Write the definitions of the following functions: setWaitingTime getArrivalTime getTransactionTime getCustomerNumber of the class customerType defined in the section Application of Queues: Simulation #ifndef H_SIMULATION #define H_SIMULATION #include #include #include "queueAsArray.h" using namespace std; //**************** customerType **************** class customerType { public: customerType(int cN = 0, int arrvTime = 0, int wTime = 0, int tTime = 0); //Constructor to initialize the instance variables //according to the parameters //If no value is specified in the object declaration, //the default values are assigned. //Postcondition: customerNumber = cN; // arrivalTime = arrvTime; // waitingTime = wTime; // transactionTime = tTime void setCustomerInfo(int customerN = 0, int inTime = 0, int wTime = 0, int tTime = 0); //Function to initialize the instance variables. //Instance variables are set according to the parameters. //Postcondition: customerNumber = customerN; // arrivalTime = arrvTime; // waitingTime = wTime; // transactionTime = tTime; int getWaitingTime() const; //Function to return the waiting time of a customer. //Postcondition: The value of waitingTime is returned. void setWaitingTime(int time); //Function to set the waiting time of a customer. //Postcondition: waitingTime = time; void incrementWaitingTime(); //Function to increment the waiting time by one time unit. //Postcondition: waitingTime++; int getArrivalTime() const; //Function to return the arrival time of a customer. //Postcondition: The value of arrivalTime is returned. int getTransactionTime() const; //Function to return the transaction time of a customer. //Postcondition: The value of transactionTime is returned. int getCustomerNumber() const; //Function to return the customer number. //Postcondition: The value of customerNumber is returned. private: int customerNumber; int arrivalTime; int waitingTime; int transactionTime; };
Write the definitions of the following functions: setWaitingTime getArrivalTime getTransactionTime getCustomerNumber of the class customerType defined in the section Application of Queues: Simulation #ifndef H_SIMULATION #define H_SIMULATION #include #include #include "queueAsArray.h" using namespace std; //**************** customerType **************** class customerType { public: customerType(int cN = 0, int arrvTime = 0, int wTime = 0, int tTime = 0); //Constructor to initialize the instance variables //according to the parameters //If no value is specified in the object declaration, //the default values are assigned. //Postcondition: customerNumber = cN; // arrivalTime = arrvTime; // waitingTime = wTime; // transactionTime = tTime void setCustomerInfo(int customerN = 0, int inTime = 0, int wTime = 0, int tTime = 0); //Function to initialize the instance variables. //Instance variables are set according to the parameters. //Postcondition: customerNumber = customerN; // arrivalTime = arrvTime; // waitingTime = wTime; // transactionTime = tTime; int getWaitingTime() const; //Function to return the waiting time of a customer. //Postcondition: The value of waitingTime is returned. void setWaitingTime(int time); //Function to set the waiting time of a customer. //Postcondition: waitingTime = time; void incrementWaitingTime(); //Function to increment the waiting time by one time unit. //Postcondition: waitingTime++; int getArrivalTime() const; //Function to return the arrival time of a customer. //Postcondition: The value of arrivalTime is returned. int getTransactionTime() const; //Function to return the transaction time of a customer. //Postcondition: The value of transactionTime is returned. int getCustomerNumber() const; //Function to return the customer number. //Postcondition: The value of customerNumber is returned. private: int customerNumber; int arrivalTime; int waitingTime; int transactionTime; };
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 18PE
Related questions
Question
Help Please:
Write the definitions of the following functions:
- setWaitingTime
- getArrivalTime
- getTransactionTime
- getCustomerNumber
of the class customerType defined in the section Application of Queues: Simulation
#ifndef H_SIMULATION
#define H_SIMULATION
#include <fstream>
#include <string>
#include "queueAsArray.h"
using namespace std;
//**************** customerType ****************
class customerType
{
public:
customerType(int cN = 0, int arrvTime = 0, int wTime = 0,
int tTime = 0);
//Constructor to initialize the instance variables
//according to the parameters
//If no value is specified in the object declaration,
//the default values are assigned.
//Postcondition: customerNumber = cN;
// arrivalTime = arrvTime;
// waitingTime = wTime;
// transactionTime = tTime
void setCustomerInfo(int customerN = 0, int inTime = 0,
int wTime = 0, int tTime = 0);
//Function to initialize the instance variables.
//Instance variables are set according to the parameters.
//Postcondition: customerNumber = customerN;
// arrivalTime = arrvTime;
// waitingTime = wTime;
// transactionTime = tTime;
int getWaitingTime() const;
//Function to return the waiting time of a customer.
//Postcondition: The value of waitingTime is returned.
void setWaitingTime(int time);
//Function to set the waiting time of a customer.
//Postcondition: waitingTime = time;
void incrementWaitingTime();
//Function to increment the waiting time by one time unit.
//Postcondition: waitingTime++;
int getArrivalTime() const;
//Function to return the arrival time of a customer.
//Postcondition: The value of arrivalTime is returned.
int getTransactionTime() const;
//Function to return the transaction time of a customer.
//Postcondition: The value of transactionTime is returned.
int getCustomerNumber() const;
//Function to return the customer number.
//Postcondition: The value of customerNumber is returned.
private:
int customerNumber;
int arrivalTime;
int waitingTime;
int transactionTime;
};
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
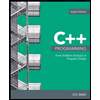
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
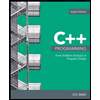
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning