Write a program that allows the user to input the r- and y-coordinates of two squares, and then prints out whether or not they form a valid ship – meaning that both squares lie on the grid, and are adjacent to one another horizontally or vertically. If one of those conditions is not met, the program should print out a message saying which condition is wrong. (If both conditions are not met, the program only needs to output a single message, which can be either one, your choice.) For example, here is a sample run: Enter x-coordinate of square one: 3 Enter y-coordinate of square one: 2 Enter x-coordinate of square two: 3 Enter y-coordinate of square two: 3 Valid - here, the numbers are input by the user, and everything else is produced by the program; this is Valid because it corresponds to the first placement shown in the figure above. Other sample runs might look like: Enter x-coordinate of square one: 3 Enter y-coordinate of square one: -1 Enter x-coordinate of square two: 5 Enter y-coordinate of square two: 2 Square(s) not on grid
Write a program that allows the user to input the r- and y-coordinates of two squares, and then prints out whether or not they form a valid ship – meaning that both squares lie on the grid, and are adjacent to one another horizontally or vertically. If one of those conditions is not met, the program should print out a message saying which condition is wrong. (If both conditions are not met, the program only needs to output a single message, which can be either one, your choice.) For example, here is a sample run: Enter x-coordinate of square one: 3 Enter y-coordinate of square one: 2 Enter x-coordinate of square two: 3 Enter y-coordinate of square two: 3 Valid - here, the numbers are input by the user, and everything else is produced by the program; this is Valid because it corresponds to the first placement shown in the figure above. Other sample runs might look like: Enter x-coordinate of square one: 3 Enter y-coordinate of square one: -1 Enter x-coordinate of square two: 5 Enter y-coordinate of square two: 2 Square(s) not on grid
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 2GZ
Related questions
Question
Simple python
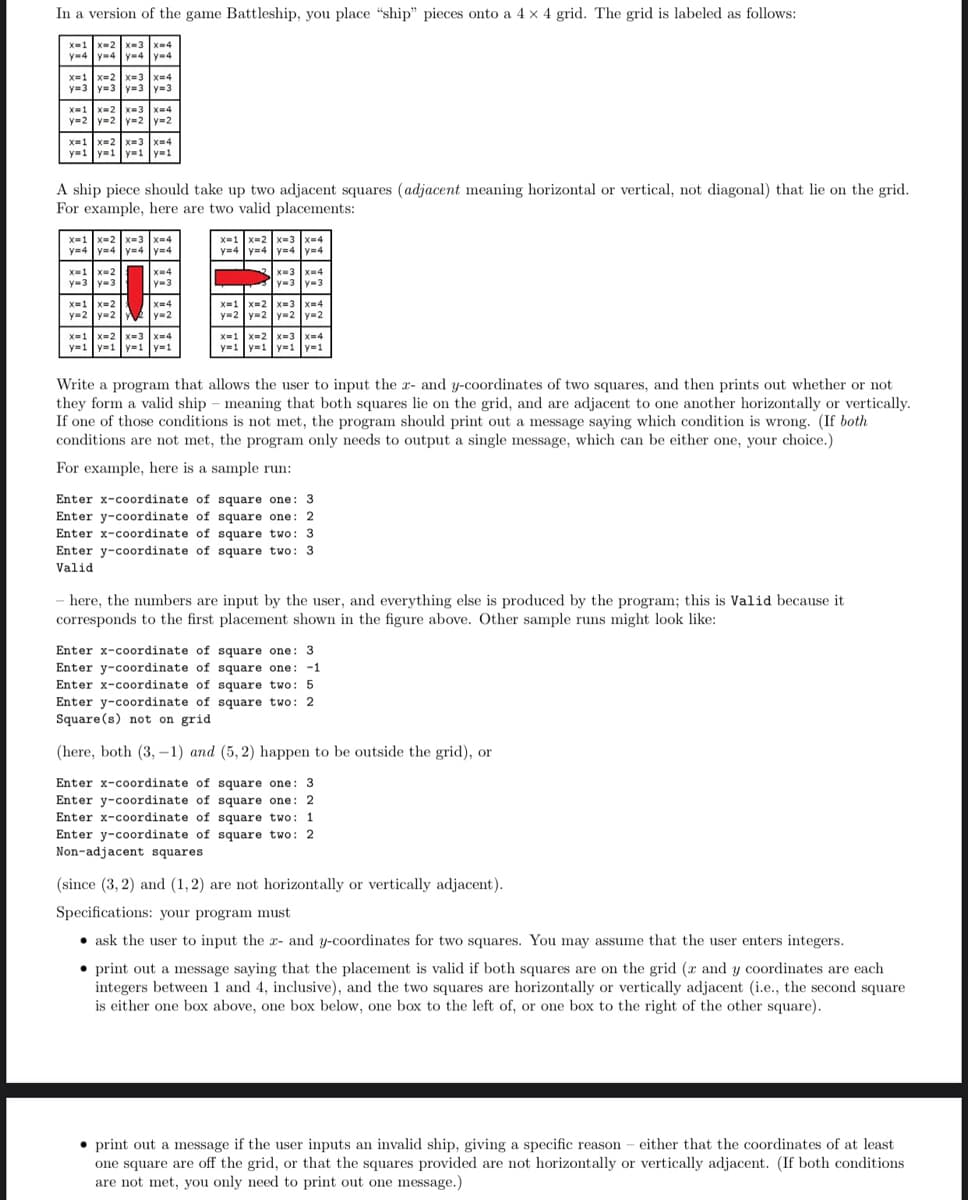
Transcribed Image Text:In a version of the game Battleship, you place "ship" pieces onto a 4 × 4 grid. The grid is labeled as follows:
X=1 x-2 x-3 x-4
y-4 y-4 y-4 y-4
x=1 x=2 x-3 x=4
y=3 y=3 y=3 y=3
x=1 x-2 x-3 x=4
y=2 y=2 y-2 y=2
x=1 x=2 x=3 x=4
y=1 y=1 y=1 y=1
A ship piece should take up two adjacent squares (adjacent meaning horizontal or vertical, not diagonal) that lie on the grid.
For example, here are two valid placements:
x=1 x=2 x=3 x=4
y=4 y=4 y4 ym4
x=1 x-2 x-3 x-4
y=4 y=4 y4 y=4
