write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves.
ON PYTHON IDLE
In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:
At the beginning, your program should draw an empty chess board and prompt the user to enter a move:
Welcome to the Chess Game!
a b c d e f g h
+---+---+---+---+---+---+---+---+
8| | | | | | | | |8
+---+---+---+---+---+---+---+---+
7| | | | | | | | |7
+---+---+---+---+---+---+---+---+
6| | | | | | | | |6
+---+---+---+---+---+---+---+---+
5| | | | | | | | |5
+---+---+---+---+---+---+---+---+
4| | | | | | | | |4
+---+---+---+---+---+---+---+---+
3| | | | | | | | |3
+---+---+---+---+---+---+---+---+
2| | | | | | | | |2
+---+---+---+---+---+---+---+---+
1| | | | | | | | |1
+---+---+---+---+---+---+---+---+
a b c d e f g h
Enter a chess piece and its position or type X to exit:
A move is represented as a three-character string where the first character corresponds to a letter, a chess piece initial: K stands for king, Q - queen, B - bishop, N - knight, and R - rook (a chariot). Pawns do not have initials, and you will not be tested on their implementation. The next character in the move is the position on the board that corresponds to one of eight columns (called files). The columns (files) are labeled as a, b, c, d, e, f, g, h. The third character is a digit that corresponds to one of eight rows (called ranks). The rows (ranks) are labeled as 1, 2, 3, 4, 5, 6, 7, 8. For example, if the user enters Re6, your program should generate the following output where R is the initial for a rook, and all possible moves of the rook are marked by x:
a b c d e f g h
+---+---+---+---+---+---+---+---+
8| | | | | x | | | |8
+---+---+---+---+---+---+---+---+
7| | | | | x | | | |7
+---+---+---+---+---+---+---+---+
6| x | x | x | x | R | x | x | x |6
+---+---+---+---+---+---+---+---+
5| | | | | x | | | |5
+---+---+---+---+---+---+---+---+
4| | | | | x | | | |4
+---+---+---+---+---+---+---+---+
3| | | | | x | | | |3
+---+---+---+---+---+---+---+---+
2| | | | | x | | | |2
+---+---+---+---+---+---+---+---+
1| | | | | x | | | |1
+---+---+---+---+---+---+---+---+
a b c d e f g h
Enter a chess piece and its position or type X to exit:
If the user enters Kd5, your program should produce the following output where K is the initial for the king, and all squares where the king can move are marked with x:
a b c d e f g h
+---+---+---+---+---+---+---+---+
8| | | | | | | | |8
+---+---+---+---+---+---+---+---+
7| | | | | | | | |7
+---+---+---+---+---+---+---+---+
6| | | x | x | x | | | |6
+---+---+---+---+---+---+---+---+
5| | | x | K | x | | | |5
+---+---+---+---+---+---+---+---+
4| | | x | x | x | | | |4
+---+---+---+---+---+---+---+---+
3| | | | | | | | |3
+---+---+---+---+---+---+---+---+
2| | | | | | | | |2
+---+---+---+---+---+---+---+---+
1| | | | | | | | |1
+---+---+---+---+---+---+---+---+
a b c d e f g h
Enter a chess piece and its position or type X to exit:
If the user does not enter a valid move that is included in all possible combinations of chess piece initials (K, Q, B, N, R), files (a, b, c, d, e, f, g, h), and ranks (1, 2, 3, 4, 5, 6, 7, 8), then the program should reprint the prompt:
Enter a chess piece and its position or type X to exit:
If the user enters X (or x), then the program should print 'Goodbye!' and terminate:
Goodbye!
NOTE: The user can use either upper or lowercase letters, for example X or x should result in the termination of the program, Ke3, KE3, kE3, or ke3 means the same move.
The class Chess_Piece has four methods init, get_index, get_name, and moves. The constructor method init() creates an object with attributes position and color and updates the board by placing the chess piece on the board at a given position. The method get_name() should return the initial of the chess piece, and it is not implemented in the superclass. However, you need to implement it in all subclasses, for example, the method in a King class should return 'K', in a Rook class - 'R', etc. The method get_index() converts a position (that is used as a key in the board dictionary) into a tuple of indexes where the first index is a column number, and the second index is a row number).

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

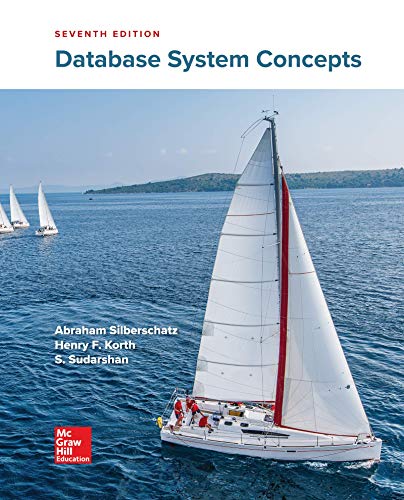
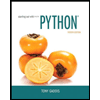
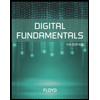
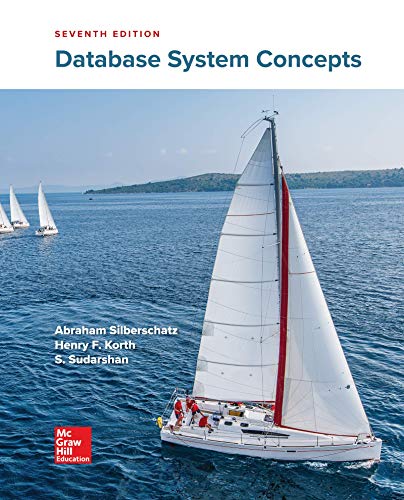
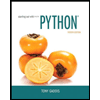
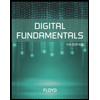
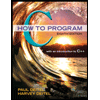
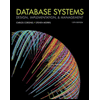
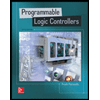