Inserting Nodes into the List: In the following code (The Code is written By C): there are 3 functions : display, insert_begin, and delete. The request is: add two functions : First Function (insert_last) : to insert values from last. Second Function (insert_mid): to insert values from Middle. Please Run the code before send it... thanks... >>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> The code: #include #include #include #include struct node { int info; struct node* next; }; struct node* start; void display(); void insert_begin(); void delete(); int main() { int choice; start = NULL; while (1) { printf("\n\t\t MENU \t\t\n"); printf("\n1.Display\n"); printf("\n2.Insert at the beginning\n"); printf("\n3.Delete\n"); printf("\n4.Exit\n"); printf("Enter your choice:\t"); scanf_s("%d", &choice); switch (choice) { case 1: display(); break; case 2: insert_begin(); break; case 3: delete(); break; case 4: exit(0); break; default: printf("\nWrong Choice.\n"); break; } } return 0; } void display() { struct node* temp; if (start == NULL) { printf("\nList is Empty"); return; } else { temp = start; printf("\n The list elements are:\n"); //start from the beginning while (temp != NULL) { printf("%d - \t", temp->info); temp = temp->next; } } } //inserting nodes into the list //function insert values from beginning void insert_begin() { struct node* tempData; int a; // allocate memory to the node tempData = (struct node*)malloc(sizeof(struct node)); if (tempData == NULL) { printf("Out of Memory"); return; } printf("Enter data for the node:\n"); scanf_s("%d", &tempData->info); a = tempData->info; if (start == NULL) { start = tempData; } else { tempData->next = start; start = tempData; } printf("%d is inserted.\n", a); } void delete() { struct node* ptr, * t; int x; if (start == NULL) { printf("List is empty\n"); return; } else { printf("Enter data to be deleted:\t"); scanf_s("%d", &x); if (x == start->info) { t = start; //assign the first node pointer to the next node pointer start = start->next; free(t); printf("\nThe %d is deleted.", x); } ptr = start; t = start->next; //navigate through the list while (t != NULL && t->info != x) { ptr = t; t = t->next; } if (t == NULL) { printf("%d does not exist\n", x); return; } else { ptr->next = t->next; } printf("%d is deleted.\n", x); free(t); } }
Inserting Nodes into the List:
In the following code (The Code is written By C): there are 3 functions :
display, insert_begin, and delete.
The request is: add two functions :
First Function (insert_last) : to insert values from last.
Second Function (insert_mid): to insert values from Middle.
Please Run the code before send it... thanks...
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
The code:
#include
#include
#include
#include
struct node
{
int info;
struct node* next;
};
struct node* start;
void display();
void insert_begin();
void delete();
int main()
{
int choice;
start = NULL;
while (1)
{
printf("\n\t\t MENU \t\t\n");
printf("\n1.Display\n");
printf("\n2.Insert at the beginning\n");
printf("\n3.Delete\n");
printf("\n4.Exit\n");
printf("Enter your choice:\t");
scanf_s("%d", &choice);
switch (choice)
{
case 1:
display();
break;
case 2:
insert_begin();
break;
case 3:
delete();
break;
case 4:
exit(0);
break;
default:
printf("\nWrong Choice.\n");
break;
}
}
return 0;
}
void display()
{
struct node* temp;
if (start == NULL)
{
printf("\nList is Empty");
return;
}
else
{
temp = start;
printf("\n The list elements are:\n");
//start from the beginning
while (temp != NULL)
{
printf("%d - \t", temp->info);
temp = temp->next;
}
}
}
//inserting nodes into the list
//function insert values from beginning
void insert_begin()
{
struct node* tempData;
int a;
// allocate memory to the node
tempData = (struct node*)malloc(sizeof(struct node));
if (tempData == NULL)
{
printf("Out of Memory");
return;
}
printf("Enter data for the node:\n");
scanf_s("%d", &tempData->info);
a = tempData->info;
if (start == NULL)
{
start = tempData;
}
else
{
tempData->next = start;
start = tempData;
}
printf("%d is inserted.\n", a);
}
void delete()
{
struct node* ptr, * t;
int x;
if (start == NULL)
{
printf("List is empty\n");
return;
}
else
{
printf("Enter data to be deleted:\t");
scanf_s("%d", &x);
if (x == start->info)
{
t = start;
//assign the first node pointer to the next node pointer
start = start->next;
free(t);
printf("\nThe %d is deleted.", x);
}
ptr = start;
t = start->next;
//navigate through the list
while (t != NULL && t->info != x)
{
ptr = t;
t = t->next;
}
if (t == NULL)
{
printf("%d does not exist\n", x);
return;
}
else
{
ptr->next = t->next;
}
printf("%d is deleted.\n", x);
free(t);
}
}

Step by step
Solved in 4 steps with 9 images

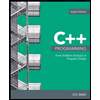
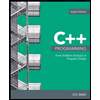