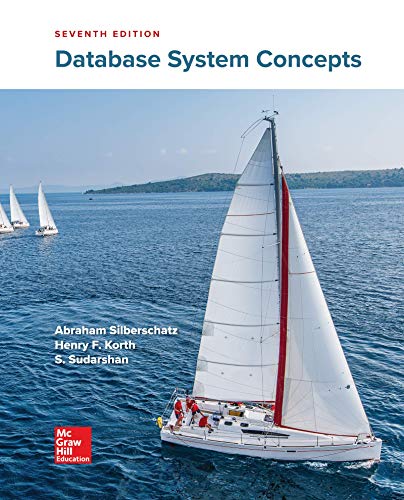
Concept explainers
Write a program that mimics a calculator. The program should take as input two integers and the operation to be performed. It should then output the numbers, the operator, and the result. For division, if the denominator is zero, output an appropriate message. Limit the supported operations to + -/ *and write an error message if the operator is not one of the supported operations. Here is some example output:
3 + 4 = 7
13 * 5 = 65
C++

#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
char op;
int numb1;
int numb2;
cout <<"Please input two values that will be calculated: ";
cin >> numb1 >> numb2;
cout <<"Please input the operator that will be used: ";
cin >> op;
cout << fixed << showpoint << setprecision(2);
switch(op)
{
case '+':
cout << numb1 << " + " << numb2 << " = " << numb1 + numb2;
break;
case '-':
cout << numb1 << " - " << numb2 << " = " << numb1 - numb2;
break;
case '*':
cout << numb1 << " * " << numb2 << " = " << numb1 * numb2;
break;
case '/':
if (numb2 == 0)
cout <<"ERROR! Dividing by 0 isn't allowed!";
else
cout << numb1 << " / " << numb2 << " = " << numb1 / numb2;
break;
default:
cout <<"ERROR! The operator you have chosen is incorrect!";
break;
}
return 0;
}
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 5 images

- Write a program that asks the user to enter 2 integers of 4 digits and displays thedifferent digits as X and * if they are the same. If the user enters a number which is notof four digits, then a convenient message is displayed.arrow_forwardIn the C language please?arrow_forwardGiven three floating-point numbers x, y, and z, output x to the power of z, x to the power of (y to the power of z), the absolute value of (x minus y), and the square root of (x to the power of z). Output each floating-point value with two digits after the decimal point, which can be achieved as follows: {your_value2:.2f} {your_value3:.2f} {your_value4:.2f}') print (f'{your_value1:.2f} Ex: If the input is: 5.0 1.5 3.2 Then the output is: 172.47 361.66 3.50 13.13 461710 3116374.qx3zqy7 LAB ACTIVITY 2.14.1: LAB: Using math functions 1 import math 2 111 Type your code here. main.py 0/10 Load default template...arrow_forward
- Write a program which: 1. prints all multiples of 5 between 5 and 50 inclusive and then 2. reads a number from the user and prints out if it is zero, above zero, or below zeroarrow_forwardGiven three floating-point numbers x, y, and z, output x to the power of z, x to the power of (y to the power of z), the absolute value of (x minus y), and the square root of (x to the power of z). Output each floating-point value with two digits after the decimal point, which can be achieved as follows: {your_value2:.2f} {your_value3:.2f} {your_value4:.2f}') print (f'{your_value1:.2f} Ex: If the input is: 5.0 1.5 3.2 Then the output is: 172.47 361.66 3.50 13.13 461710.3116374.qx3zqy7 LAB ACTIVITY 2.14.1: LAB: Using math functions 1 import math 2x = float(input( "Enter x: ")) 3 y = float(input("Enter y: ")) 4 z float(input( "Enter z: ")) 1 5 pow_x_z X**Z 6 pow_x_y_z = x**(y**z) 7 abs_x_y= abs(x - y) Develop mode Submit mode main.py 8 sqrt_x_z = (x**z)**0.5 9 print (f' {pow_x_z:.2f} {pow_x_y_z:.2f} {abs_x_y:.2f} {sqrt_x_z:.2f}'D] Enter program input (optional) 5.0 1.5 0/10 Load default template... 4 Run your program as often as you'd like, before submitting for grading. Below, type any…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
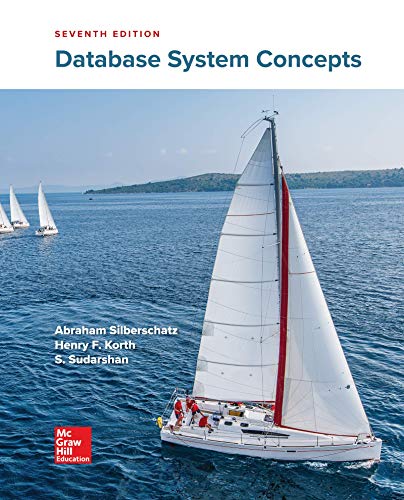
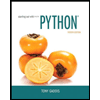
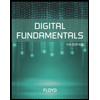
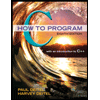
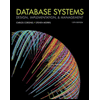
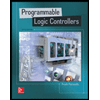