Write a program that accepts the lengths of three sides of a triangle as inputs. The program output should indicate whether or not the triangle is a right triangle. Recall from the Pythagorean theorem that in a right triangle, the square of one side equals the sum of the squares of the other two sides. Use The triangle is a right triangle. and The triangle is not a right triangle. as your final outputs. Make sure to use exponential operator ** to compute the value of each side of the triangle. Any of the three sides of the triangle could be the hypotenuse. The program should handle this appropriately This is what I try to do but it tells me there is an error. a = float(input("Enter the first side: ")) b = float(input("Enter the second side: ")) c = float(input("Enter the third side: ")) if ((a**2 == b + c) or (b**2 == a + c) or (c**2 == a + b)): print ("The triangle is a right triangle.") else: print ("The triangle is not a right triangle.")
Write a program that accepts the lengths of three sides of a triangle as inputs. The program output should indicate whether or not the triangle is a right triangle. Recall from the Pythagorean theorem that in a right triangle, the square of one side equals the sum of the squares of the other two sides. Use The triangle is a right triangle. and The triangle is not a right triangle. as your final outputs. Make sure to use exponential operator ** to compute the value of each side of the triangle. Any of the three sides of the triangle could be the hypotenuse. The program should handle this appropriately This is what I try to do but it tells me there is an error. a = float(input("Enter the first side: ")) b = float(input("Enter the second side: ")) c = float(input("Enter the third side: ")) if ((a**2 == b + c) or (b**2 == a + c) or (c**2 == a + b)): print ("The triangle is a right triangle.") else: print ("The triangle is not a right triangle.")
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 12E: (Program) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
Write a
Recall from the Pythagorean theorem that in a right triangle, the square of one side equals the sum of the squares of the other two sides.
- Use The triangle is a right triangle. and The triangle is not a right triangle. as your final outputs.
Make sure to use exponential operator ** to compute the value of each side of the triangle.
Any of the three sides of the triangle could be the hypotenuse. The program should handle this appropriately
