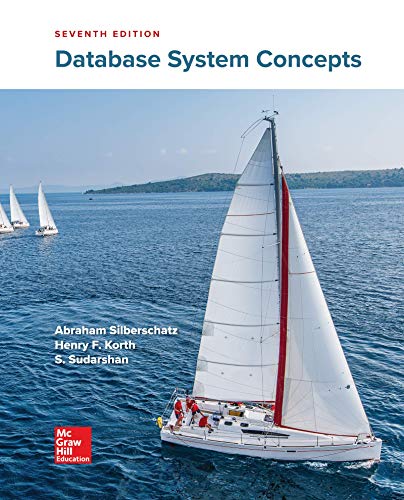
Write a
As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2
Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string.
Ex: If the input is:
6
the output is:
110
Your program must define and call the following two functions. The IntegerToReverseBinary() function should return a string of 1's and 0's representing the integer in binary (in reverse). The ReverseString() function should return a string representing the input string in reverse.
string IntegerToReverseBinary(int integerValue)
string ReverseString(string userString)
#include <iostream>
using namespace std;
/* Define your functions here */
int main() {
/* Type your code here. Your code must call the functions. */
return 0;
}
Please help me with this problem using c++.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Using Pythonarrow_forwardWrite a function number_of_pennies() that returns the total number of pennies given a number of dollars and (optionally) a number of pennies. Ex: If you have $5.06 then the input is 5 6, and if you have $4.00 then the input is 4. Sample output with inputs: 5 64 506 400 Learn how our autograder works 461710.3116374.qx3zqy7 1 2 Your solution goes here '' 3 4 print (number_of_pennies (int(input()), int(input()))) 5 print(number_of_pennies (int(input()))) # Both dollars and pennies # Dollars onlyarrow_forwardThe sigmoid function takes an input x of any real number and returns an output value in the range of -1 and 1. Write a program that takes a real number x as the input (x is of type double) and computes its sigmoid value. The sigmoid can be computed using the equation below. For ez use the function exp(), which is available in #include <cmath>. Expected output 1 (bold is user input) Enter a value for x: 1 The sigmoid for x=1 is 0.731059 Expected output 2 (bold is user input) Enter a value for x: 10 The sigmoid for x=10 is 0.999955 The file should be named as calcSigmoid.cpp. Don’t forget to head over to Coderunner on Canvas and paste your solution in the answer box!arrow_forward
- Define function print popcorn_time() with parameter bag ounces. If bag ounces is less than 3, print "Too small". If greater than 10, print "Too large". Otherwise, compute and print 6* bag ounces followed by 'seconds". End with a newline. Sample output with input: 7 42 seconds 1 2 Your solution goes here *** 3 4 user_ounces int(input()) 5 print popcorn_time(user_ounces) Iarrow_forwardWrite the loop condition and function body to complete the program below. The function should generate random (x,y) coordinates between 1-10, and return a Point object with those coordinates. This program randomly generates locations for two players, repeating until one of their coordinates are the same for both players. /* Here is the Sample Output your completed program should produce: This program randomly generates (x,y) locationsbetween 1-10 for two players, repeating untilone of their coordinates are the same for both players.Player1 location: (8,7)Player2 location: (2,9) Player1 location: (9,9)Player2 location: (4,1) Player1 location: (3,8)Player2 location: (3,8) This program randomly generates (x,y) locationsbetween 1-10 for two players, repeating untilone of their coordinates are the same for both players.Player1 location: (10,3)Player2 location: (3,1) Player1 location: (1,5)Player2 location: (9,2) Player1 location: (4,9)Player2 location: (7,1) Player1…arrow_forwardCan u code it in Pythonarrow_forward
- Write a python program with the following functions:1. the function factorial_loop(num) that takes an integer num and returns the value of the factorial of num written as num!, where num! = num * (num-1) * (num-2) * … * 2 * 1. This function must use a loop (iteration) to compute the value of num!.2. the function factorial_recursive(num) that takes an integer num and returns the value of the factorial of num (i.e., num!) as defined above. This function must use a recursive algorithm to compute the value of num!.arrow_forwardWrite a function that finds and returns the smallest value of n for which: 1 + 2 + 3 + ... + n is greater than or equal to max (where max is a number input from the user). You can use the following prototype: int SmallestValue(int); In the main program be sure to declare all variables and show the call. Be user friendly! Sample run: What is the max number: 23 The smallest value of n where the sum of 1 to n is greater or equal to 23 is: 7 Again? Y What is the max number: 12 The smallest value of n where the sum of 1 to n is greater or equal to 12 is: 5 Again? N in c++ please use basic coding I'm not too advancedarrow_forwardExercise 5 The greatest common divisor (GCD) of a and b is the largest number that divides both of them with no remainder. One way to find the GCD of two numbers is based on the observation that if r is the remainder when a is divided by b, then gcd(a, b) = gcd(b, r). As a base case, we can use gcd(a, 0) = a. Write a function called gcd that takes parameters a and b and returns their greatest common divisor.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
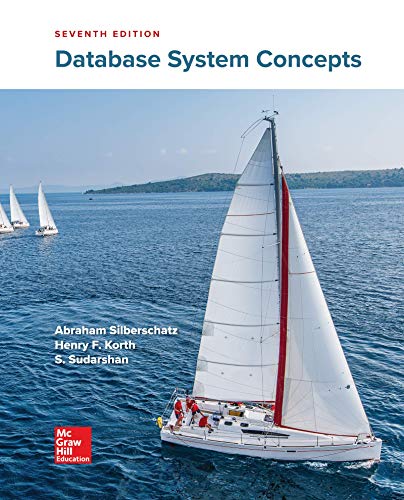
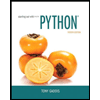
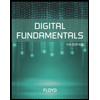
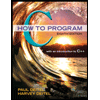
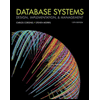
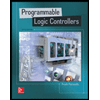