Write a Java program that implements the following classes: Show • name: String - times Watched: int - rate: double - maxRate: int =5 + Show() + Show(name: String) + getName(): String + setName(name:String):void + getRate(): double + getTimesWatched(): int + getMaxRate(): int + updateRate(): void + toString(): String Movie Series - duration: int - production Year: int + Movie() + Movie(name: String, duration: int, production Year:int) + toString(): String + getDuration(): int getProduction Year(): int - numOfEpisodes: int - episodeDuration: int + Series() + Series(name: String, numOfEpisodes:int, episodeDuration: int) + toString():String + getNumOfEpisodes(): int + getEpisode Duration(): int • In the Show class: - The timesWatched and rate must be initialized to zero. - The no-arg constructor reads the name from the user. - The Show(name: String) constructor initializes the data field name with the value of the passed parameter name. - The updateRate method reads the user rate and re-computes the rate data field. Note that: The rate is always computed as the average rates entered by the users. Assume that all users who watched a show must rate it, which means that the number of times a show is watched is the same as the number of times it is rated. If the user does not enter a rate between 0 and the maxGrade, your code should keep on asking the user to enter a rate in the valid range. The updateRate method should also increment the number of times the show is watched (times Watched data field). The toString method must be overridden such that it returns a String that contains the name, rate, and the number of times watched on one line: name - rate - times Watched
Write a Java program that implements the following classes: Show • name: String - times Watched: int - rate: double - maxRate: int =5 + Show() + Show(name: String) + getName(): String + setName(name:String):void + getRate(): double + getTimesWatched(): int + getMaxRate(): int + updateRate(): void + toString(): String Movie Series - duration: int - production Year: int + Movie() + Movie(name: String, duration: int, production Year:int) + toString(): String + getDuration(): int getProduction Year(): int - numOfEpisodes: int - episodeDuration: int + Series() + Series(name: String, numOfEpisodes:int, episodeDuration: int) + toString():String + getNumOfEpisodes(): int + getEpisode Duration(): int • In the Show class: - The timesWatched and rate must be initialized to zero. - The no-arg constructor reads the name from the user. - The Show(name: String) constructor initializes the data field name with the value of the passed parameter name. - The updateRate method reads the user rate and re-computes the rate data field. Note that: The rate is always computed as the average rates entered by the users. Assume that all users who watched a show must rate it, which means that the number of times a show is watched is the same as the number of times it is rated. If the user does not enter a rate between 0 and the maxGrade, your code should keep on asking the user to enter a rate in the valid range. The updateRate method should also increment the number of times the show is watched (times Watched data field). The toString method must be overridden such that it returns a String that contains the name, rate, and the number of times watched on one line: name - rate - times Watched
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
that's a java language
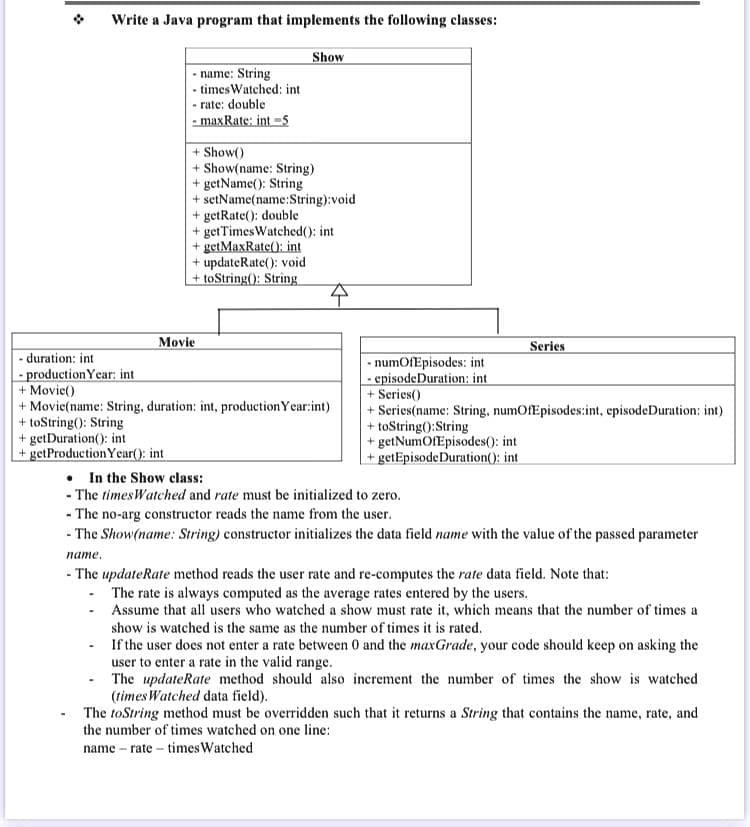
Transcribed Image Text:Write a Java program that implements the following classes:
Show
- name: String
• times Watched: int
• rate: double
- maxRate: int -5
+ Show()
+ Show(name: String)
+ getName(): String
+ setName(name:String):void
+ getRate(): double
+ getTimesWatched(): int
+ getMaxRate(): int
updateRate(): void
+ toString(): String
Movie
Series
- duration: int
- production Year: int
+ Movie()
+ Movie(name: String, duration: int, productionY ear:int)
+ toString(): String
+ getDuration(): int
+ getProduction Year(): int
- numOfEpisodes: int
- episodeDuration: int
+ Series()
+ Series(name: String, numOfEpisodes:int, episodeDuration; int)
+ toString():String
+ getNumOfEpisodes(): int
+ getEpisodeDuration(): int
• In the Show class:
- The timesWatched and rate must be initialized to zero.
