Write a Python module that stores books' titles and ISBN(10 digits) in the library first, then perform several operations. The program should print a main menu that gives a list of options. Option 1: Add books to the library The librarian should be able to enter all books by title and unique ISBN space-separated. The ISBN must be unique, ten digits, and should contain numbers only. If the ISBN is not in the required format, or is duplicated, print ‘Invalid entry’ and keep prompting for valid entries until all books are entered.
Write a Python module that stores books' titles and ISBN(10 digits) in the library first, then perform several operations.
The program should print a main menu that gives a list of options.
Option 1: Add books to the library
The librarian should be able to enter all books by title and unique ISBN space-separated. The ISBN must be unique, ten digits, and should contain numbers only. If the ISBN is not in the required format, or is duplicated, print ‘Invalid entry’ and keep prompting for valid entries until all books are entered.
Option 2: Print available books in the library
The librarian should be able to print all the available books in the library including the title and ISBN.
Option 3: Create book collections
The librarian should be able to create book collections. The program should first ask for the number of books per collection. Next, prompt the librarian to enter all the books in each collection using the book ISBN until there are no more books left in the library.
Option 4: Sort books in the collections
The librarian should be able to sort the books in a collection by ascending or descending order of ISBN. The program should prompt the librarian for the sorting order.
Option 5: Delete a collection
The librarian should be able to delete a book collection. Once a collection is deleted, it should be added back to the library of available books. If there is no collection available, the program should print ‘Invalid entry’.
Option 6:
The program should quit.
There is an image attached for the minimum required functions for this program, and example output.
A dictionary is recommended for the library. If the total number of books is not a multiple of the collection size, then the last collection will have fewer books.
If the librarian chooses option 2 while there are no books in the library, print ‘Invalid entry’.
A book can be in only one collection.
Books available in the library should change depending on whether collections are created or deleted.
When printing the book title and ISBN, the required alignment is 20 spaces per column. The program should always check all invalid operations. Print the standard error message ‘Invalid entry’ for invalid operations.
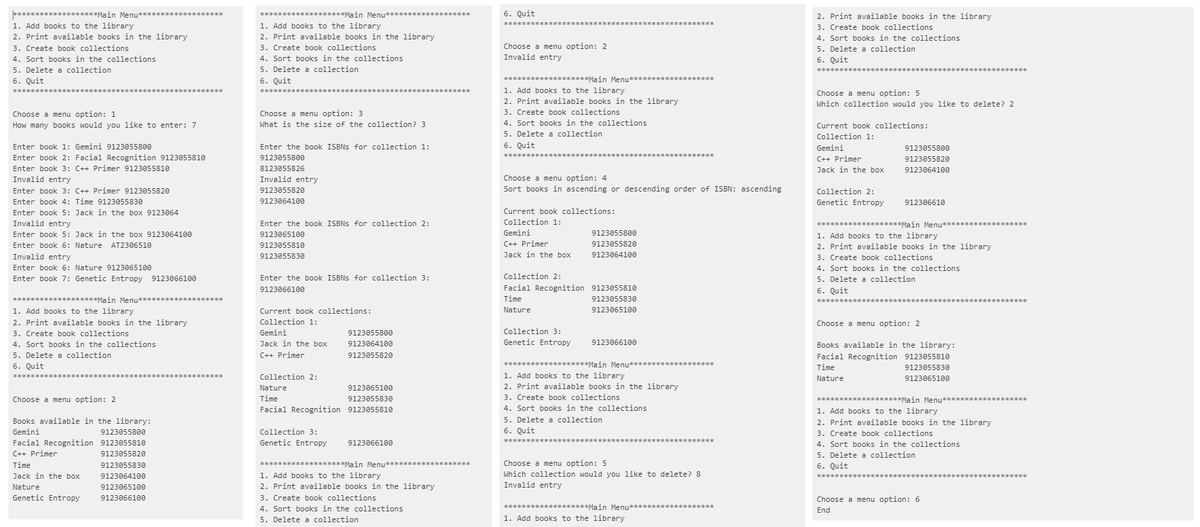
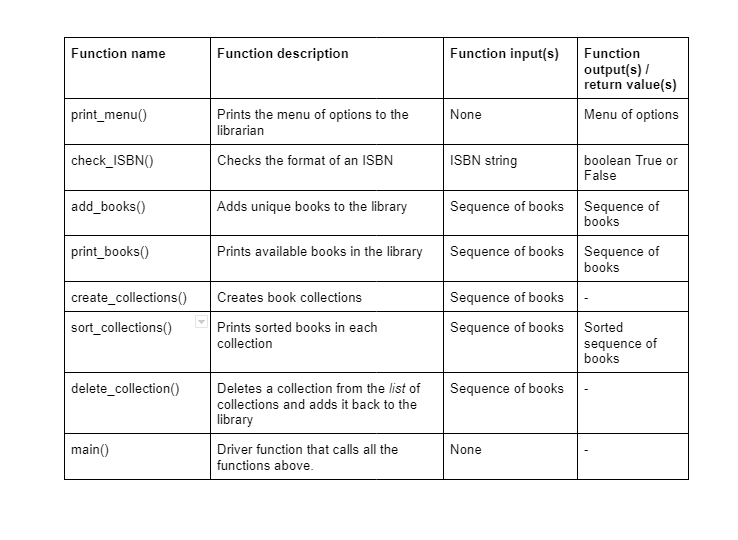

Step by step
Solved in 2 steps with 2 images

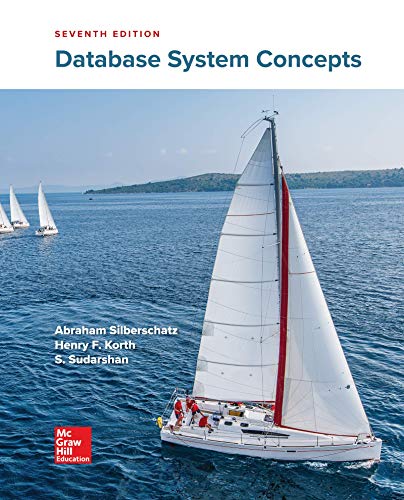
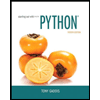
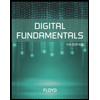
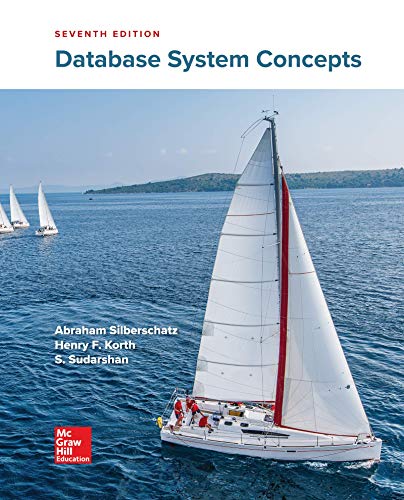
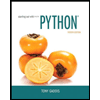
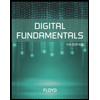
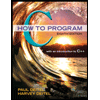
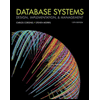
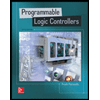