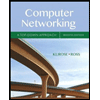
Modify this program to use namespaces and separate compilation. using c++
-Put the class definition and other function declarations
-Place the implementations in a separate file.
-Distribute the namespace definition across the two files.
-Place the demonstration program in a third file.
-To provide access to names in namespaces, you may use local using declarations such as using std::cout; or use local using directives such as using namespace std; inside a block, or qualify names using the names of namespaces, such as std::cout.
-You may not use global namespace directives such as the following which are not in a block and apply to the entire file: using namespace std;"
code
#include <iostream>
#include<string>
using namespace std;
class PFArrayD
{
public:
PFArrayD( );
//Initializes with a capacity of 50.
PFArrayD(int capacityValue);
PFArrayD(const PFArrayD& pfaObject);
void addElement(double element);
//Precondition: The array is not full.
//Postcondition: The element has been added.
bool full( ) const { return (capacity == used); }
//Returns true if the array is full, false otherwise.
int getCapacity( ) const { return capacity; }
int getNumberUsed( ) const { return used; }
void emptyArray( ){ used = 0; }
//Empties the array.
double& operator[](int index);
//Read and change access to elements 0 through numberUsed - 1.
PFArrayD& operator =(const PFArrayD& rightSide);
~PFArrayD( );
private:
double *a; //For an array of doubles
int capacity; //For the size of the array
int used; //For the number of array positions currently in use
};
PFArrayD::PFArrayD( ) :capacity(50), used(0)
{
a = new double[capacity];
}
PFArrayD::PFArrayD(int size) :capacity(size), used(0)
{
a = new double[capacity];
}
PFArrayD::PFArrayD(const PFArrayD& pfaObject)
:capacity(pfaObject.getCapacity( )), used(pfaObject.getNumberUsed( ))
{
a = new double[capacity];
for (int i = 0; i < used; i++)
a[i] = pfaObject.a[i];
}
void PFArrayD::addElement(double element)
{
if (used >= capacity)
{
cout << "Attempt to exceed capacity in PFArrayD.\n";
exit(0);
}
a[used] = element;
used++;
}
double& PFArrayD::operator[](int index)
{
if (index >= used)
{
cout << "Illegal index in PFArrayD.\n";
exit(0);
}
}
PFArrayD& PFArrayD::operator =(const PFArrayD& rightSide)
{
if (capacity != rightSide.capacity)
{
delete [] a;
a = new double[rightSide.capacity];
}
capacity = rightSide.capacity;
used = rightSide.used;
for (int i = 0; i < used; i++)
a[i] = rightSide.a[i];
return *this;
}
PFArrayD::~PFArrayD( )
{
delete[] a;
}
//PROGRAM TO TEST PFArrayD
#include <iostream>
using namespace std;
class PFArrayD
{
<The rest of the class definition is the same as in Display 10.10.>
};
void testPFArrayD( );
//Conducts one test of the class PFArrayD.
int main( )
{
cout << "This program tests the class PFArrayD.\n";
char ans;
do
{
testPFArrayD( );
cout << "Test again? (y/n) ";
cin >> ans;
} while ((ans == 'y') || (ans == 'Y'));
return 0;
}
void testPFArrayD( )
{
int cap;
cout << "Enter capacity of this super array: ";
cin >> cap;
PFArrayD temp(cap);
cout << "Enter up to " << cap << " nonnegative numbers.\n";
cout << "Place a negative number at the end.\n";
double next;
cin >> next;
while ((next >= 0) && (!temp.full( )))
{
temp.addElement(next);
cin >> next;
}
cout << "You entered the following "
<< temp.getNumberUsed( ) << " numbers:\n";
int index;
int count = temp.getNumberUsed( );
for (index = 0; index < count; index++)
cout << temp[index] << " ";
cout << endl;
cout << "(plus a sentinel value.)\n";
}
note:don't use chegg

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Project Statement (C++ Language) Your Project Statement should clearly describe the following, 1. A general explanation of the problem, expected input, and output. 2. Part A - Explaining the data structures and functions required to approach the solution to the problem. 3. Part B - Explaining the algorithms that can be used to program the functions mentioned in Part A, and the techniques to improve the efficiency of these algorithms.arrow_forwardNeed urgent help with Python Decorator creation Never used python decorator before Answer the following questions in the Python decorator context. Your answers should be written in a Markdown file. The quality of answers matters!! What is higher-order function and how it is different from functor? What are First-class objects? What is the significance of functions being First-class objects? What are inner functions? What is the major benefit of inner functions and why is it important for decorator Why @ symbol is called syntactic sugar? What's the biggest advantage of using it when decorators are used? How would it help Python's weak OOP encapsulation of Class? (Hint: google "Python @Property decorator")arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
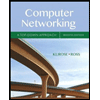
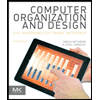
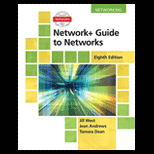
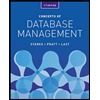
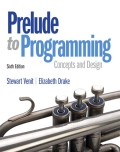
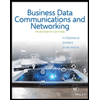