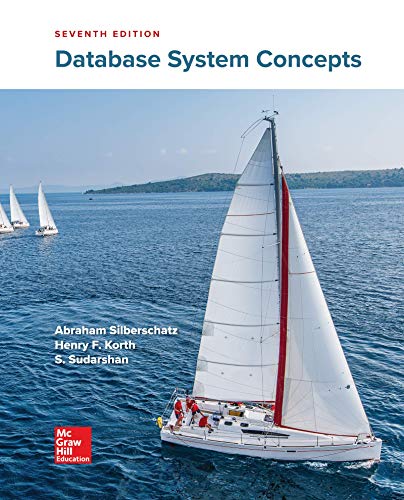
Language: C++
Write a Program with Comments for your code to improve quick sort. The quick sort
Revise it by selecting the median among the first, middle, and last elements in the list.
Explain your Outputs or Answers for the following:
-
(A) Analyze your algorithm, and give the results using order notation.
-
(B) Give a list of n items (for example, an array of 10 integers) representing a scenario.
Please compile your answers in a file including all source code with comments, Explain your running Outputs or Answers in (A) and (B). Can you label which one is for (A) and (B).
Code that was mentioned:
#include <iostream>
using namespace std;
// Function prototypes
void quickSort(int list[], int arraySize);
void quickSort(int list[], int first, int last);
int partition(int list[], int first, int last);
void quickSort(int list[], int arraySize)
{
quickSort(list, 0, arraySize - 1);
}
void quickSort(int list[], int first, int last)
{
if (last > first)
{
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex - 1);
quickSort(list, pivotIndex + 1, last);
}
}
// Partition the array list[first..last]
int partition(int list[], int first, int last)
{
int pivot = list[first]; // Choose the first element as the pivot
int low = first + 1; // Index for forward search
int high = last; // Index for backward search
while (high > low)
{
// Search forward from left
while (low <= high && list[low] <= pivot)
low++;
// Search backward from right
while (low <= high && list[high] > pivot)
high--;
// Swap two elements in the list
if (high > low)
{
int temp = list[high];
list[high] = list[low];
list[low] = temp;
}
}
while (high > first && list[high] >= pivot)
high--;
// Swap pivot with list[high]
if (pivot > list[high])
{
list[first] = list[high];
list[high] = pivot;
return high;
}
else
{
return first;
}
}
int main()
{
const int SIZE = 9;
int list[] = {1, 7, 3, 4, 9, 3, 3, 1, 2};
quickSort(list, SIZE);
for (int i = 0; i < SIZE; i++)
cout << list[i] << " ";
return 0;
}
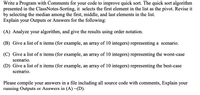
![```cpp
#include <iostream>
using namespace std;
// Function prototypes
void quickSort(int list[], int arraySize);
void quickSort(int list[], int first, int last);
int partition(int list[], int first, int last);
void quickSort(int list[], int arraySize)
{
quickSort(list, 0, arraySize - 1);
}
void quickSort(int list[], int first, int last)
{
if (last > first)
{
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex - 1);
quickSort(list, pivotIndex + 1, last);
}
}
// Partition the array list[first..last]
int partition(int list[], int first, int last)
{
int pivot = list[first]; // Choose the first element as the pivot
int low = first + 1; // Index for forward search
int high = last; // Index for backward search
while (high > low)
{
// Search forward from left
while (low <= high && list[low] <= pivot)
low++;
// Search backward from right
while (low <= high && list[high] > pivot)
high--;
// Swap two elements in the list
if (high > low)
{
int temp = list[high];
list[high] = list[low];
list[low] = temp;
}
}
while (high > first && list[high] >= pivot)
high--;
// Swap pivot with list[high]
if (pivot > list[high])
{
list[first] = list[high];
list[high] = pivot;
return high;
}
else
{
return first;
}
}
int main()
{
const int SIZE = 9;
int list[] = {1, 7, 3, 4, 9, 3, 3, 1, 2};
quickSort(list, SIZE);
for (int i = 0; i < SIZE; i++)
cout << list[i] << " ";
return 0;
}
```
**Explanation:**
This C++ code implements the Quick Sort algorithm, which is a well-known sorting algorithm. The code is divided into several parts:
1. **Function Prototypes:**
- `](https://content.bartleby.com/qna-images/question/04d72593-097f-4b69-938e-8eb3398dcc08/a63f0781-d1a2-4ef3-a80b-9615b4dfae18/u4cagss_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Request: Can you please help me with the following? By the way, you don't need to answer the part "provide a time complexity of merge sort" as I can answer that, and if you will provide me some code, can it please be in C or C++? Thank you. Question: Use the array below to write a program using merge sort and provide a time complexity of merge sort. 4 12 7 8 2 5 15arrow_forwarda C++ program that will load 100 integers into array n. Separate the even from the odd valued elements. The procedure should place the even values in ascending order starting from the first location of the original array and place the odd values in ascending order starting from the last position of the original array. Attached photo is the supposed result of the program created.arrow_forwardSuppose you are given a vector x. Write first a pseudo-code or algorithm that would print out the sum of all the entries in x. You will probably want to use a some kind of for loop construction to achieve this. 1: for i = start : finish do 2: statements 3: end for Now use the start:increment:finish notation, and modify your procedure slightly, to print out the sum of the odd elements in the array (that is, the elements in entries 1, 3, 5, and so on). Implement and test your algorithm with an arbitrary array of real numbers using Python.arrow_forward
- Use C++arrow_forwardSort Realize direct insertion sort, half insertion sort, bubble sort, quick sort, select sort, heap sort and merge sort. Raw data is generated randomly. For different problem size, output the number of comparisons and moves required by various sorting algorithms When the program is running, input the problem size from the keyboard, the source data is randomly generated by the system, and then output the comparison times and movement times required by various sorting algorithms under this problem scale. do this in C langauge ...it should ne able to do like the attached picturearrow_forwardSolve the following codes below and discuss how array, stack, and queues are used in each problem. Must have comments. 1. Write a python program that takes two three-dimensional numeric datasets andperform elementwise addition. 2. Write a python program that ask a user to enter a n-by-m numeric matrix andperform matrix addition and multiplication. 3. Write a python program that asks user to input 10 positive and negative integers andbe stored in an array of 20 indices, The program will then rearrange the arrayelements so that all negative numbers appear before all positive numbers.arrow_forward
- Select problem below. Your post must include: The problem statement. A description of your solution highlighting the use of the split(), join(), lists and the different list operations involved in solving the problems. Include line numbers from your program dont use JAVA Make Username. Create a program that reads a full name as a single string, and and uses the split creates a username with the following rules: The first character of the username is the first character of the first name. The second character of the username is the first letter of the middle name, if one was provided. The rest of the characters will be a prefix of the last name, long enough to meet the length requirement. The username can have up to 8 characters only, and in lowercase. Here is a sample execution:arrow_forwardBinary Search of Strings1. Write a version of the selection sort algorithm presented in the unit, which is usedto search a list of strings.2. Write a version of the binary search algorithm presented in the unit, which isused to search a list of strings. (Use the selection sort that you designed aboveto sort the list of strings.)3. Create a test program that primes the list with a set of strings, sorts the list, andthen prompts the user to enter a search string. Your program should then searchthe list using your binary search algorithm to determine if the string is in the list.Allow the user to continue to search for strings until they choose to exit theprogramarrow_forwardLanguage: C++ Write a Program with Comments for your code to improve quick sort. The quick sort algorithm presented in the ClassNotes-Sorting, it selects the first element in the list as the pivot. Revise it by selecting the median among the first, middle, and last elements in the list.Explain your Outputs or Answers for the following: (A) Analyze your algorithm, and give the results using order notation. (B) Give a list of n items (for example, an array of 10 integers) representing a scenario. Please compile your answers in a file including all source code with comments, Explain your running Outputs or Answers in (A) and (B). Quicksort that was mentioned in the ClassNotes: #include <iostream>using namespace std; // Function prototypesvoid quickSort(int list[], int arraySize);void quickSort(int list[], int first, int last);int partition(int list[], int first, int last); void quickSort(int list[], int arraySize){quickSort(list, 0, arraySize - 1);} void quickSort(int…arrow_forward
- Language: C++ Write a Program with Comments for your code to improve quick sort. The quick sort algorithm presented in the ClassNotes-Sorting, it selects the first element in the list as the pivot. Revise it by selecting the median among the first, middle, and last elements in the list.Explain your Outputs or Answers for the following: (C) Give a list of n items (for example, an array of 10 integers) representing the worst-case scenario. (D) Give a list of n items (for example, an array of 10 integers) representing the best-case scenario. Please compile your answers in a file including all source code with comments, Explain your running Outputs or Answers in (A) ~(D). Quicksort that was mentioned in the ClassNotes: #include <iostream>using namespace std; // Function prototypesvoid quickSort(int list[], int arraySize);void quickSort(int list[], int first, int last);int partition(int list[], int first, int last); void quickSort(int list[], int arraySize){quickSort(list, 0,…arrow_forwardSort Realize direct insertion sort, half insertion sort, bubble sort, quick sort, select sort, heap sort and merge sort. Raw data is generated randomly. For different problem size, output the number of comparisons and moves required by various sorting algorithms When the program is running, input the problemsize from the keyboard, the source data is randomly generated by the system, and then output the comparison times and movement times required by various sorting algorithms under this problem scale.. Do this in C language..the attached picture is just to show you how the program should bearrow_forwardSort Realize direct insertion sort, half insertion sort, bubble sort, quick sort, select sort, heap sort and merge sort. Raw data is generated randomly. For different problem size, output the number of comparisons and moves required by various sorting algorithms When the program is running, input the problemsize from the keyboard, the source data is randomly generated by the system, and then output the comparison times and movement times required by various sorting algorithms under this problem scale.. Do this in C++ language..do it according to the above requirementarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
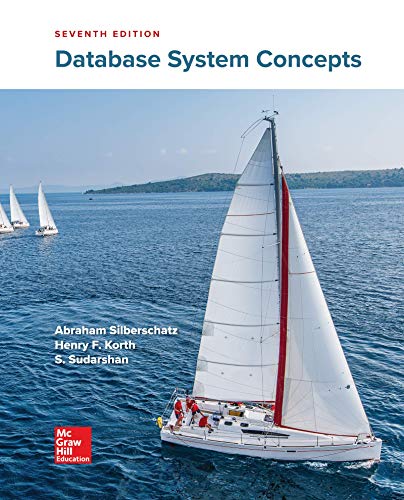
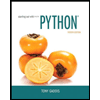
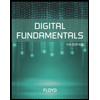
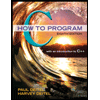
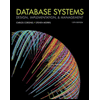
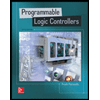