Write a program that: 1. Prompts the user to input the number of buckets of change to calculate. 2. For each bucket, prompts the user to input some amount of monetary change. 3. Uses a new typedef struct and user defined functions to calculate the coins the change represents. 4. Finds the bucket of change with the most coins from the input provided. 5. Outputs the results, as shown below. Your program will need 1 new typedef: • named change, with a struct named change_struct • contains 5 data members (all integers): • quarters, dimes, nickles, pennies o numCoins Your program will need the following 3 functions: GetChange o has a change array and an int parameter uses a for loop to: • prompt the user to enter the amount of change • call ComputeChange to fill the array, passing the user input as an argument • ComputeChange o has one int parameter o calculates the coins the change represents (calculate quarters first, then dimes, then nickels, etc) o returns a change struct to the calling function PrintChange • (output text provided in starter code, just change TBD values) has a change parameter o prints all the data members of the chage struct with the most coins Within your main function you will need to: • prompt the user to enter the number of buckets • create an appropriately sized array of change structs • use GetChange and CalculateChange as described above to prompt for and read data into the array use a for loop to find the bucket with the most coins use PrintChange to print the struct with the most coins, as shown below NOTES: Be sure to include your function prototypes before your main function, and your function definitions after your main function. You DO NOT need to validate input. Sample output (sample input shown with underline): How many buckets: 2 How much change: 25 How much change: 35 Most coins found in bucket 2. Quarters: 1 Dimes: 1 Nickles: 0 Pennies: 0 Total number of coins: 2
Write a program that: 1. Prompts the user to input the number of buckets of change to calculate. 2. For each bucket, prompts the user to input some amount of monetary change. 3. Uses a new typedef struct and user defined functions to calculate the coins the change represents. 4. Finds the bucket of change with the most coins from the input provided. 5. Outputs the results, as shown below. Your program will need 1 new typedef: • named change, with a struct named change_struct • contains 5 data members (all integers): • quarters, dimes, nickles, pennies o numCoins Your program will need the following 3 functions: GetChange o has a change array and an int parameter uses a for loop to: • prompt the user to enter the amount of change • call ComputeChange to fill the array, passing the user input as an argument • ComputeChange o has one int parameter o calculates the coins the change represents (calculate quarters first, then dimes, then nickels, etc) o returns a change struct to the calling function PrintChange • (output text provided in starter code, just change TBD values) has a change parameter o prints all the data members of the chage struct with the most coins Within your main function you will need to: • prompt the user to enter the number of buckets • create an appropriately sized array of change structs • use GetChange and CalculateChange as described above to prompt for and read data into the array use a for loop to find the bucket with the most coins use PrintChange to print the struct with the most coins, as shown below NOTES: Be sure to include your function prototypes before your main function, and your function definitions after your main function. You DO NOT need to validate input. Sample output (sample input shown with underline): How many buckets: 2 How much change: 25 How much change: 35 Most coins found in bucket 2. Quarters: 1 Dimes: 1 Nickles: 0 Pennies: 0 Total number of coins: 2
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 12PE: Write a program that takes as input five numbers and outputs the mean (average) and standard...