X-1 x-2 x-4
y=3 y-3
X-3 x-4
y=3 y-3
y-3
X=1 X=2
y=2 y=2
y=2
x=1 x-2 x=3 x-4
y=2 y=2 y-2 y=2
x-3 x=4
x=1 x=2 x=3 x=4
y=1 y=1 y=1 |y=1
X=1 x-2
y=1 y=1 y=1 y=1
Write a program that allows the user to input the r- and y-coordinates of two squares, and then prints out whether or not
they form a valid ship – meaning that both squares lie on the grid, and are adjacent to one another horizontally or vertically.
If one of those conditions is not met, the program should print out a message saying which condition is wrong. (If both
conditions are not met, the program only needs to output a single message, which can be either one, your choice.)
For example, here is a sample run:
Enter x-coordinate of square one: 3
Enter y-coordinate of square one: 2
Enter x-coordinate of square two: 3
Enter y-coordinate of square two: 3
Valid
here, the numbers are input by the user, and everything else is produced by the program; this is Valid because it
corresponds to the first placement shown in the figure above. Other sample runs might look like:
Enter x-coordinate of square one: 3
Enter y-coordinate of square one: -1
Enter x-coordinate of square two: 5
Enter y-coordinate of square two: 2
Square(s) not on grid
(here, both (3, -1) and (5, 2) happen to be outside the grid), or
Enter x-coordinate of square one: 3
Enter y-coordinate of square one: 2
Enter x-coordinate of square two: 1
Enter y-coordinate of square two: 2
Non-adjacent squares
(since (3, 2) and (1,2) are not horizontally or vertically adjacent).
Specifications: your program must
• ask the user to input the x- and y-coordinates for two squares. You may assume that the user enters integers.
• print out a message saying that the placement is valid if both squares are on the grid (r and y coordinates are each
integers between 1 and 4, inclusive), and the two squares are horizontally or vertically adjacent (i.e., the second square
is either one box above, one box below, one box to the left of, or one box to the right of the other square).
• print out a message if the user inputs an invalid ship, giving a specific reason
one square are off the grid, or that the squares provided are not horizontally or vertically adjacent. (If both conditions
are not met, you only need to print out one message.)
either that the coordinates of at least
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
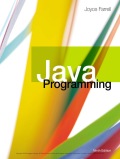
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
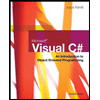
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
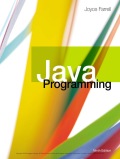
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
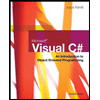
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,