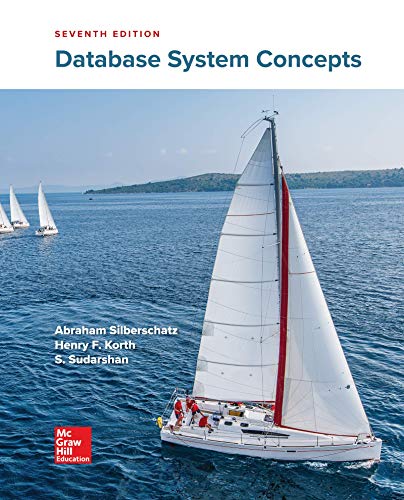
Problem Statement: Consider an input string TAM of letters ‘A’, ‘M’, and ‘T’. This string, which is given by the user, ends with ‘#’. It should be stored in a table (or array), called TAMUK. The number of each of these letters is unknown. We have a function, called SWAP(TAM,i,j), which places the ith letter in the jth entry of string TAM and the jth letter in the ith entry of TAM. Note that SWAP(TAM,i,j) is defined for all integers i and j between 0 and length(TAM)–1, where length(TAM) is the number of letters of TAM.
1. Using our algorithmic language, write an
- Constraint 1: Each letter (‘A’, ‘M’, or ‘T’) is evaluated only once.
- Constraint 2: The function SWAP(TAM,i,j) is used only when it is necessary.
- Constraint 3: No extra space can be used by the algorithm Sort_TAM. In other words,only the array TAMUK can be used to sort the ‘A’, ‘M’, or ‘T’.
- Constraint 4: You cannot count the number of each letter ‘A’, ‘M’, or ‘T’.
2. Show that the algorithm Sort_TAM is correct using an informal proof (i.e., discussion).
3. Give a program corresponding to Sort_TAM using your favorite
Want to solve this program using "R" programming

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- In C++ language For number 2 through 4 create an integer array with 100 randomly generated values between 0 and 99; pass this array into all subsequent functions. Place code in your main to call all the methods and demonstrate they work correctly. Using just the at, length, and substr string methods and the + (concatenate) operator, write a function that accepts a string s, a start position p, and a length l, and returns a subset of s with the characters starting at position p for a length of l removed. Don’t forget that strings start at position 0. Thus (“abcdefghijk”, 2, 4) returns “abghijk” Create a function that accepts the integer array described above returns the standard deviation of the values in a. The standard deviation is a statistical measure of the average distance each value in an array is from the mean. To calculate the standard deviation, you first call a second mean function that you need to write (do not use a built in gadget. Then sum the square of the difference…arrow_forwardC++arrow_forwardcan you write in C++arrow_forward
- Q2: In this question, you will write a Python function to compute the Hadamard product of two matrices. The Hadamard product is the "component-wise" product of two matrices. That is, each entry of the product matrix is equal to the product of its corresponding entries of the input matrices. Here is an example: a11 a12 a21 a22 a23 a31 a13 SH a32 033 A b11 b12 b13 b21 b22 b23 b31 b32 b33. B = [an bu a21 b21 a31 b31 a12 b12 a22 b22 a32 b32 a13 b13 a23 b23 a33 b33. Hadamard product of A and B Write a Python function to compute the Hadamard product of two matrices as follows. a) The inputs to the function are two matrices. b) The function should check whether the two matrices have the same size. If not, it should print "The input matrices are not of the same size". c) If two matrices are of the same size, the function computes the Hadamard product and returns the answer. Use for loops to compute the Hadamard product.arrow_forwardPlease solve using C language The function get_tokens gets a string str, and a char delim, and returns the array with the tokens in the correct order. The length of the array should be the number of tokens, computed in count_tokens. char** get_tokens(const char* str, char delim); For example: ● get_tokens("abc-EFG--", '-') needs to return ["abc","EFG"] ● get_tokens("++a+b+c", '+') needs to return ["a","b","c"]. ● get_tokens("***", '*') needs to return either NULL or an empty array. Note that the returned array and the strings in it must all be dynamically allocated.arrow_forwardJupyter Notebook Fixed Income - Certicificate of Deposit (CD) - Compound Interest Schedule An interest-at-maturity CD earns interest at a compounding frequency, and pays principal plus all earned interest at maturity. Write a function, called CompoundInterestSchedule, that creates and returns a pandas DataFrame, where each row has: time (in years, an integer starting at 1), starting balance, interest earned, and ending balance, for an investment earning compoundedinterest. Use a for(or while) loop to create this table. The equation for theith year's ending balance is given by: Ei =Bi (1+r/f)f where: Ei is year i's ending balance Bi is year i's beginning balance (note: B1 is the amount of the initial investment (principal) r is the annual rate of interest (in decimal, e.g., 5% is .05) f is the number of times the interest rate compounds (times per year) The interest earned for a given year is Ei - Bi Note the term of the investment (in years) is not in the above equation; it is used…arrow_forward