Related questions
Question
C
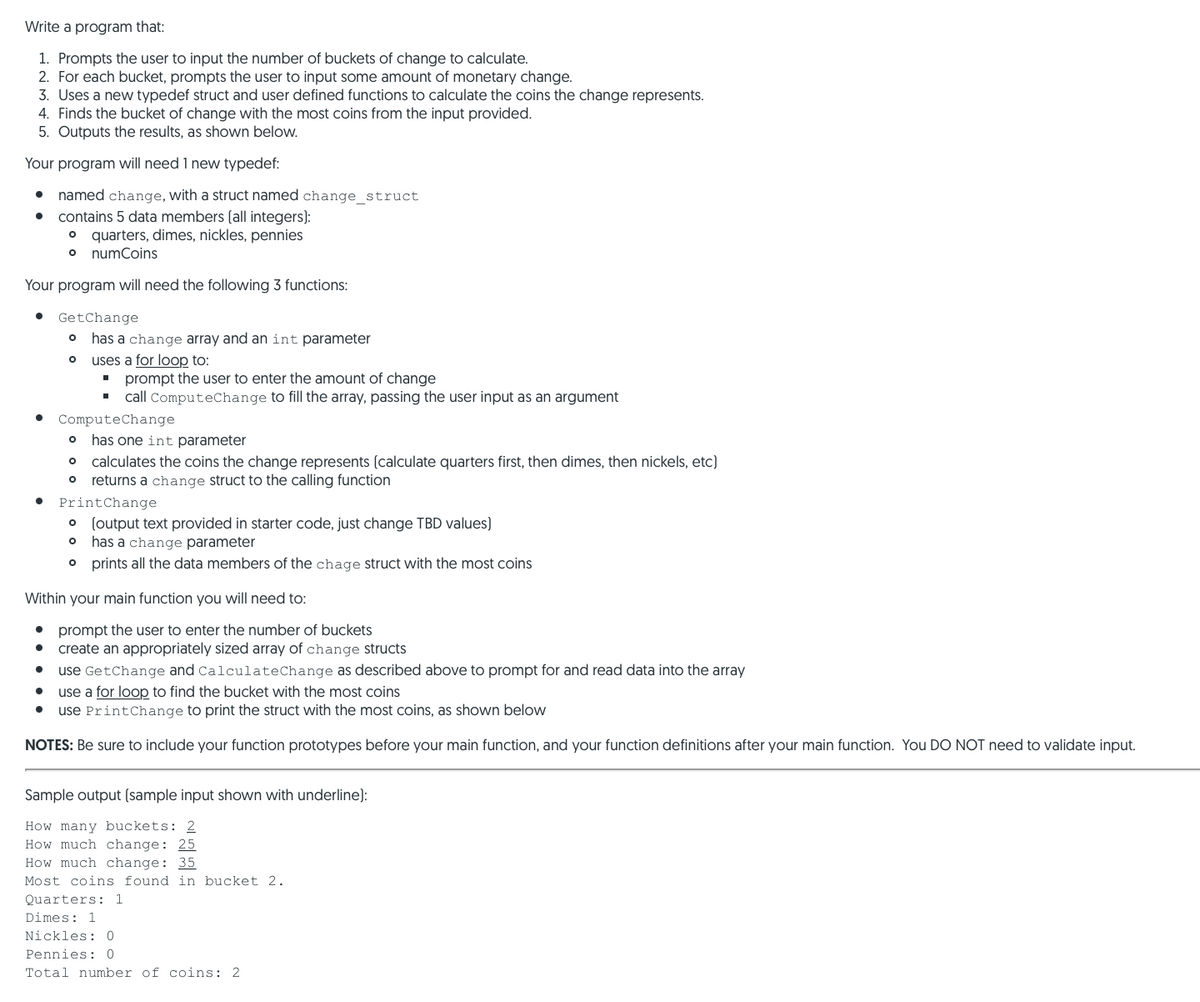
Transcribed Image Text:Write a program that:
1. Prompts the user to input the number of buckets of change to calculate.
2. For each bucket, prompts the user to input some amount of monetary change.
3. Uses a new typedef struct and user defined functions to calculate the coins the change represents.
4. Finds the bucket of change with the most coins from the input provided.
5. Outputs the results, as shown below.
Your program will need 1 new typedef:
named change, with a struct named change_struct
contains 5 data members (all integers):
quarters, dimes, nickles, pennies
numCoins
Your program will need the following 3 functions:
GetChange
o has a change array and an int parameter
uses a for loop to:
prompt the user to enter the amount of change
call ComputeChange to fill the array, passing the user input as an argument
ComputeChange
has one int parameter
calculates the coins the change represents (calculate quarters first, then dimes, then nickels, etc)
returns a change struct to the calling function
PrintChange
o (output text provided in starter code, just change TBD values)
has a change parameter
o prints all the data members of the chage struct with the most coins
Within your main function you will need to:
prompt the user to enter the number of buckets
create an appropriately sized array of change structs
use GetChange and CalculateChange as described above to prompt for and read data into the array
use a for loop to find the bucket with the most coins
use PrintChange to print the struct with the most coins, as shown below
NOTES: Be sure to include your function prototypes before your main function, and your function definitions after your main function. You DO NOT need to validate input.
Sample output (sample input shown with underline):
How many buckets: 2
How much change: 25
How much change: 35
Most coins found in bucket 2.
Quarters: 1
Dimes: 1
Nickles: 0
Pennies: 0
Total number of coins: 2
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
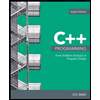
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
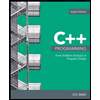
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning