Write code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods
Write code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods:
1. set: This method receives the array of person class and set the attribute.
2. displayAll: This method will display values of all persons.
3. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort.
4. reverse: This method will reverse the order of the array.
5. getPersons: This method will return the array of the persons (attribute).
6. displayEvenAgePerson: This method will display all those persons values who have even age.
7. displayOddAgePerson: This method will display all those persons values who have odd age.
8. displayPrimeAgePerson: This method will display only those persons values who have prime number age.
Following is the class of Person:
public class Person {
private String Name;
public int age;
public void set(String Name, int age)
{
this.Name = Name;
if(age > 0 && age <= 100)
this.age = age;
else
this.age = 1;
}
public void display()
{
System.out.println("Name: " + this.Name +" ,Age: " + this.age);
}
public static void main(String[] args)
{
PersonManagement personManagement = new PersonManagement();
Person p1 = new Person(), p2 = new Person(), p3 = new Person(), p4 = new Person(), p5 = new Person();
p1.set("Asad", 103);
p2.set("Ali", 17);
p3.set("Usman", 16);
p4.set("Raees", 18);
p5.set("Mujeeb", 15);
Person[] persons = new Person[]{p1,p2,p3,p4,p5};
System.out.println("Persons in Array");
personManagement.set(persons);
personManagement.displayAll();
System.out.println("Persons after Selection Sort");
personManagement.sort();
personManagement.displayAll();
System.out.println("Persons after Reverse of Array");
personManagement.reverse();
personManagement.displayAll();
System.out.println("Persons with Even Ages");
personManagement.displayEvenAgePerson();
System.out.println("Persons with Odd Ages");
personManagement.displayOddAgePerson();
System.out.println("Persons with Prime Ages");
personManagement.displayPrimeAgePerson();
}
}
Output of the Main method is:
Persons in Array
Name: Asad ,Age: 1
Name: Ali ,Age: 17
Name: Usman ,Age: 16
Name: Raees ,Age: 18
Name: Mujeeb ,Age: 15
Persons after Selection Sort
Name: Asad ,Age: 1
Name: Mujeeb ,Age: 15
Name: Usman ,Age: 16
Name: Ali ,Age: 17
Name: Raees ,Age: 18
Persons after Reverse of Array
Name: Raees ,Age: 18
Name: Ali ,Age: 17
Name: Usman ,Age: 16
Name: Mujeeb ,Age: 15
Name: Asad ,Age: 1
Persons with Even Ages
Name: Raees ,Age: 18
Name: Usman ,Age: 16
Persons with Odd Ages
Name: Ali ,Age: 17
Name: Mujeeb ,Age: 15
Name: Asad ,Age: 1
Persons with Prime Ages
Name: Ali ,Age: 17 Answer:

Step by step
Solved in 3 steps with 8 images

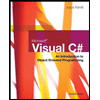
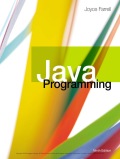
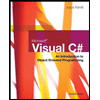
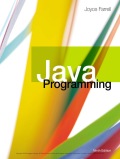