You are writing a program to calculate the average overall scores for two divers in a diving competition. The competition has 5 judges, each from a different country. Each judge will award each diver a score between 0 and 10. Your program will include the following functions • getScore: One double reference parameter. In this function, ask for the name of the country giving the score and the score amount. If the score amount is invalid (i.e. not between 0 and 10), ask again. Return the country name and "return" the score (i.e. use a reference parameter). • findSmallest: Five double parameters. The smallest amount is returned. • findLargest: Five double parameters. The largest amount is returned. • getAverage: Five double parameters. The lowest and highest scores should be dropped, and the remaining three should be averaged. You can determine which three should be averaged by adding all five numbers together, then calling findSmallest and findLargest and subtracting both return results from the total. Then, average the remaining score. Return the result. • scoreDive: This function will return the average overall score for a single diver. It should call getScore five times. Afterwards, print out each country's name and corresponding score. Finally, call getAverage (passing the five scores) and return the result. In main, call the scoreDive function twice, once for diver 1 and once for diver 2. Then, print the final score earned by each.
You are writing a program to calculate the average overall scores for two divers in a diving competition. The competition has 5 judges, each from a different country. Each judge will award each diver a score between 0 and 10. Your program will include the following functions • getScore: One double reference parameter. In this function, ask for the name of the country giving the score and the score amount. If the score amount is invalid (i.e. not between 0 and 10), ask again. Return the country name and "return" the score (i.e. use a reference parameter). • findSmallest: Five double parameters. The smallest amount is returned. • findLargest: Five double parameters. The largest amount is returned. • getAverage: Five double parameters. The lowest and highest scores should be dropped, and the remaining three should be averaged. You can determine which three should be averaged by adding all five numbers together, then calling findSmallest and findLargest and subtracting both return results from the total. Then, average the remaining score. Return the result. • scoreDive: This function will return the average overall score for a single diver. It should call getScore five times. Afterwards, print out each country's name and corresponding score. Finally, call getAverage (passing the five scores) and return the result. In main, call the scoreDive function twice, once for diver 1 and once for diver 2. Then, print the final score earned by each.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.3: Returning Multiple Value
Problem 6E
Related questions
Question
C++ Question
Hello, Please create the correct code for the attached picture. Create the code based on the given requirements. PLEASE DO NOT USE ARRAYS IN THE CODE. There is also a 2nd picture of how the layout of the beginning of the code should look like. Please solve it correctly. Thank you!
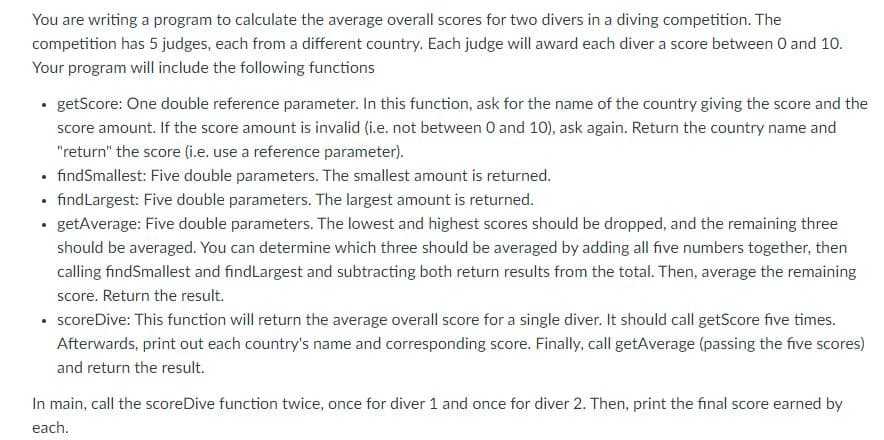
Transcribed Image Text:You are writing a program to calculate the average overall scores for two divers in a diving competition. The
competition has 5 judges, each from a different country. Each judge will award each diver a score between 0 and 10.
Your program will include the following functions
getScore: One double reference parameter. In this function, ask for the name of the country giving the score and the
score amount. If the score amount is invalid (i.e. not between 0 and 10), ask again. Return the country name and
"return" the score (i.e. use a reference parameter).
• findSmallest: Five double parameters. The smallest amount is returned.
• findLargest: Five double parameters. The largest amount is returned.
• getAverage: Five double parameters. The lowest and highest scores should be dropped, and the remaining three
should be averaged. You can determine which three should be averaged by adding all five numbers together, then
calling findSmallest and findLargest and subtracting both return results from the total. Then, average the remaining
score. Return the result.
• scoreDive: This function will return the average overall score for a single diver. It should call getScore five times.
Afterwards, print out each country's name and corresponding score. Finally, call getAverage (passing the five scores)
and return the result.
In main, call the scoreDive function twice, once for diver 1 and once for diver 2. Then, print the final score earned by
each.
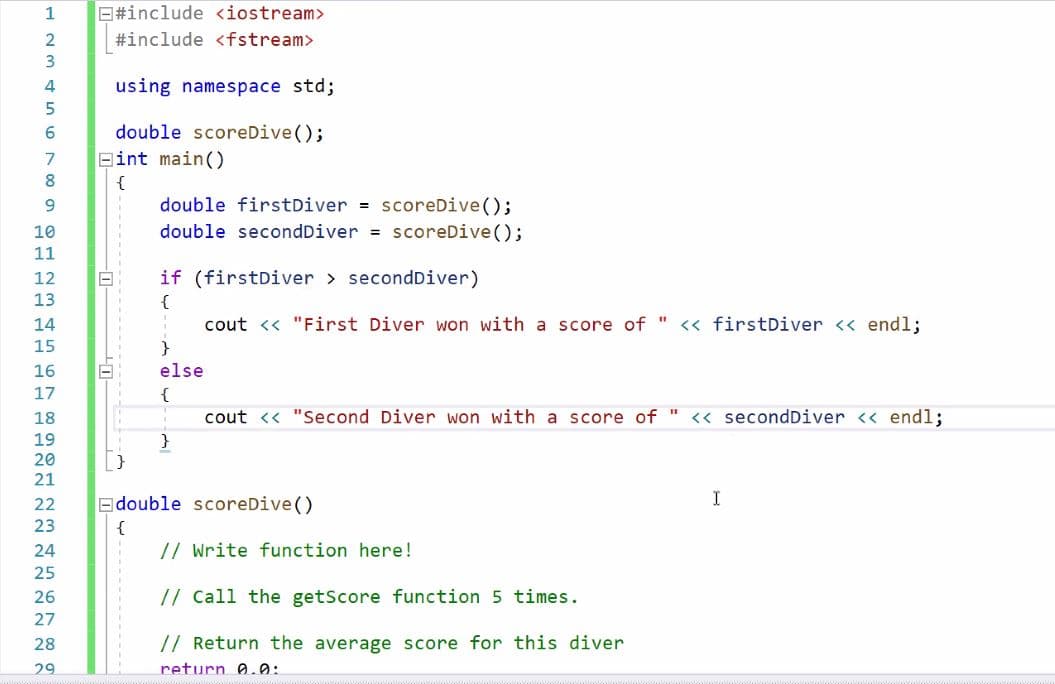
Transcribed Image Text:1
O#include <iostream>
#include <fstream>
using namespace std;
6.
double scoreDive();
7
Bint main()
8.
{
double firstDiver = scoreDive();
double secondDiver = scoreDive();
10
11
12
if (firstDiver > secondDiver)
13
{
cout <« "First Diver won with a score of " << firstDiver <« endl;
%3D
14
15
}
16
else
17
{
18
cout <« "Second Diver won with a score of " << secondDiver << endl;
19
20
21
22
Edouble scoreDive ()
I
23
{
24
// Write function here!
25
26
// Call the getScore function 5 times.
27
28
// Return the average score for this diver
29
return 0.0:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
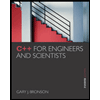
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
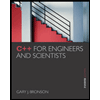
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr