Java Constructor(String) This constructor will take a string of digits(no commas) and turn it into an UnboundedInt object (MUST BE STRING INPUT) UnboundedInt add (UnboundedInt ) A method that adds the current UnboundedInt with a passed in one. The return is a new UnboundedInt. UnboundedInt multiply (UnboundedInt ) - do this one last! A method that multiplies the current UnboundedInt with a passed in one. The return is a new UnboundedInt. void addEnd ( int ) -optional method (helpful) A method to add a new element at the end of the sequence , used for building up each higher term in a single sequence. (i.e. adding a new IntNode to the linked list) UnboundedInt clone( ) a method that returns a copy of the original structure boolean equals ( Object ) a method that returns true if linked list represents the same numerical number as the input parameter. False otherwise. Overrides method in Object class. String toString ( ) creates a string of all elements in order separated by commas, making sure leading zeros are added when needed. (i.e. 12,005,016 or 34,000 ) Throw an IllegalStateException if the sequence is empt
Java
Constructor(String)
This constructor will take a string of digits(no commas) and turn it into an UnboundedInt object (MUST BE STRING INPUT)
UnboundedInt add (UnboundedInt )
A method that adds the current UnboundedInt with a passed in one. The return is a new UnboundedInt.
UnboundedInt multiply (UnboundedInt ) - do this one last!
A method that multiplies the current UnboundedInt with a passed in one. The return is a new UnboundedInt.
void addEnd ( int ) -optional method (helpful)
A method to add a new element at the end of the sequence , used for building up each higher term in a single sequence. (i.e. adding a new IntNode to the linked list)
UnboundedInt clone( )
a method that returns a copy of the original structure
boolean equals ( Object )
a method that returns true if linked list represents the same numerical number as the input parameter. False otherwise. Overrides method in Object class.
String toString ( )
creates a string of all elements in order separated by commas, making sure leading zeros are added when needed. (i.e. 12,005,016 or 34,000 )
Throw an IllegalStateException if the sequence is empty

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

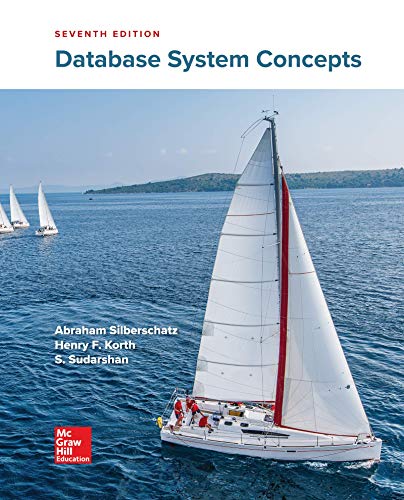
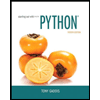
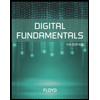
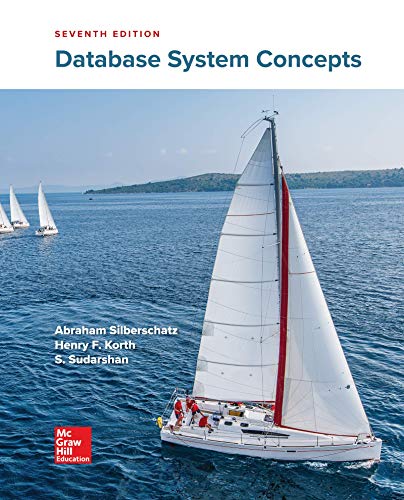
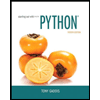
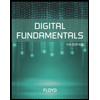
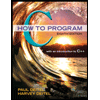
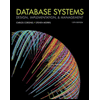
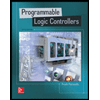