This is what I try to do but it tells me there is an error.
a = float(input("Enter the first side: "))
b = float(input("Enter the second side: "))
c = float(input("Enter the third side: "))
if ((a**2 == b + c) or (b**2 == a + c) or (c**2 == a + b)):
print ("The triangle is a right triangle.")
else:
print ("The triangle is not a right triangle.")
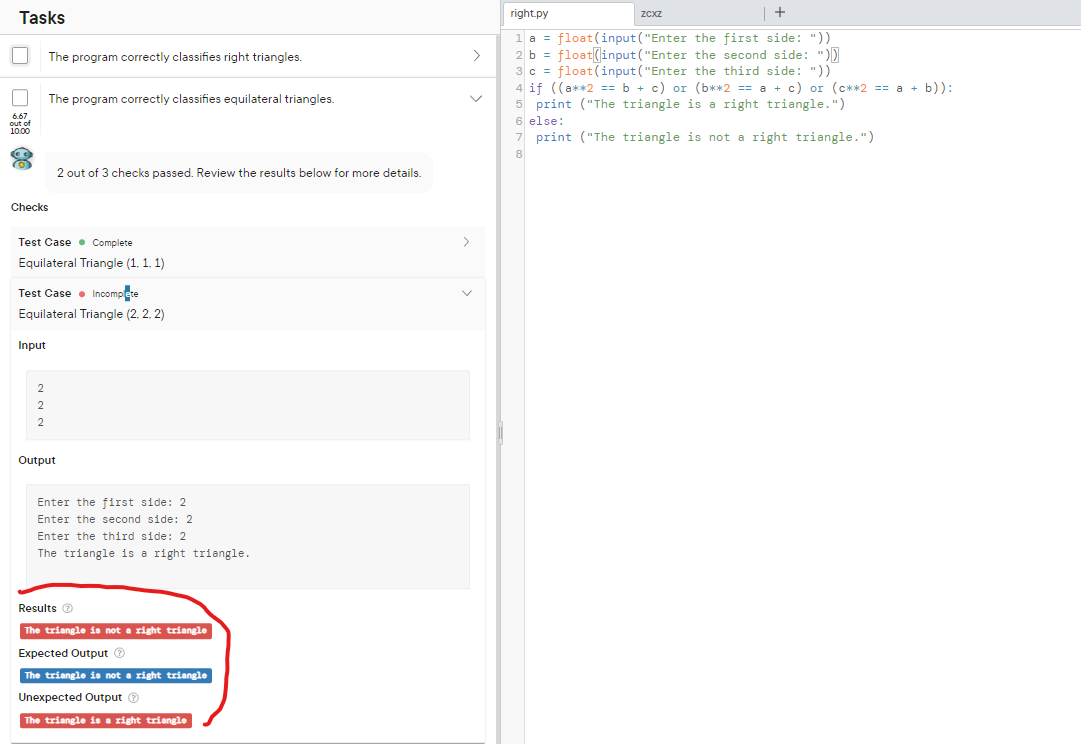
Transcribed Image Text:Tasks
6.67
out of
10.00
@
The program correctly classifies right triangles.
The program correctly classifies equilateral triangles.
Checks
Input
2 out of 3 checks passed. Review the results below for more details.
Test Case
Complete
Equilateral Triangle (1. 1. 1)
Test Case Incomplete
Equilateral Triangle (2. 2. 2)
Output
Enter the first side: 2
Enter the second side: 2
Enter the third side: 2
The triangle is a right triangle.
Results Ⓒ
The triangle is not a right triangle
Expected Output Ⓒ
The triangle is not a right triangle
Unexpected Output →
The triangle is a right triangle
right.py
1a = float(input("Enter the first side: "))
2 b = float(input ("Enter the second side: "))
3 c = float(input("Enter the third side: "))
4 if ((a**2 == b + c) or (b**2 == a + c) or (c**2 == a + b)):
5 print ("The triangle is a right triangle.")
ZCxZ
6 else:
7 print ("The triangle is not a right triangle.")
8
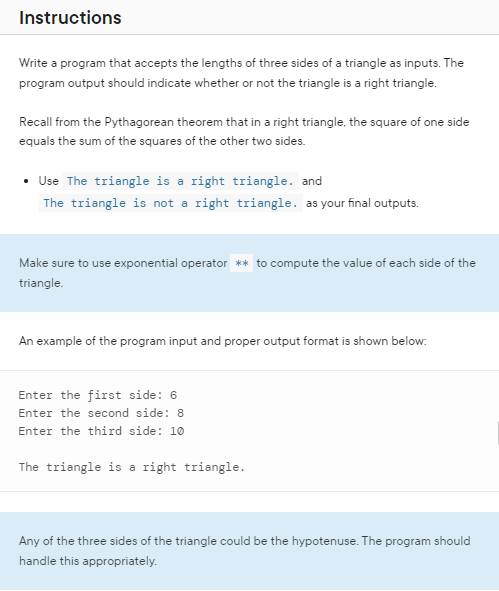
Transcribed Image Text:Instructions
Write a program that accepts the lengths of three sides of a triangle as inputs. The
program output should indicate whether or not the triangle is a right triangle.
Recall from the Pythagorean theorem that in a right triangle, the square of one side
equals the sum of the squares of the other two sides.
• Use The triangle is a right triangle. and
The triangle is not a right triangle. as your final outputs.
Make sure to use exponential operator ** to compute the value of each side of the
triangle.
An example of the program input and proper output format is shown below:
Enter the first side: 6
Enter the second side: 8
Enter the third side: 10
The triangle is a right triangle.
Any of the three sides of the triangle could be the hypotenuse. The program should
handle this appropriately.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
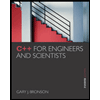
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
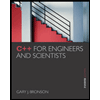
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr