(Write C++ Code) (computer science) 1. Monitor class header file: Monitor.h Instructions: 1. Code the class specification file and name it as Monitor.h. You can code the constructor initialization steps in-line. The rest of the member functions should just be prototypes. The class specification should define the prototypes of each functions. Class name: Monitor Private Member Attributes: string brand string model int width (px) int height(px) int ppi double refreshRate (hz) char speakers (Y/N) int ports (no. of ports) string portType (list of port types separated by commas) Public Member Inline Functions Default Constructor with no parameters will initialize all numeric variables to 0. Constructor that accepts 3 int parameters for width, height, ppi. This constructor defaults the brand to "Generic", model to "Basic", the refreshRate to 60, speakers to 'N', ports to 1, and portType to "HDMI". Destructor (empty destructor) Public Member Functions as Prototypes: Prototype for Setters (Mutators) for each member attribute that takes parameter for the attribute and returns no value. Prototype for Getters (Accessors) for each member attribute returns the data type as specified in the attribute list. Prototype for getScreenSize() function that calculates and returns the diagonal size of the monitor in inches. Be sure the data types will not demote numbers in the calculations. Use the formula: Diagonal = √ (w/ppi)^2 + (h/ppi)^2 Use: sqrt() and pow(x,y) functions 4. Prototype for listMonitor() that takes no parameter and no return value but generates cout of the the Monitor as: Brand: Acer,Model: H243H,Screen size (pixels) 1920x1080 @ 70ppi, (diagonal) "result of getScreenSize" Monitor class - Monitor.cpp Instructions: 2. Code the class cpp file and name it as Monitor.cpp. This should contain the setters and getter functions that are not inline, including the getScreenSize function and listMonitor function. Return type should be int. You do not need to round the results of the calculation. Class name: Monitor Private Member Attributes: string brand string model int width (px) int height(px) int ppi double refreshRate (hz) char speakers (Y/N) int ports (no. of ports) string portType (list of port types separated by commas) Public Members Functions that are not inline: Setters (Mutators) for each member attribute Getters (Accessors) for each member attribute returns the data type as specified in the attribute list. getScreenSize() function that calculates the diagonal size of the monitor in inches. Be sure the data types will not demote numbers in the calculations. Use the formula below. listMonitor function that list Monitor specifications. Brand: Acer,Model: H243H,Screen size (pixels) 1920x1080 @ 70ppi, (diagonal) "result of getScreenSize" Diagonal = √ (w/ppi)^2 + (h/ppi)^2 Use: sqrt() and pow(x,y) functions Instructions: 1) Code main() function and name it getMonitors.cpp. 2) Instantiate an Acer object of the Monitor class and set width and height as 1920 x 1080, Brand as Acer, Model as H243H, and ppi as 70, 1 HDMI port, 60Hz refresh rate. Call the functions to calculate the Screen Size and write the output listing. Output should be: Brand: Acer,Model: H243H,Screen size (pixels) 1920x1080 @ 70ppi, (diagonal) "result of getScreenSize" 3) Instantiate a Sony object of the Monitor class and set width and height as 1440 x 980, Brand as Sony, Model as VX200, and ppi as 72, 1 HDMI port, 60Hz refresh rate. Call the functions to calculate the Screen Size and write the output listing the Sony monitor. Brand: Sony,Model: VX200,Screen size (pixels) 1440x980@ 72ppi, (diagonal) "result of getScreenSize" 4) Upload the getMonitors.cpp. Upload image file showing test run and results of getMonitors C++ program. Image can be generated from either console command line or IDE run dialogue. Draw a UML diagram for the Monitor class. Upload the image of the Monitor class UML diagram.
(Write C++ Code)
(computer science)
1. Monitor class header file: Monitor.h
Instructions:
1. Code the class specification file and name it as Monitor.h. You can code the constructor initialization steps in-line. The rest of the member functions should just be prototypes.
The class specification should define the prototypes of each functions.
Class name: Monitor
Private Member Attributes:
- string brand
- string model
- int width (px)
- int height(px)
- int ppi
- double refreshRate (hz)
- char speakers (Y/N)
- int ports (no. of ports)
- string portType (list of port types separated by commas)
Public Member Inline Functions
- Default Constructor with no parameters will initialize all numeric variables to 0.
- Constructor that accepts 3 int parameters for width, height, ppi. This constructor defaults the brand to "Generic", model to "Basic", the refreshRate to 60, speakers to 'N', ports to 1, and portType to "HDMI".
- Destructor (empty destructor)
Public Member Functions as Prototypes:
- Prototype for Setters (Mutators) for each member attribute that takes parameter for the attribute and returns no value.
- Prototype for Getters (Accessors) for each member attribute returns the data type as specified in the attribute list.
- Prototype for getScreenSize() function that calculates and returns the diagonal size of the monitor in inches. Be sure the data types will not demote numbers in the calculations. Use the formula:
Diagonal = √ (w/ppi)^2 + (h/ppi)^2
Use: sqrt() and pow(x,y) functions
4. Prototype for listMonitor() that takes no parameter and no return value but generates cout of the the Monitor as:
Brand: Acer,Model: H243H,Screen size (pixels) 1920x1080 @ 70ppi, (diagonal) "result of getScreenSize"
-
Monitor class - Monitor.cpp
Instructions:
2. Code the class cpp file and name it as Monitor.cpp. This should contain the setters and getter functions that are not inline, including the getScreenSize function and listMonitor function. Return type should be int. You do not need to round the results of the calculation.
Class name: Monitor
Private Member Attributes:- string brand
- string model
- int width (px)
- int height(px)
- int ppi
- double refreshRate (hz)
- char speakers (Y/N)
- int ports (no. of ports)
- string portType (list of port types separated by commas)
Public Members Functions that are not inline:
- Setters (Mutators) for each member attribute
- Getters (Accessors) for each member attribute returns the data type as specified in the attribute list.
- getScreenSize() function that calculates the diagonal size of the monitor in inches. Be sure the data types will not demote numbers in the calculations. Use the formula below.
- listMonitor function that list Monitor specifications.
Brand: Acer,Model: H243H,Screen size (pixels) 1920x1080 @ 70ppi, (diagonal) "result of getScreenSize"
Diagonal = √ (w/ppi)^2 + (h/ppi)^2
Use: sqrt() and pow(x,y) functions
-
Instructions:
1) Code main() function and name it getMonitors.cpp.
2) Instantiate an Acer object of the Monitor class and set width and height as 1920 x 1080, Brand as Acer, Model as H243H, and ppi as 70, 1 HDMI port, 60Hz refresh rate. Call the functions to calculate the Screen Size and write the output listing. Output should be:
Brand: Acer,Model: H243H,Screen size (pixels) 1920x1080 @ 70ppi, (diagonal) "result of getScreenSize"
3) Instantiate a Sony object of the Monitor class and set width and height as 1440 x 980, Brand as Sony, Model as VX200, and ppi as 72, 1 HDMI port, 60Hz refresh rate. Call the functions to calculate the Screen Size and write the output listing the Sony monitor.
Brand: Sony,Model: VX200,Screen size (pixels) 1440x980@ 72ppi, (diagonal) "result of getScreenSize"
4) Upload the getMonitors.cpp.
Upload image file showing test run and results of getMonitors C++ program. Image can be generated from either console command line or IDE run dialogue.
Draw a UML diagram for the Monitor class. Upload the image of the Monitor class UML diagram.

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

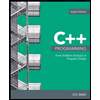
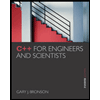
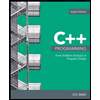
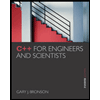