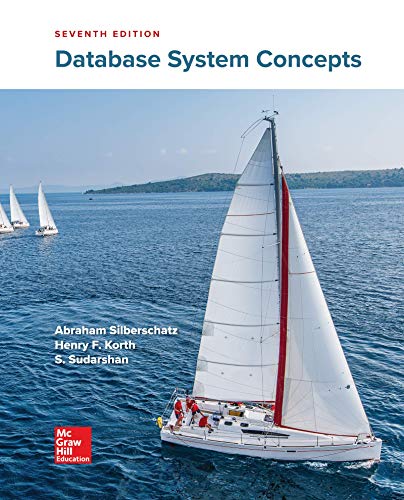
Concept explainers
Language: C++
Write a Program with Comments for your code to improve quick sort. The quick sort
Explain your Outputs or Answers for the following:
- (C) Give a list of n items (for example, an array of 10 integers) representing the worst-case scenario.
-
(D) Give a list of n items (for example, an array of 10 integers) representing the best-case scenario.
Please compile your answers in a file including all source code with comments, Explain your running Outputs or Answers in (A) ~(D).
Quicksort that was mentioned in the ClassNotes:
#include <iostream>
using namespace std;
// Function prototypes
void quickSort(int list[], int arraySize);
void quickSort(int list[], int first, int last);
int partition(int list[], int first, int last);
void quickSort(int list[], int arraySize)
{
quickSort(list, 0, arraySize - 1);
}
void quickSort(int list[], int first, int last)
{
if (last > first)
{
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex - 1);
quickSort(list, pivotIndex + 1, last);
}
}
// Partition the array list[first..last]
int partition(int list[], int first, int last)
{
int pivot = list[first]; // Choose the first element as the pivot
int low = first + 1; // Index for forward search
int high = last; // Index for backward search
while (high > low)
{
// Search forward from left
while (low <= high && list[low] <= pivot)
low++;
// Search backward from right
while (low <= high && list[high] > pivot)
high--;
// Swap two elements in the list
if (high > low)
{
int temp = list[high];
list[high] = list[low];
list[low] = temp;
}
}
while (high > first && list[high] >= pivot)
high--;
// Swap pivot with list[high]
if (pivot > list[high])
{
list[first] = list[high];
list[high] = pivot;
return high;
}
else
{
return first;
}
}
int main()
{
const int SIZE = 9;
int list[] = {1, 7, 3, 4, 9, 3, 3, 1, 2};
quickSort(list, SIZE);
for (int i = 0; i < SIZE; i++)
cout << list[i] << " ";
return 0;
}
![1
#include <iostream>
using namespace std;
3
// Function prototypes
void quickSort(int list[], int arraysize);
void quickSort (int list[), int first, int last);
int partition(int list[], int first, int last);
4
6.
7
8.
void quickSort(int list[], int arraysize)
{
quickSort (list, 0, arraysize - 1);
9
10
11
12
13
void quickSort (int list[], int first, int last)
15
14
16
if (last > first)
17
{
18
int pivotIndex - partition(list, first, last);
quickSort (list, first, pivotIndex - 1);
quickSort (list, pivotIndex + 1, 1last);
19
20
21
22
23
// Partition the array list[first..last]
int partition (int list[U, int first, int last)
24
25
26
{
int pivot = list[first]; // Choose the first element as the pivot
int low = first + 1; // Index for forward search
27
28
29
int high - last; // Index for backward search
30
31
while (high > low)
32
{
// Search forward from left
while (low <= high && list[low] <= pivot)
low++;
33
34
35
36
// Search backward from right
while (low <- high && list[high] > pivot)
37
38
39
high--;
40
// Swap two elements in the list
if (high > low)
41
42
43
{
44
int temp = list[high];
list(high] = list[low];
list(low] - temp;
45
46
47
48
}
49
while (high > first && list[high] >= pivot)
high--;
50
51
52
// Swap pivot with list[high]
if (pivot > list[high])
53
54
55
{
list[first] - list[high];
list[high] = pivot;
return high;
56
57
58
59
60
else
61
{
62
return first;
63
}
64
65
int main()
67
66
const int SIZE = 9;
int list[] = {1, 7, 3, 4, 9, 3, 3, 1, 2};
quickSort (list, SIZE);
for (int i - 0; i < SIZE; i++)
cout <« list[i] <<
68
69
70
71
72
%3D
";
73
74
return 0;
75](https://content.bartleby.com/qna-images/question/04d72593-097f-4b69-938e-8eb3398dcc08/ce6fdb8f-c51a-46cf-9645-62daf2f3377b/oylfoi_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Use C++arrow_forwardmake c+++ codearrow_forwardLanguage: C++ Write a Program with Comments for your code to improve quick sort. The quick sort algorithm presented in the ClassNotes-Sorting, it selects the first element in the list as the pivot. Revise it by selecting the median among the first, middle, and last elements in the list. Explain your Outputs or Answers for the following: (A) Analyze your algorithm, and give the results using order notation. (B) Give a list of n items (for example, an array of 10 integers) representing a scenario. Please compile your answers in a file including all source code with comments, Explain your running Outputs or Answers in (A) and (B). Can you label which one is for (A) and (B). Code that was mentioned: #include <iostream>using namespace std; // Function prototypesvoid quickSort(int list[], int arraySize);void quickSort(int list[], int first, int last);int partition(int list[], int first, int last); void quickSort(int list[], int arraySize){quickSort(list, 0, arraySize - 1);}…arrow_forward
