these 4 objects.
Loop through the array and print the contents of the array on separate lines.
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
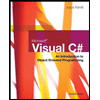
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
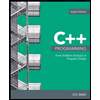
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
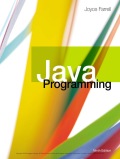
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
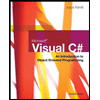
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
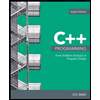
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
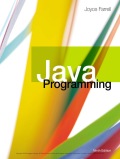
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT