Write a program which requests two lengths in feet and inches (both whole numbers) and prints the sum of the lengths in feet and inches. For example, if the lengths are 5 ft. 4 in. and 8 ft. 11 in., your program should print 14ft. 3 in. (1 ft. = 12 in.) Your program must use a function that takes 4 parameters (2 for the feet and inches of the first length, and 2 for the feet an inches of the second length). The function must return a string with the answer. below is my code and l would like it to use a function that takes 4 parameters and also the function must return a string with the answer.
Write a
Your program must use a function that takes 4 parameters (2 for the feet and inches of the first length, and 2 for the feet an inches of the second length). The function must return a string with the answer.
below is my code and l would like it to use a function that takes 4 parameters and also the function must return a string with the answer.
using System;
public class main
{
public static void Sum(int f1, int i1, int f2, int i2)
{
int ftotal;
int itotal;
ftotal = f1 + f2;
itotal = i1 + i2;
while (itotal >= 12)
{
itotal = itotal - 12;
ftotal = ftotal + 1;
}
Console.WriteLine("\n The total feet is "); Console.WriteLine(ftotal);
Console.WriteLine("\n The total inches is "); Console.WriteLine(itotal);
}
public static void Main()
{
Console.Write("Enter feet for distance 1: ");
int f1 = Convert.ToInt32(Console.ReadLine());
Console.Write("Enter inches for distance 1: ");
int i1 = Convert.ToInt32(Console.ReadLine());
Console.Write("Enter feet for distance 2: ");
int f2 = Convert.ToInt32(Console.ReadLine());
Console.Write("Enter inches for distance 2: ");
int i2 = Convert.ToInt32(Console.ReadLine());
Sum(f1, i1, f2, i2);
}
}
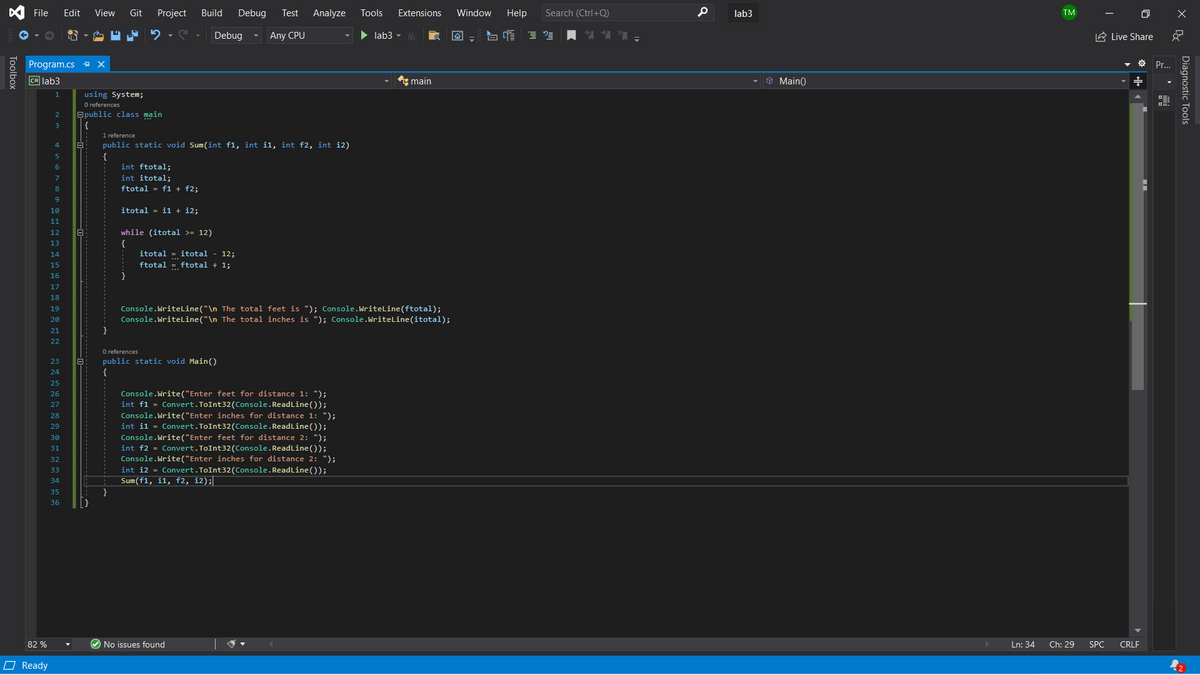

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

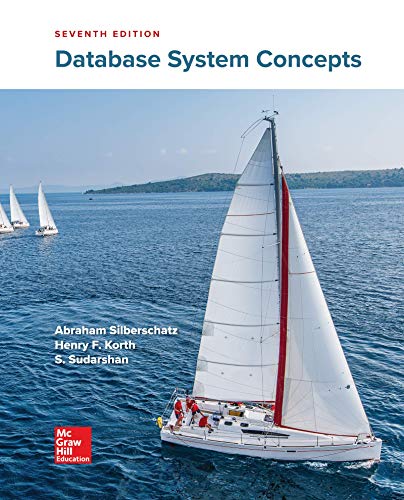
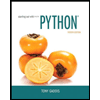
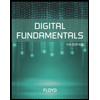
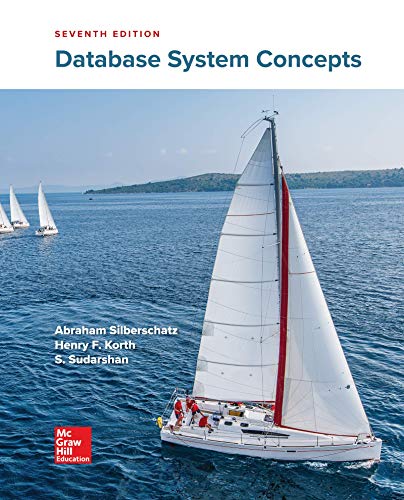
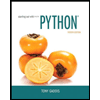
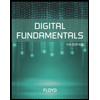
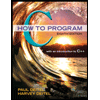
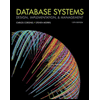
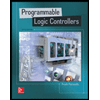