- In C ++ You will need to sort whitespace-separated integers using three different sort algorithms (described here: http://theoryapp.com/selection-insertion-and-bubble-sort/ and https://en.wikipedia.org/wiki/Insertion_sort). For each of the three sorting algorithms (selection, insertion, and bubble), your program should output the initial list of numbers as well as after each swap. Note: You should use the Wikipedia entry for insertion sort. Do not perform swaps if they don't change the vector. Example Input: 1 1 6 3 8 4 2 3 9 2 4 Output: 1 Selection Sort 2 1 6 3 8 4 2 3 9 2 4 3 1 2 3 8 4 6 3 9 2 4 4 1 2 2 8 4 6 3 9 3 4 5 1 2 2 3 4 6 8 9 3 4 6 1 2 2 3 3 6 8 9 4 4 7 1 2 2 3 3 4 8 9 6 4 8 1 2 2 3 3 4 4 9 6 8 9 1 2 2 3 3 4 4 6 9 8 10 1 2 2 3 3 4 4 6 8 9 11 Insertion Sort 12 1 6 3 8 4 2 3 9 2 4 13 1 3 6 8 4 2 3 9 2 4 14 1 3 6 4 8 2 3 9 2 4 15 1 3 4 6 8 2 3 9 2 4 16 1 3 4 6 2 8 3 9 2 4 17 1 3 4 2 6 8 3 9 2 4 18 1 3 2 4 6 8 3 9 2 4 19 1 2 3 4 6 8 3…arrow_forwardIn the description of bubble sort in the previous question, the sorted section of the list was at the end of the list. In this question, bubble sort will maintain the sorted section of the beginning of the list. Make sure that you are still implementing bubble sort! a) Rewrite the English description of bubble sort from the previous question with the necessary changes so that the sorted elements are at the beginning of the list instead of the end. b) Using your English description of bubble sort, write an outline of the bubble sort algorithm in English. c) Write the bubble_sort_2(L) function. d) Write Nose test cases for bubble_sort_2.arrow_forwardIn C++, write a program that reads in an array of type int. You may assume that there are fewer than 20 entries in the array. The output must be a two-column list. The first column is a list of the distinct array elements and the second column is the count of the number of occurences of each element.arrow_forward
- Language: C++ Write a Program with Comments for your code to improve quick sort. The quick sort algorithm presented in the ClassNotes-Sorting, it selects the first element in the list as the pivot. Revise it by selecting the median among the first, middle, and last elements in the list.Explain your Outputs or Answers for the following: (A) Analyze your algorithm, and give the results using order notation. (B) Give a list of n items (for example, an array of 10 integers) representing a scenario. Please compile your answers in a file including all source code with comments, Explain your running Outputs or Answers in (A) and (B). Quicksort that was mentioned in the ClassNotes: #include <iostream>using namespace std; // Function prototypesvoid quickSort(int list[], int arraySize);void quickSort(int list[], int first, int last);int partition(int list[], int first, int last); void quickSort(int list[], int arraySize){quickSort(list, 0, arraySize - 1);} void quickSort(int…arrow_forwardSort Realize direct insertion sort, half insertion sort, bubble sort, quick sort, select sort, heap sort and merge sort. Raw data is generated randomly. For different problem size, output the number of comparisons and moves required by various sorting algorithms When the program is running, input the problemsize from the keyboard, the source data is randomly generated by the system, and then output the comparison times and movement times required by various sorting algorithms under this problem scale.. Do this in C language..the attached picture is just to show you how the program should bearrow_forwardC++ Nested loops: Print seats. Given numRows and numColumns, print a list of all seats in a theater. Rows are numbered, columns lettered, as in 1A or 3E. Print a space after each seat, including after the last. Ex: numRows = 2 and numColumns = 3 prints:1A 1B 1C 2A 2B 2C #include <iostream>using namespace std; int main() { int numRows; int numColumns; int currentRow; int currentColumn; char currentColumnLetter; cin >> numRows; cin >> numColumns; /* Your solution goes here */ cout << endl; return 0;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
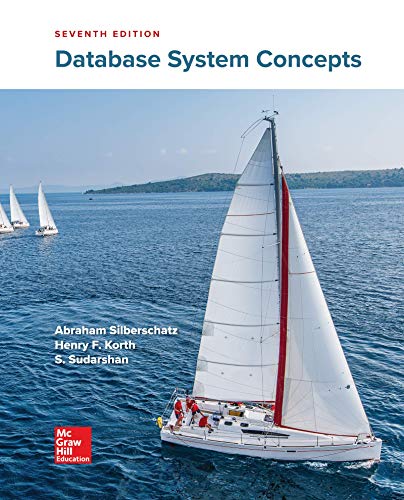
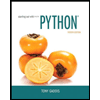
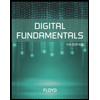
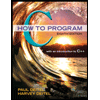
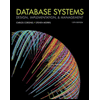
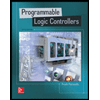