- Given a file of unsorted words with mixed case: read the entries in the file and sort those words lexicographically. The program (in c++) should then prompt the user for an index, and display the word at that index. Since you must store the entire list in an array, you will need to know the length. The "List of 1000 Mixed Case Words" contains 1000 words. You are guaranteed that the words in the array are unique, so you don't have to worry about the order of, say, "bat" and "Bat."arrow_forwardGiven a double precision floating point number with a decimal point, write a function named prg_question 3 to return the whole number and decimal part of the number as a std::pair object of integers with first part being the whole number part and the second part being the fraction or decimal part. For example given the number 3.14, the "first" part of the pair object must be 3 and the "second" must be 14. Do the following: 1. Follow UMPIRE process to get the algorithm. Write only the algorithm as code comments 2. Implement your function 3. Test your function for numbers 3.14159 and 2.71828. Please follow the test criteria given in the code templatearrow_forwardPYTHON Problem Statement Given a list of numbers (nums), for each element in nums, calculate how many numbers in the list are smaller than it. Write a function that does the calculation and returns the result (as a list). For example, if you are given [6,5,4,8], your function should return [2, 1, 0, 3] because there are two numbers less than 6, one number less than 5, zero numbers less than 4, and three numbers less than 8. Sample Input smaller_than_current([6,5,4,8]) Sample Output [2, 1, 0, 3]arrow_forward
- Write a C++ Program that does the following: Implement a sort function using a vector.Ask the user to enter numbers to be sorted. Add them to the vector. The user will signal the end of input bygiving you a negative number.Then, sort the vector. Because this is a vector, you don’t need to pass the number of entriesin the vector.Then, print out the vector in order.Remember the following things about vectors.a) You declare a vector v of ints by saying vector⟨int⟩ v;b) You add elements to a vector by saying v.push back(thing to be added);c) You access elements of a vector with [ ] just like an array;d) One of the vector member functions is .length()arrow_forwardWrite a C++ program that reads a list of numbers from a file into an array, then uses that array to find the average of all the numbers, the average of the positive and negative numbers (0 is neither positive nor negative!), and the largest number. The point is to be able to store data in an array and do things with it Your program must contain at least the following four functions... read_list() This function will take as input parameters an array of integers and a string filename. It will open that file and read in numbers, storing them in the array, stopping at the end of the file. Remember that there are tricky issues with extra whitespace at the end of the file. You can assume the file contains only integers. The function will return the number of numbers that were read in.Note: In versions of Visual C++ pre-2010, when using the open function with a string argument, you must call the c_str() function on the string variable, such as:my_input_stream.open( filename.c_str() ) 2.…arrow_forwardUsing either pseudocode of C++ code, write a function that takes three parameters and performs a sequential search. The first parameter is an array of integers. The second parameter is an integer representing the size of the array. The third parameter takes the value to be search form. The function should return the subscripts at which the value is found or -1 is the array does not contain the search term. Please dont use vectors and explain each steparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
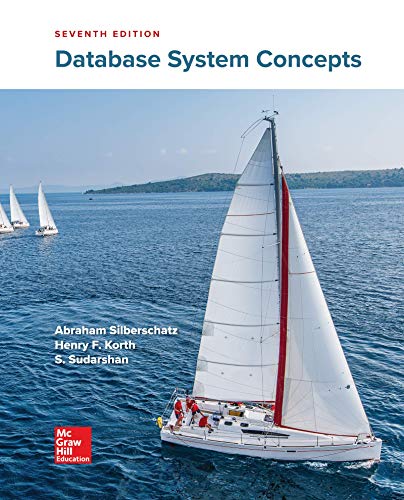
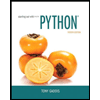
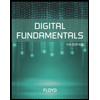
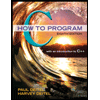
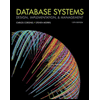
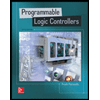