- The no-arg constructor reads the name from the user.
- The Show(name: String) constructor initializes the data field name with the value of the passed parameter
пате.
- The updateRate method reads the user rate and re-computes the rate data field. Note that:
- The rate is always computed as the average rates entered by the users.
Assume that all users who watched a show must rate it, which means that the number of times a
show is watched is the same as the number of times it is rated.
If the user does not enter a rate between 0 and the maxGrade, your code should keep on asking the
user to enter a rate in the valid range.
The updateRate method should also increment the number of times the show is watched
(times Watched data field).
The toString method must be overridden such that it returns a String that contains the name, rate, and
the number of times watched on one line:
name – rate - times Watched
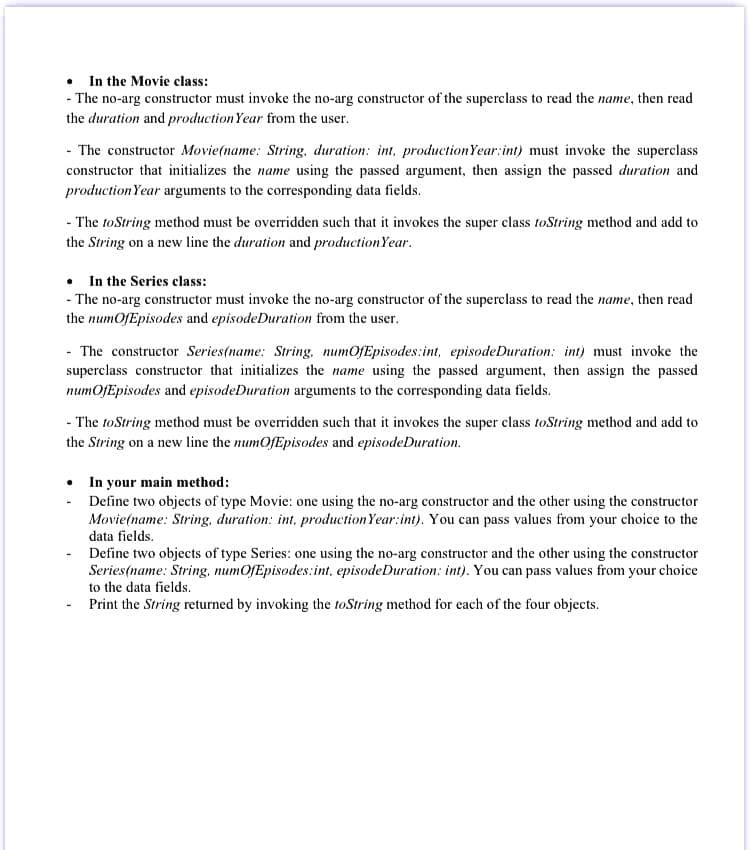
Transcribed Image Text:• In the Movie class:
- The no-arg constructor must invoke the no-arg constructor of the superclass to read the name, then read
the duration and production Year from the user.
- The constructor Movie(name: String, duration: int, productionYear:int) must invoke the superclass
constructor that initializes the name using the passed argument, then assign the passed duration and
production Year arguments to the corresponding data fields.
- The toString method must be overridden such that it invokes the super class toString method and add to
the String on a new line the duration and productionYear.
• In the Series class:
- The no-arg constructor must invoke the no-arg constructor of the superclass to read the name, then read
the numOfEpisodes and episodeDuration from the user.
- The constructor Series(name: String, numOfEpisodes:int, episodeDuration: int) must invoke the
superclass constructor that initializes the name using the passed argument, then assign the passed
numOfEpisodes and episodeDuration arguments to the corresponding data fields.
- The toString method must be overridden such that it invokes the super class toString method and add to
the String on a new line the numOfEpisodes and episodeDuration.
• In your main method:
Define two objects of type Movie: one using the no-arg constructor and the other using the constructor
Movie(name: String, duration: int, productionYear:int). You can pass values from your choice to the
data fields.
- Define two objects of type Series: one using the no-arg constructor and the other using the constructor
Series(name: String, numOfEpisodes:int, episodeDuration: int). You can pass values from your choice
to the data fields.
Print the String returned by invoking the toString method for each of the four objects.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 1 images

Recommended textbooks for you
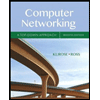
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
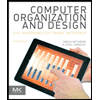
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
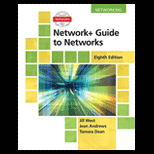
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
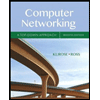
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
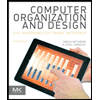
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
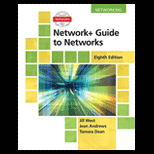
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
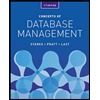
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
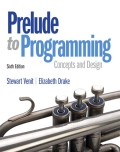
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
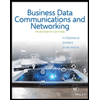
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY