Write a program in C++ that defines a class named StringOps. Declare data members of this class as described below: • variable str of data type string. • variable size of data type integer which represents the length of the string str. Define member functions in StringOps as given below: parameterless and parameterized constructors that initialize the string variable str. void input () : This function accepts a string as input from the user and stores it in str. The length of this string is computed and stored in the variable size. • Overload operator + to create a new string obtained by concatenation of two StringOps class objects. The size of this new string should be appropriately set. Overload operator[] to return the character present at the specific index of the string variable str. The index specified begins from 1 and can range upto size. Index can also receive a negative value in which case -1 means the last character of the string variable str. The range in the negative side would be from -1 to -size. For example, if str = "hello"; then index 1 would correspond to character 'h'; index = -1 would correspond to character 'o', index = -2 for character '1' and so on. Zero index would stand invalid. • int findMatch (char set[0): This function searches the characters from the array set in the string str and returns the sum of frequency of matched characters in str. For example, if str "C++ programming" and set ('p', 'm', '+', 'h'}, then the value returned is 1+2+2 5. • void display () : This function should display the string object str and its size.
Write a program in C++ that defines a class named StringOps. Declare data members of this class as described below: • variable str of data type string. • variable size of data type integer which represents the length of the string str. Define member functions in StringOps as given below: parameterless and parameterized constructors that initialize the string variable str. void input () : This function accepts a string as input from the user and stores it in str. The length of this string is computed and stored in the variable size. • Overload operator + to create a new string obtained by concatenation of two StringOps class objects. The size of this new string should be appropriately set. Overload operator[] to return the character present at the specific index of the string variable str. The index specified begins from 1 and can range upto size. Index can also receive a negative value in which case -1 means the last character of the string variable str. The range in the negative side would be from -1 to -size. For example, if str = "hello"; then index 1 would correspond to character 'h'; index = -1 would correspond to character 'o', index = -2 for character '1' and so on. Zero index would stand invalid. • int findMatch (char set[0): This function searches the characters from the array set in the string str and returns the sum of frequency of matched characters in str. For example, if str "C++ programming" and set ('p', 'm', '+', 'h'}, then the value returned is 1+2+2 5. • void display () : This function should display the string object str and its size.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section12.2: Providing Class Conversion Capabilities
Problem 6E
Related questions
Question
![Write a program in C++ that defines a class named StringOps. Declare data members of
this class as described below:
variable str of data type string.
• variable size of data type integer which represents the length of the string str.
Define member functions in StringOps as given below:
parameterless and parameterized constructors that initialize the string variable str.
• void input () : This function accepts a string as input from the user and stores it in
str. The length of this string is computed and stored in the variable size.
• Overload operator + to create a new string obtained by concatenation of two
StringOps class objects. The size of this new string should be appropriately set.
• Overload operator[] to return the character present at the specific index of the string
variable str. The index specified begins from 1 and can range upto size. Index can
also receive a negative value in which case -1 means the last character of the string
variable str. The range in the negative side would be from -1 to -size. For example,
if str = "hello"; then index = 1 would correspond to character 'h'; index -1
would correspond to character 'o', index = -2 for character '1' and so on. Zero index
would stand invalid.
int findMatch (char set[]): This function searches the characters from the
array set in the string str and returns the sum of frequency of matched characters in
str. For example, if str = "C++ programming" and set = ('p', 'm',
'+', 'h'}, then the value returned is 1+2+2 = 5.
void display (): This function should display the string object str and its size.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faf323155-9f68-45f5-9cb5-b806bec08f6e%2F42080b2e-57d5-4356-9941-93c911a2f0a7%2F9kwz8on_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write a program in C++ that defines a class named StringOps. Declare data members of
this class as described below:
variable str of data type string.
• variable size of data type integer which represents the length of the string str.
Define member functions in StringOps as given below:
parameterless and parameterized constructors that initialize the string variable str.
• void input () : This function accepts a string as input from the user and stores it in
str. The length of this string is computed and stored in the variable size.
• Overload operator + to create a new string obtained by concatenation of two
StringOps class objects. The size of this new string should be appropriately set.
• Overload operator[] to return the character present at the specific index of the string
variable str. The index specified begins from 1 and can range upto size. Index can
also receive a negative value in which case -1 means the last character of the string
variable str. The range in the negative side would be from -1 to -size. For example,
if str = "hello"; then index = 1 would correspond to character 'h'; index -1
would correspond to character 'o', index = -2 for character '1' and so on. Zero index
would stand invalid.
int findMatch (char set[]): This function searches the characters from the
array set in the string str and returns the sum of frequency of matched characters in
str. For example, if str = "C++ programming" and set = ('p', 'm',
'+', 'h'}, then the value returned is 1+2+2 = 5.
void display (): This function should display the string object str and its size.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
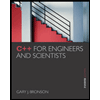
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
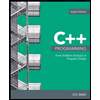
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
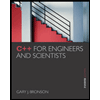
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
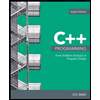
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning